User:NNikkhoui (WMF)/Sandbox
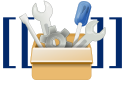
Hooks allow custom code to be executed when some defined event (such as saving a page or a user logging in) occurs.
For example, the following code snippet will trigger a call to the function MyExtensionHooks::pageContentSaveComplete
whenever the PageContentSaveComplete
hook runs, passing it function arguments specific to PageContentSaveComplete .
Hooks can be registered by mapping the name of the hook to the callback in the extension's extension.json file:
"Hooks": { "PageContentSaveComplete": "MyExtensionHooks::onPageContentSaveComplete" }
MediaWiki provides many hooks like this to extend the functionality of the MediaWiki software. Assigning a function (known as an event handler) to a hook will cause that function to be called at the appropriate point in the main MediaWiki code, to perform whatever additional task(s) the developer thinks would be useful at that point. Each hook can have multiple handlers assigned to it, in which case it will call the functions in the order that they are assigned, with any modifications made by one function passed on to subsequent functions in the chain.
Assign functions to hooks at the end of LocalSettings.php or in your own extension file at the file scope (not in a $wgExtensionFunctions
function or the ParserFirstCallInit hook). For extensions, if the hook function's behavior is conditioned on a setting in LocalSettings.php, the hook should be assigned and the function should terminate early if the condition was not met.
You can also create new hooks in your own extension; if you do so, add them to the Extension hook registry.
Background
[edit]A hook is triggered by a call to the function Hooks::run (described in file hooks.txt, and defined in GlobalFunctions.php. The first argument to Hooks::run is the name of the hook, the second is the array of arguments for that hook. It will find the event handlers to run in the array $wgHooks
. It calls the PHP function call_user_func_array with arguments being the function to be called and its arguments.
See also the hook specification in core.
In this example from the doPurge
function in WikiPage.php, doPurge calls Hooks::run to run the ArticlePurge hook, passing &$wikiPage
as argument:
Hooks::run( 'ArticlePurge', [ &$wikiPage ] );
The Core calls many hooks, but Extensions can also call hooks.
Writing an event handler
[edit]An event handler is a function you assign to a hook, which will be run whenever the event represented by that hook occurs. It consists of:
- a function with some optional accompanying data, or
- an object with a method and some optional accompanying data.
Register the event handler by adding it to the global $wgHooks
array for a given event.
Hooks can be added from any point in the execution before the hook is called, but are most commonly added in LocalSettings.php, its included files, or, for extensions, in the file extension.json.
All the following are valid ways to define a hook function for the event EventName that is passed two parameters, showing the code that will be executed when EventName happens:
Format | Syntax | Resulting function call. |
---|---|---|
Static function | $wgHooks['EventName'][] = 'MyExtensionHooks::onEventName';
|
MyExtensionHooks::onEventName( $param1, $param2 );
|
Function, no data | $wgHooks['EventName'][] = 'someFunction';
|
someFunction( $param1, $param2 );
|
Function with data | $wgHooks['EventName'][] = [ 'someFunction', $someData ];
|
someFunction( $someData, $param1, $param2 );
|
Function, no data (weird syntax, but OK) |
$wgHooks['EventName'][] = [ 'someFunction' ];
|
someFunction( $param1, $param2 );
|
inline anonymous function |
$wgHooks['EventName'][] = function ( $param1, $param2 ) {
// ...function body
}
|
(the anonymous function is called with the hook's parameters) |
Object only | $wgHooks['EventName'][] = $object;
|
$object->onEventName( $param1, $param2 );
|
Object with method | $wgHooks['EventName'][] = [ $object, 'someMethod' ];
|
$object->someMethod( $param1, $param2 );
|
Object with method and data | $wgHooks['EventName'][] = [ $object, 'someMethod', $someData ];
|
$object->someMethod( $someData, $param1, $param2 );
|
Object only (weird syntax, but OK) |
$wgHooks['EventName'][] = [ $object ];
|
$object->onEventName( $param1, $param2 );
|
For extensions, the syntax is similar in the file extension.json
(corresponding to the first and second case above):
{
"Hooks": {
"EventName": [
"MyExtensionHooks::onEventName",
"someFunction"
]
}
}
When an event occurs, the function (or object method) that you registered will be called, the event's parameters, along with any optional data you provided at registration. Note that when an object is the hook and you didn't specify a method, the method called is 'onEventName'. For other events this would be 'onArticleSave', 'onUserLogin', etc.
