Manual:Parser functions
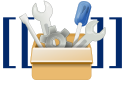
Parser functions, added in MediaWiki 1.7, are a type of extension that integrate closely with the parser. The phrase "parser function" should not be confused with Extension:ParserFunctions , which is a collection of simple parser functions. (See Help:Extension:ParserFunctions for those.)
Description
Whereas a tag extension is expected to take unprocessed text and return HTML to the browser, a parser function can 'interact' with other wiki elements in the page. For example, the output of a parser function could be used as a template parameter or in the construction of a link.
The typical syntax for a parser function is:
{{ #functionname: param1 | param2 | param3 }}
For more information, see the documentation for Parser::setFunctionHook ( $id, $callback, $flags = 0 )
. This documentation states:
- The callback function should have the form:
function myParserFunction( $parser, $arg1, $arg2, $arg3 ) { ... }
- Or with
SFH_OBJECT_ARGS
:function myParserFunction( $parser, $frame, $args ) { ... }
The first variant of the call passes all arguments as plain text.
The second passes all arguments as an array of PPNode s, except for the first ($args[0]
), which is currently text, though this may change in the future.
These represent the unexpanded wikitext.
The $frame
parameter can be used to expand these arguments as needed.
This is commonly used for conditional processing so that only the true
case is evaluated with an if-
or switch-like
parser function.
The frame object can also climb up the document tree to get information about the caller and has functions to determine and manage call depth, time-to-live, and whether the result of the parser function is volatile.
Creating a parser function is slightly more complicated than creating a new tag because the function name must be a magic word, a keyword that supports aliases and localization.
Simple example
Below is an example of an extension that creates a parser function.
The registration goes into extension.json and the code into src/ExampleExtensionHooks.php respectively:
{
"name": "ExampleExtension",
"author": "Me",
"version": "1.0.0",
"url": "https://www.mediawiki.org/wiki/Extension:ExampleExtension",
"descriptionmsg": "exampleextension-desc",
"license-name": "GPL-2.0-or-later",
"type": "parserhook",
"MessagesDirs": {
"ExampleExtension": [
"i18n"
]
},
"AutoloadClasses": {
"ExampleExtensionHooks": "src/ExampleExtensionHooks.php"
},
"ExtensionMessagesFiles": {
"ExampleExtensionMagic": "ExampleExtension.i18n.php"
},
"Hooks": {
"ParserFirstCallInit": "ExampleExtensionHooks::onParserFirstCallInit"
},
"manifest_version": 1
}
<?php
class ExampleExtensionHooks {
// Register any render callbacks with the parser
public static function onParserFirstCallInit( Parser $parser ) {
// Create a function hook associating the <code>example</code> magic word with renderExample()
$parser->setFunctionHook( 'example', [ self::class, 'renderExample' ] );
}
// Render the output of {{#example:}}.
public static function renderExample( Parser $parser, $param1 = '', $param2 = '', $param3 = '' ) {
// The input parameters are wikitext with templates expanded.
// The output should be wikitext too.
$output = "param1 is $param1 and param2 is $param2 and param3 is $param3";
return $output;
}
}
Another file, ExampleExtension.i18n.php, in your extension directory (Not in the src/ subdirectory) should contain:
<?php
/**
* @license GPL-2.0-or-later
* @author Your Name (YourUserName)
*/
$magicWords = [];
/** English
* @author Your Name (YourUserName)
*/
$magicWords['en'] = [
'example' => [ 0, 'example' ],
];
With this extension enabled,
- {{#example: hello | hi | hey}}
produces:
- param1 is hello and param2 is hi and param3 is hey
LocalSettings.php
MediaWiki\MediaWikiServices::getInstance()->getContentLanguage()->mMagicExtensions['wikicodeToHtml'] = ['MAG_CUSTOM', 'custom'];
Within LocalSettings.php
Magic words and their handling parser functions can be defined entirely in LocalSettings.php.
$wgHooks['ParserFirstCallInit'][] = function ( Parser $parser )
{
MediaWiki\MediaWikiServices::getInstance()->getContentLanguage()->mMagicExtensions['wikicodeToHtml'] = ['wikicodeToHtml', 'wikicodeToHtml'];
$parser->setFunctionHook( 'wikicodeToHtml', 'wikicodeToHtml' );
};
function wikicodeToHtml( Parser $parser, $code = '' )
{
$title = $parser->getTitle();
$options = $parser->Options();
$options->enableLimitReport(false);
$parser = $parser->getFreshParser();
return [$parser->parse($code, $title, $options)->getText(), 'isHTML' => true];
}
Longer functions
For longer functions, you may want to split the hook functions out to a _body.php or .hooks.php file and make them static functions of a class. Then you can load the class with $wgAutoloadClasses and call the static functions in the hooks; e.g.:
Put this in your extension.json
file:
"Hooks": {
"ParserFirstCallInit": "ExampleExtensionHooks::onParserFirstCallInit"
},
"AutoloadClasses": {
"ExampleExtensionHooks": "src/ExampleExtensionHooks.php"
}
- See: writing an event handler for other styles.
Then put this is in your src/ExampleExtensionHooks.php
file:
class ExampleExtensionHooks {
public static function onParserFirstCallInit( Parser $parser ) {
$parser->setFunctionHook( 'example', [ self::class, 'renderExample' ] );
}
}
Parser interface
Controlling the parsing of output
To have the wikitext returned by your parser function be fully parsed (including expansion of templates), set the noparse
option to false
when returning:
return [ $output, 'noparse' => false ];
It seems the default value for noparse
changed from false
to true
, at least in some situations, sometime around version 1.12.