The optional data is useful if you want to use the same function or object for different purposes. For example:
$wgHooks['PageContentSaveComplete'][] = [ 'ircNotify', 'TimStarling' ];
$wgHooks['PageContentSaveComplete'][] = [ 'ircNotify', 'brion' ];
This code would result in ircNotify being run twice when a page is saved: once for 'TimStarling', and once for 'brion'.
Event handlers can return one of three possible values:
- no return value (or null): the hook handler has operated successfully. (Before MediaWiki 1.23, returning true was required.)
- "some string": an error occurred; processing should stop and the error should be shown to the user
- false: the hook handler has done all the work necessary, or replaced normal handling. This will prevent further handlers from being run, and in some cases tells the calling function to skip normal processing.
Returning false makes less sense for events where the action is complete, and will normally be ignored by the caller.
Hook behavior in MediaWiki 1.35 and after
[edit]MediaWiki 1.35 introduced a new system for creating and using hooks. Previous to 1.35, hook handlers had no way to inject dependencies. This problem was solved by defining hook handlers as ObjectFactory objects. Additionally, using hook interfaces allows validation of hook arguments as well as serving as documentation for the hook itself. Finally, the hook runner is instantiated as a service instead of a static function allowing for dependency injection and ease of testability.
Registration
[edit]Create handler
namespace MediaWiki\Extension\MyExtension;
use OutputPage;
use Skin;
class HookHandler implements BeforePageDisplayHook {
public function onBeforePageDisplayHook( ( OutputPage $out, Skin $skin ) { ... }
}
Attach hook in extension.json
{
"Hooks": {
"MyHook": {
"handler": "main"
}
},
"HookHandlers": {
"main": {
"class": "MediaWiki\\Extension\\MyExtension\\HookHandler",
"services": [ "UserNameUtils" ]
}
}
}
Deprecation
[edit]- ( handling deprecation warnings and acknowledging deprecation) To acknowledge deprecation, include the deprecated property.
- Will log a deprecation warning if: - 1. the hook is marked deprecated - 2. an extension registers a handler in the new way but does not acknowledge deprecation
- Silent deprecation has not been merged yet (https://gerrit.wikimedia.org/r/c/mediawiki/core/+/601950)
{
"Hooks": {
"MyHook": {
"handler": "main",
"deprecated": true
}
}
}
Hook behavior before MediaWiki 1.22 vs after
[edit]Extracted from: change 500542: for non-abortable hooks (most hooks) returning true has been redundant since MediaWiki 1.22 (in 2015). This was done to reduce chances of accidental failure because we had experienced several outages and broken features due to silent failures where e.g. one hook callback somewhere accidentally returned a non-bool or false instead of true/void and thus short-circuits the whole system.
(Returning non-true/non-void in a MediaWiki Hook is equivalent to e.preventDefault
and e.stopImmediatePropagation
in JavaScript events, it kills other listeners for the same event).
For example, if onBeforePageDisplay
hook were to return false in MobileFrontend, it would mean Popups stops because its callback would no longer run.
See differences below, assuming the hook onBeforePageDisplay
.
Before MediaWiki 1.22
public static function onBeforePageDisplay( OutputPage $out, Skin $skin ) {
// some code
return true; // explicit
}
or
public static function onBeforePageDisplay( OutputPage $out, Skin $skin ) {
// some code
return; // explicit
}
MediaWiki 1.22+
public static function onBeforePageDisplay( OutputPage $out, Skin $skin ) {
// some code
// no need for a return true or return
}
Documentation
[edit]Currently, hooks in MediaWiki core have to be documented both in docs/hooks.txt (in the source code repository) and here on MediaWiki.org. In some cases, one of these steps may not yet have been completed, so if a hook appears undocumented, check both.
To document a hook on-wiki, use {{MediaWikiHook }}.
Available hooks
[edit]Hooks grouped by function
[edit]Some of these hooks can be grouped into multiple functions.
- Sections: Article Management - Edit Page - Page Rendering - User Interface - Special Pages - User Management - Logging - Skinning Templates - API - Import/Export - Miscellaneous
Function | Version | Hook | Description |
---|---|---|---|
Article Management | 1.4.0 | ArticleDelete | Occurs whenever the software receives a request to delete an article |
1.4.0 | ArticleDeleteComplete | Occurs after the delete article request has been processed | |
1.9.1 | ArticleUndelete | When one or more revisions of an article are restored | |
1.12.0 | ArticleRevisionUndeleted | Occurs after an article revision is restored | |
1.8.0 | ArticleFromTitle | Called to determine the class to handle the article rendering, based on title | |
1.4.0 | ArticleProtect | Occurs whenever the software receives a request to protect an article | |
1.4.0 | ArticleProtectComplete | Occurs after the protect article request has been processed | |
1.6.0 | ArticleInsertComplete | Occurs after a new article has been created | |
1.11.0 | RevisionInsertComplete | Called after a revision is inserted into the DB | |
1.4.0 | ArticleSave | (deprecated) (use MultiContentSave) Occurs whenever the software receives a request to save an article | |
1.21.0 | PageContentSave | (deprecated) (use MultiContentSave) Occurs whenever the software receives a request to save an article | |
1.35.0 | MultiContentSave | Occurs whenever the software receives a request to save an article | |
1.4.0 | ArticleSaveComplete | (deprecated) (use PageContentSaveComplete) Occurs after the save article request has been processed | |
1.21.0 | ConvertContent | Called when a conversion to another content model is requested. | |
1.21.0 | PageContentSaveComplete | Occurs after the save article request has been processed | |
1.11.0 | ArticleUpdateBeforeRedirect | Occurs after a page is updated (usually on save), before the user is redirected back to the page | |
1.5.7 | ArticleEditUpdateNewTalk | Allows an extension to prevent user notification when a new message is added to their talk page. | |
1.14.0 | ArticleEditUpdates | Called when edit updates (mainly link tracking) are made when an article has been changed. | |
1.6.0 | ArticleEditUpdatesDeleteFromRecentchanges | Occurs before saving to the database. If returning false old entries are not deleted from the recentchangeslist. | |
1.8.0 | RecentChange_save | Called after a "Recent Change" is committed to the DB | |
1.6.0 | SpecialMovepageAfterMove | Called after a page is moved. | |
1.22.0 | TitleMove | Occurs before a requested pagemove is performed | |
1.4.0 | TitleMoveComplete | Occurs whenever a request to move an article is completed | |
1.25.0 | MovePageIsValidMove | Specify whether a page can be moved for technical reasons. | |
1.12.0 | ArticleRollbackComplete | Occurs after an article rollback is completed | |
1.12.0 | ArticleMergeComplete | after merging to article using Special:Mergehistory | |
1.6.0 | ArticlePurge | Allows an extension to cancel a purge. | |
1.13.0 | ArticleRevisionVisibilitySet | called when changing visibility of one or more revisions of an article | |
1.21.0 | ContentHandlerDefaultModelFor | called when deciding the default content model for a given title. | |
1.21.0 | ContentHandlerForModelID | called when a ContentHandler is requested for a given content model name, but no entry for that model exists in $wgContentHandlers . | |
1.23.0 | Article::MissingArticleConditions | called when showing a page. | |
1.20.0 | TitleIsAlwaysKnown | Allows overriding default behaviour for determining if a page exists. | |
Edit Page | 1.6.0 | AlternateEdit | Used to replace the entire edit page, altogether. |
1.21.0 | AlternateEditPreview | Allows replacement of the edit preview | |
1.6.0 | EditFilter | Perform checks on an edit | |
1.12.0 | EditFilterMerged | (deprecated) (use EditFilterMergedContent) Post-section-merge edit filter | |