Conversely, to have your parser function return HTML that remains unparsed, rather than returning wikitext, use this:
return [ $output, 'noparse' => true, 'isHTML' => true ];
Naming
By default, MW adds a hash character (number sign, #
) to the name of each parser function.
To suppress that addition (and obtain a parser function with no #
prefix), include the SFH_NO_HASH constant in the optional flags argument to setFunctionHook, as described below.
When choosing a name without a hash prefix, note that transclusion of a page with a name starting with that function name followed by a colon is no longer possible. In particular, avoid function names equal to a namespace name. In the case that interwiki transclusion [1] is enabled, also avoid function names equal to an interwiki prefix.
The setFunctionHook hook
For more details of the interface into the parser, see the documentation for setFunctionHook in includes/Parser.php. Here's a (possibly dated) copy of those comments:
function setFunctionHook( $id, $callback, $flags = 0 )
Parameters:
- string $id - The magic word ID
- mixed $callback - The callback function (and object) to use
- integer $flags - Optional. Values:
- SFH_NO_HASH (1) constant if you call the function without
#
. - SFH_OBJECT_ARGS (2) if you pass a PPFrame object and array of arguments instead of a series of function arguments, for which see above.
- Defaults to 0 (no flags).
- SFH_NO_HASH (1) constant if you call the function without
Return value: The old callback function for this name, if any
Create a function, e.g., {{#sum:1|2|3}}
. The callback function should have the form:
function myParserFunction( $parser, $arg1, $arg2, $arg3 ) { ... }
The callback may either return the text result of the function, or an array with the text in element 0, and a number of flags in the other elements. The names of the flags are specified in the keys. Valid flags are:
Name | Type | Default | Description |
---|---|---|---|
found | Boolean | true
|
true if the text returned is valid and processing of the template must stop.
|
text | ? | ? | The text to return from the function. If isChildObj or isLocalObj are specified, this should be a DOM node instead. |
noparse | Boolean | true
|
true if text should not be preprocessed to a DOM tree, e.g. unsafe HTML tags should not be stripped, etc.
|
isHTML | Boolean | ? | true if the returned text is HTML and must be armoured against wikitext transformation. But see discussion
|
nowiki | Boolean | usually false
|
true if wiki markup in the return value (text) should be escaped.
|
isChildObj | Boolean | ? | true if the text is a DOM node needing expansion in a child frame.
|
isLocalObj | Boolean | ? | true if the text is a DOM node needing expansion in the current frame. The default value depends on other values and outcomes.
|
preprocessFlags | ? | false
|
Optional PPFrame flags to use when parsing the returned text. This only applies when noparse is false .
|
title | ? | false
|
The Title object where the text came from. |
forceRawInterwiki | Boolean | ? | true if interwiki transclusion must be forced to be done in raw mode and not rendered.
|
Expensive parser functions
Some parser functions represent a significant use of a wiki's resources and should be marked as "expensive". The number of expensive parser functions on any given page is limited by the $wgExpensiveParserFunctionLimit setting. What counts as expensive is left up to the function itself, but typically, anything that is likely to cause a delay that extends beyond simple processing of data should be considered. This includes things like database reads and writes, launching a shell script synchronously, or file manipulation. On the other hand, not all such functions should necessarily be tagged. Semantic MediaWiki, for example, only marks a percentage of its database reads as expensive. This is due to the fact that on certain data-intensive pages, it could easily overflow the normal expensive parser function limits. In cases like this, the potential for noticeably slower performance that doesn't get flagged as expensive is a trade-off to having the functionality that SMW offers.
To mark your parser function as expensive, from within the body of the function's code, use $result = $parser->incrementExpensiveFunctionCount();
.
The return value will be false
if the expensive function limit has been reached or exceeded.
Named parameters
Parser functions do not support named parameters the way templates and tag extensions do, but it is occasionally useful to fake it. Users are often accustomed to using vertical bars ( |
) to separate arguments, so it's nice to be able to do that in the parser function context, too. Here's a simple example of how to accomplish this:
function ExampleExtensionRenderParserFunction( &$parser ) {
// Suppose the user invoked the parser function like so:
// {{#myparserfunction: foo=bar | apple=orange | banana }}
$options = extractOptions( array_slice( func_get_args(), 1 ) );
// Now you've got an array that looks like this:
// [foo] => 'bar'
// [apple] => 'orange'
// [banana] => true
// Continue writing your code...
}
/**
* Converts an array of values in form [0] => "name=value"
* into a real associative array in form [name] => value
* If no = is provided, true is assumed like this: [name] => true
*
* @param array string $options
* @return array $results
*/
function extractOptions( array $options ) {
$results = [];
foreach ( $options as $option ) {
$pair = array_map( 'trim', explode( '=', $option, 2 ) );
if ( count( $pair ) === 2 ) {
$results[ $pair[0] ] = $pair[1];
}
if ( count( $pair ) === 1 ) {
$results[ $pair[0] ] = true;
}
}
return $results;
}
See also
General and related guides:
- Manual:Developing extensions , or for more general information about extensions, see Manual:Extensions and Extensions FAQ .
- Manual:Tag extensions
- Manual:Magic words
Code:
- Manual:Parser.php
- Manual:Hooks/ParserFirstCallInit
- Parser function hooks - an (incomplete) list of parser functions provided by core and extensions
- The Parser Hooks PHP library, which provides an object orientated interface for declarative parser hooks
- Manual:Extension data
Examples:
- The ParserFunctions extension is a well-known collection of parser functions.
- Help:Extension:ParserFunctions
- Category:Parser function extensions