1.21.0 | EditFilterMergedContent | Post-section-merge edit filter | |
1.7.0 | EditFormPreloadText | Called when edit page for a new article is shown. This lets you fill the text-box of a new page with initial wikitext. | |
1.8.3 | EditPage::attemptSave | Called before an article is saved, that is before insertNewArticle() is called | |
1.6.0 | EditPage::showEditForm:fields | Allows injection of form field into edit form. | |
1.6.0 | EditPage::showEditForm:initial | Allows injection of HTML into edit form | |
1.21.0 | EditPage::showStandardInputs:options | Allows injection of form fields into the editOptions area | |
1.13.0 | EditPageBeforeConflictDiff | Allows modifying the EditPage object and output when there's an edit conflict. | |
1.12.0 | EditPageBeforeEditButtons | Used to modify the edit buttons on the edit form | |
1.14.0 | EditPageBeforeEditChecks | Allows modifying the edit checks below the textarea in the edit form | |
Page Rendering | 1.27.0 | AfterBuildFeedLinks | Executed after all feed links are created. |
1.6.0 | ArticlePageDataBefore | Executes before data is loaded for the article requested. | |
1.6.0 | ArticlePageDataAfter | Executes after loading the data of an article from the database. | |
1.6.0 | ArticleViewHeader | Called after an articleheader is shown. | |
1.6.0 | PageRenderingHash | Alter the parser cache option hash key. | |
1.6.0 | ArticleAfterFetchContent | Used to process raw wiki code after most of the other parser processing is complete. | |
1.22.0 | GalleryGetModes | Allows extensions to add classes that can render different modes of a gallery. | |
1.6.0 | ParserClearState | Called at the end of Parser::clearState() | |
1.21.0 | ParserCloned | Called when the Parser object is cloned. | |
1.5.0 | ParserBeforeStrip | Used to process the raw wiki code before any internal processing is applied. | |
1.5.0 | ParserAfterStrip | (deprecated) Before version 1.14.0, used to process raw wiki code after text surrounded by <nowiki> tags have been protected but before any other wiki text has been processed. In version 1.14.0 and later, runs immediately after ParserBeforeStrip.
| |
1.6.0 | ParserBeforeInternalParse | Replaces the normal processing of stripped wiki text with custom processing. Used primarily to support alternatives (rather than additions) to the core MediaWiki markup syntax. | |
1.6.0 | ParserGetVariableValueVarCache | Use this to change the value of the variable cache or return false to not use it. | |
1.6.0 | ParserGetVariableValueTs | Use this to change the value of the time for the {{LOCAL...}} magic word. | |
1.6.0 | ParserGetVariableValueSwitch | Assigns a value to a user defined variable. | |
1.10.0 | InternalParseBeforeLinks | Used to process the expanded wiki code after <nowiki>, HTML-comments, and templates have been treated. Suitable for syntax extensions that want to customize the treatment of internal link syntax, i.e. [[....]] .
| |
1.13.0 | LinkerMakeExternalLink | Called before the HTML for external links is returned. Used for modifying external link HTML. | |
1.13.0 | LinkerMakeExternalImage | Called before external image HTML is returned. Used for modifying external image HTML. | |
1.23.0 | LinkerMakeMediaLinkFile | Called before the HTML for media links is returned. Used for modifying media link HTML. | |
1.11.0 | EditSectionLink | (deprecated) Override the return value of Linker::editSectionLink(). Called after creating [edit] link in header in Linker::editSectionLink but before HTML is displayed. | |
1.11.0 | EditSectionLinkForOther | (deprecated) Override the return value of Linker::editSectionLinkForOther(). Called after creating [edit] link in header in Linker::editSectionLinkForOther but before HTML is displayed. | |
1.5.0 | ParserBeforeTidy | Used to process the nearly-rendered html code for the page (but before any html tidying occurs). | |
1.5.0 | ParserAfterTidy | Used to add some final processing to the fully-rendered page output. | |
1.24.0 | ContentGetParserOutput | Customize parser output for a given content object, called by AbstractContent::getParserOutput. May be used to override the normal model-specific rendering of page content. | |
1.8.0 | OutputPageParserOutput | Called after parse, before the HTML is added to the output. | |
1.6.0 | OutputPageBeforeHTML | Called every time wikitext is added to the OutputPage, after it is parsed but before it is added. Called after the page has been rendered, but before the HTML is displayed. | |
1.4.3 | CategoryPageView | Called before viewing a categorypage in CategoryPage::view | |
1.5.1 | ArticleViewRedirect | Allows an extension to prevent the display of a "Redirected From" link on a redirect page. | |
1.10.0 | IsFileCacheable | Allow an extension to disable file caching on pages. | |
1.10.1 | BeforeParserFetchTemplateAndtitle | Allows an extension to specify a version of a page to get for inclusion in a template. | |
1.18.0 | BeforeParserFetchFileAndTitle | Allows an extension to select a different version of an image to link to. | |
1.10.1 | BeforeParserrenderImageGallery | Allows an extension to modify an image gallery before it is rendered. | |
1.17.0 | ResourceLoaderRegisterModules | Allows registering modules with ResourceLoader | |
1.24.0 | SidebarBeforeOutput | Directly before the sidebar is output | |
User Interface | 1.5.4 | AutoAuthenticate | Called to authenticate users on external/environmental means |
1.4.0 | UserLoginComplete | Occurs after a user has successfully logged in | |
1.18.0 | BeforeWelcomeCreation | Allows an extension to change the message displayed upon a successful login. | |
1.4.0 | UserLogout | Occurs when the software receives a request to log out | |
1.4.0 | UserLogoutComplete | Occurs after a user has successfully logged out | |
1.6.0 | userCan | To interrupt/advise the "user can do X to Y article" check | |
1.4.0 | WatchArticle | Occurs whenever the software receives a request to watch an article | |
1.4.0 | WatchArticleComplete | Occurs after the watch article request has been processed | |
1.4.0 | UnwatchArticle | Occurs whenever the software receives a request to unwatch an article | |
1.4.0 | UnwatchArticleComplete | Occurs after the unwatch article request has been processed | |
1.6.0 | MarkPatrolled | Called before an edit is marked patrolled | |
1.6.0 | MarkPatrolledComplete | Called after an edit is marked patrolled | |
1.4.0 | EmailUser | Occurs whenever the software receives a request to send an email from one user to another | |
1.4.0 | EmailUserComplete | Occurs after an email has been sent from one user to another | |
1.6.0 | SpecialMovepageAfterMove | Called after a page is moved. | |
1.19.0 | SpecialSearchCreateLink | Called when making the message to create a page or go to an existing page | |
1.17.0 | SpecialSearchGo | (deprecated) | |
1.6.0 | SpecialSearchNogomatch | Called when the 'Go' feature is triggered and the target doesn't exist. Full text search results are generated after this hook is called | |
1.19.0 | SpecialSearchPowerBox | the equivalent of SpecialSearchProfileForm for the advanced form | |
1.5.7 | ArticleEditUpdateNewTalk | Before updating user_newtalk when a user talk page was changed. | |
1.5.7 | UserRetrieveNewTalks | called when retrieving "You have new messages!" message(s) | |
1.5.7 | UserClearNewTalkNotification | called when clearing the "You have new messages!" message, return false to not delete it | |
1.6.0 | ArticlePurge | Executes before running "&action=purge" | |
File Upload | 1.6.0 | UploadVerification | (deprecated) (use UploadVerifyFile) Called when a file is uploaded, to allow extra file verification to take place |
1.17 | UploadVerifyFile | Called when a file is uploaded, to allow extra file verification to take place | |
1.28 | UploadVerifyUpload | (preferred) Can be used to reject a file upload. Unlike 'UploadVerifyFile' it provides information about upload comment and the file description page, but does not run for uploads to stash. | |
1.6.4 | UploadComplete | Called when a file upload has completed. | |
Special pages | 1.6.0 | SpecialPageGetRedirect | |
1.24.0 | SpecialBlockModifyFormFields | Add or modify block fields of Special:Block | |
1.28.0 | SpecialContributions::formatRow::flags | Called before rendering a Special:Contributions row. | |
1.13.0 | SpecialListusersDefaultQuery | Called right before the end of UsersPager::getDefaultQuery() | |
1.13.0 | SpecialListusersFormatRow | Called right before the end of UsersPager::formatRow() | |
1.13.0 | SpecialListusersHeader | Called before closing the <fieldset> in UsersPager::getPageHeader()
| |
1.13.0 | SpecialListusersHeaderForm | Called before adding the submit button in UsersPager::getPageHeader() | |
1.13.0 | SpecialListusersQueryInfo | Called right before the end of UsersPager::getQueryInfo() | |
1.6.0 | SpecialPageExecuteBeforeHeader | (deprecated) called before setting the header text of the special page | |
1.6.0 | SpecialPageExecuteBeforePage | (deprecated) Called before setting the page text of the special page | |
1.6.0 | SpecialPageExecuteAfterPage | (deprecated) called after executing a special page | |
1.6.0 | SpecialVersionExtensionTypes | (deprecated) Adds to the types that can be used with $wgExtensionCredits | |
1.7.0 | SpecialPage_initList | Called after the Special Page list is populated | |
1.9.0 | UploadForm:initial | Called just before the upload form is generated | |
1.9.0 | UploadForm:BeforeProcessing | Called just before the file data (for example description) are processed, so extensions have a chance to manipulate them. | |
1.14.0 | UserrightsChangeableGroups | Called after the list of groups that can be manipulated via Special:UserRights is populated, but before it is returned. | |
1.24.0 | WhatLinksHereProps | Allows extensions to annotate WhatLinksHere entries. | |
User Management | 1.5.0 | AddNewAccount | Called after a user account is created (deprecated) |
1.26.0 | LocalUserCreated | Called immediately after a local user has been created and saved to the database. | |
1.27.0 | SessionMetadata | Add metadata to a session being saved | |
1.27.0 | SessionCheckInfo | Validate session info as it's being loaded from storage | |
1.27.0 | SecuritySensitiveOperationStatus | Affect the return value from AuthManager::securitySensitiveOperationStatus() | |
1.27.0 | UserLoggedIn | Called after a user is logged in | |
1.4.0 | BlockIp | Occurs whenever the software receives a request to block (or change the block settings of) an IP address or user | |
1.4.0 | BlockIpComplete | Occurs after the request to block (or change block settings of) an IP or user has been processed | |
1.29.0 | UnblockUser | Occurs whenever the software receives a request to unblock an IP address or user | |
1.29.0 | UnblockUserComplete | Occurs after the request to unblock an IP or user has been processed | |
1.29.0 | ChangeUserGroups | Called before a user's group memberships are changed | |
1.6.0 | UserRights | (deprecated) (use UserGroupsChanged) Called after a user's group memberships are changed | |
1.11.0 | UserGetRights | Called in User::getRights() to dynamically add rights | |
1.32.0 | UserGetRightsRemove | Called in User::getRights() to dynamically remove rights | |
1.6.0 | GetBlockedStatus | Fired after the user's getBlockStatus is set | |
1.26.0 | UserIsLocked | Fired to check if a user account is locked | |
Logging | 1.6.0 | LogPageActionText | (deprecated) Strings used by wfMsg as a header. |
1.5.0 | LogPageLogHeader | (deprecated) | |
1.5.0 | LogPageLogName | (deprecated) | |
1.5.0 | LogPageValidTypes | (deprecated) | |
1.26.0 | LogException | Called before an exception (or PHP error) is logged. | |
Skinning / Templates | 1.7.0 | BeforePageDisplay | Allows last minute changes to the output page, e.g. adding of CSS or Javascript by extensions. |
1.6.0 | MonoBookTemplateToolboxEnd | Called by Monobook skin after toolbox links have been rendered (useful for adding more) | |
1.7.0 | PersonalUrls | (SkinTemplate.php) Called after the list of personal URLs (links at the top in Monobook) has been populated. | |
1.24.0 | PostLoginRedirect | (SpecialUserlogin.php) Modify the post login redirect behavior. | |
1.23.0 | BaseTemplateAfterPortlet | (SkinTemplate.php) After rendering of portlets. | |
1.11.0 | SkinAfterBottomScripts | (Skin.php) At the end of Skin::bottomScripts() | |
1.12.0 | SkinSubPageSubtitle | (Skin.php) Called before the list of subpage links on top of a subpage is generated | |
1.5.0 | SkinTemplateContentActions | Called after the default tab list is populated (list is context dependent i.e. "normal" article or "special page"). | |
1.16.0 | SkinTemplateNavigation | Called on content pages only after tabs have been added, but before variants have been added. See the other two SkinTemplateNavigation hooks for other points tabs can be modified at. | |
1.18.0 | SkinTemplateNavigation::Universal | Called on both content and special pages after variants have been added | |
1.18.0 | SkinTemplateNavigation::SpecialPage | Called on special pages after the special tab is added but before variants have been added | |
1.6.0 | SkinTemplatePreventOtherActiveTabs | Called to enable/disable the inclusion of additional tabs to the skin. | |
1.6.0 | SkinTemplateSetupPageCss | (deprecated) Sets up the CSS for a skin | |
1.6.0 | SkinTemplateBuildContentActionUrlsAfterSpecialPage | (deprecated) After the single tab when showing a special page | |
1.6.0 | SkinTemplateBuildNavUrlsNav_urlsAfterPermalink | Called after the permalink has been entered in navigation URL array. | |
1.23.0 | SkinTemplateGetLanguageLink | Called after building the data for a language link from which the actual html is constructed. | |
1.27.0 | AuthChangeFormFields | Allows modification of AuthManager-based forms | |
1.25.0 | LoginFormValidErrorMessages | Allows to add additional error messages (SpecialUserLogin.php). | |
1.25.0 | MinervaDiscoveryTools | Allow other extensions to add or override discovery tools (SkinMinerva.php). | |
API | 1.23.0 | ApiBeforeMain | Called before ApiMain is executed |
1.13.0 | APIEditBeforeSave | Called right before saving an edit submitted through api.php?action=edit | |
1.13.0 | APIQueryInfoTokens | Use this hook to add custom tokens to prop=info | |
1.13.0 | APIQueryRevisionsTokens | Use this hook to add custom tokens to prop=revisions | |
1.14.0 | APIQueryRecentChangesTokens | Use this hook to add custom tokens to list=recentchanges | |
1.14.0 | APIGetAllowedParams | Use this hook to modify a module's parameters | |
1.14.0 | APIGetParamDescription | Use this hook to modify a module's parameter descriptions | |
1.14.0 | APIAfterExecute | Use this hook to extend core API modules | |
1.14.0 | APIQueryAfterExecute | Use this hook to extend core API query modules | |
1.14.0 | APIQueryGeneratorAfterExecute | Use this hook to extend core API query modules | |
1.23.0 | GetExtendedMetadata | Allows including additional file metadata information in the imageinfo API. | |
1.23.0 | AddNewAccountApiForm | Allows modifying the internal login form when creating an account via the API. | |
1.23.0 | AddNewAccountApiResult | Modifies the API output when an account is created via the API. | |
1.25.0 | ApiOpenSearchSuggest | Called when constructing the OpenSearch results. Hooks can alter or append to the array. | |
Import/Export | 1.17.0 | AfterImportPage | When a page import is completed |
1.17.0 | ImportHandleLogItemXMLTag | When parsing a XML tag in a log item | |
1.17.0 | ImportHandlePageXMLTag | When parsing a XML tag in a page | |
1.17.0 | ImportHandleRevisionXMLTag | When parsing a XML tag in a page revision | |
1.17.0 | ImportHandleToplevelXMLTag | When parsing a top level XML tag | |
1.17.0 | ImportHandleUploadXMLTag | When parsing a XML tag in a file upload | |
1.16.0 | ModifyExportQuery | Modify the query used by the exporter. | |
1.15.0 | WikiExporter::dumpStableQuery | Get the SELECT query for "stable" revisions dumps | |
1.16.0 | XmlDumpWriterOpenPage | Called at the end of XmlDumpWriter::openPage, to allow extra metadata to be added. | |
1.16.0 | XmlDumpWriterWriteRevision | Called at the end of a revision in an XML dump, to add extra metadata. | |
Miscellaneous | 1.19.0 | AlternateUserMailer | Called before mail is sent so that mail could be logged (or something else) instead of using PEAR or PHP's mail(). |
1.6.0 | ArticleEditUpdatesDeleteFromRecentchanges | Occurs before saving to the database. | |
1.21.0 | CategoryAfterPageAdded | Called after a page is added to a category | |
1.21.0 | CategoryAfterPageRemoved | Called after a page is removed from a category | |
1.19.0 | Collation::factory | Allows extensions to register new collation names, to be used with $wgCategoryCollation | |
1.8.0 | DisplayOldSubtitle | Allows extensions to modify the displaying of links to other revisions when browsing through revisions. | |
1.18.0 | GetDefaultSortkey | Allows to override what the default sortkey is, which is used to order pages in a category. | |
1.25.0 | GetDifferenceEngine | Allows custom difference engine extensions such as Special:MyLanguage/Extension:WikEdDiff. | |
1.21.0 | GetDoubleUnderscoreIDs | hook for modifying the list of magic words | |
1.6.0 | GetInternalURL | Used to modify fully-qualified URLs (useful for squid cache purging) | |
1.6.0 | GetLocalURL | Used to modify local URLs as output into page links | |
1.6.0 | GetFullURL | Used to modify fully-qualified URLs used in redirects/export/offsite data | |
1.16.0 | ImgAuthBeforeStream | Executed before file is streamed to user, but only when using img_auth | |
1.22.0 | LanguageLinks | Manipulate a page's language links. | |
1.14.0 | LinkBegin | Used when generating internal and interwiki links in Linker::link() | |
1.14.0 | LinkEnd | Used when generating internal and interwiki links in Linker::link(), just before the function returns a value. | |
1.8.0 | LoadAllMessages | (deprecated) Called when $wgMessageCache->loadAllMessages() has been invoked, allows extensions to inject their own messages. | |
1.6.0 | MagicWordMagicWords | (deprecated) Allows extensions to define new magic words. | |
1.6.0 | MagicWordwgVariableIDs | Tells MediaWiki that one or more magic word IDs should be treated as variables. | |
1.12.0 | MediaWikiPerformAction | Override MediaWiki::performAction() | |
1.27.0 | MediaWikiServices | Called when a global MediaWikiServices instance is initialised. | |
1.5.7 | MessagesPreLoad | Occurs when loading a message from the database | |
1.6.0 | ParserTestParser | called when creating a new instance of Parser for parser tests | |
1.8.0 | RecentChange_save | Called after a "Recent Change" is committed to the DB | |
1.5.0 | SpecialContributionsBeforeMainOutput | Before the form on Special:Contributions | |
1.22.0 | TitleSquidURLs | To modify/provide alternate URLs to send HTTP PURGE requests. | |
1.17.0 | UnitTestsList | Add tests that should be run as part of the unit test suite. | |
1.30.0 | UnitTestsAfterDatabaseSetup | Called right after MediaWiki's test infrastructure has finished creating/duplicating core tables for unit tests. | |
1.30.0 | UnitTestsBeforeDatabaseTeardown | Called right before MediaWiki's test infrastructure begins tearing down tables for unit tests. | |
1.4.0 | UnknownAction | (deprecated) Before MediaWiki 1.19, used to add new query-string actions. | |
1.24.0 | UserMailerChangeReturnPath | Called to generate a VERP return address when UserMailer sends an email, with a bounce handling extension. | |
1.8.0 | UserToggles | (deprecated) Called before returning user preference names. Use the GetPreferences hook instead. | |
1.6.0 | wgQueryPages | Runs for every Special page that extends the QueryPage class (fired when including the file QueryPage.php). It is only useful in maintenance/updateSpecialPages.php and in QueryPage Api. |
Alphabetical list of hooks
[edit]For a complete list of hooks, use the category , which should be kept more up to date.
See also
[edit]$wgHooks
- Category:Hook extensions
- Manual:Tag extensions
- Manual:Parser functions
- hooks.txt — documentation of the hooks in MediaWiki core
- Extension:Example — contains examples of hooks
- mw.hook — the JavaScript/front-end system of hooks