Manual:Hooks
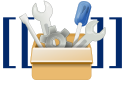
Hooks allow custom code to be executed when some defined event (such as saving a page or a user logging in) occurs.
For example, the following code snippet will trigger a call to the function MyExtensionHooks::onPageContentSaveComplete
whenever the PageContentSaveComplete
hook runs, passing it function arguments specific to PageContentSaveComplete .
Hooks can be registered by mapping the name of the hook to the callback in the extension's extension.json file:
"Hooks": {
"PageContentSaveComplete": "MyExtensionHooks::onPageContentSaveComplete",
"MyCustomHook": "MyExtensionHooks::myCustomHook"
}
MediaWiki provides many hooks like this to extend the functionality of the MediaWiki software. Assigning a function (known as an hook handler) to a hook will cause that function to be called at the appropriate point in the main MediaWiki code, to perform whatever additional task(s) the developer thinks would be useful at that point. Each hook can have multiple handlers assigned to it, in which case it will call the functions in the order that they are assigned, with any modifications made by one function passed on to subsequent functions in the chain.
Assign functions to hooks at the end of LocalSettings.php or in your own extension file at the file scope (not in a $wgExtensionFunctions
function or the ParserFirstCallInit hook).
For extensions, if the hook function's behaviour is conditioned on a setting in LocalSettings.php, the hook should be assigned and the function should terminate early if the condition was not met.
You can also create new hooks in your own extension.
It is registered in extension.json the same way as if you were registering a built-in MediaWiki hook to use in your extension.
You can then run your hook within your extension by calling HookContainer::run( 'HookName', [ $param1, $param2 ] );
.
Lastly, don't forget to add them to Category:Extension hooks .
Background
A hook is triggered by a call to HookContainer::run
, usually via a method in HookRunner.
HookContainer will find the hook handlers to run and call them with the parameters given to HookContainer::run
.
Hook handlers are registered via extension.json .
See also Hooks.md.
In this example from the doPurge
function in WikiPage.php, doPurge calls HookRunner::onArticlePurge
to run the ArticlePurge hook, passing $this
as argument:
$this->getHookRunner()->onArticlePurge( $this )
The Core calls many hooks, but Extensions can also call hooks.
Writing a hook handler
A hook handler is a function you register, which will be called whenever the hook in question is run.
For extensions, register your hook handlers in extension.json
:
{
"Hooks": {
"EventName": [
"MyExtensionHooks::onEventName",
"someFunction"
]
}
}
Hook handlers can also be registered via the global $wgHooks
array.
This is most commonly used for site-specific customisations in LocalSettings.php, or in legacy extensions that predate extension.json
.
All the following are valid ways to define a hook handler for the EventName hook, with two parameters passed:
Format | Syntax | Resulting function call. |
---|---|---|
Static function | $wgHooks['EventName'][] = 'MyExtensionHooks::onEventName';
|
MyExtensionHooks::onEventName( $param1, $param2 );
|
Function, no data | $wgHooks['EventName'][] = 'efSomeFunction';
|
efSomeFunction( $param1, $param2 );
|
Function with data | $wgHooks['EventName'][] = [ 'efSomeFunction', $someData ];
|
efSomeFunction( $someData, $param1, $param2 );
|
Inline anonymous function |
$wgHooks['EventName'][] = function ( $param1, $param2 ) {
// ...function body
};
|
|
Object only | $wgHooks['EventName'][] = $object;
|
$object->onEventName( $param1, $param2 );
|
Object with method | $wgHooks['EventName'][] = [ $object, 'someMethod' ];
|
$object->someMethod( $param1, $param2 );
|
Object with method and data | $wgHooks['EventName'][] = [ $object, 'someMethod', $someData ];
|
$object->someMethod( $someData, $param1, $param2 );
|
Note that when an object is assigned, and you don't specify a method, the method called is "onEventName". For example "onArticleSave", "onUserLogin", etc.
The optional data is useful if you want to use the same function or object for different purposes. For example:
$wgHooks['PageContentSaveComplete'][] = [ 'efIrcNotify', 'TimStarling' ];
$wgHooks['PageContentSaveComplete'][] = [ 'efIrcNotify', 'brion' ];
This code would result in efIrcNotify()
being run twice when a page is saved: once for 'TimStarling', and once for 'brion'.
Hook handler return values
Hooks fall into one of two categories, distinguished by the supported return values:
MediaWiki version: | ≥ 1.23 |
Void hooks
A void hook does not return anything. Void hook should be the most common kind in new code (T245364).
- Documentation:
@return void
- Usage:
$hookRunner->onExample();
The interface for void hooks must include a native return type hint like : void
, so that extensions that choose to implement the interface (as opposed to handling it without interface validation), benefit from static analysis and thus avoid accidentally returning something.
This prevents severe bugs that are hard to catch otherwise (T173615). These hooks ignore the return value and so in isolation, a return value is simply unused, harmless, with no visible effect.
In production, it would cancel listeners from other extensions for the same hook.
Effectively undeploying only part of an extension this way can have major consequeces.
Abortable hooks
Abortable hooks may return boolean false.
When a non-void return value is given to an abortable hook, it skips any and all other listeners for the same hook (i.e. in other extensions), and returns false to the hook caller.
This is similar to Event.stopImmediatePropagation()
and Event.preventDefault()
in JavaScript.
If as the author of a feature, you want to allow an extension to disallow or cancel the user action, you can provide an abortable hook for this.
- Documentation:
@return bool|void
- Usage:
if ( $hookRunner->onExample() ) { … }
It is important that abortable hooks allow a void return. The majority of hook consumers do not make use of the abortable feature, and thus should be able to type their implementation to @return void
and safely avoid the need to return something, and with it benefit from having no room for mistakingly returning false.
Return values:
void
(no return value, ornull
) - the hook handler finished successfully. (Before MediaWiki 1.23, returningtrue
was required.)
false
- the hook handler has done all the work necessary, or replaced normal handling. This will prevent further handlers from being run, and in some cases tells the calling function to skip normal processing.
MediaWiki version: | ≤ 1.40 |
Prior to MediaWiki 1.41, abortable hooks could return an error message as string. This was a shortcut for producing an Internal Server Error (HTTP 500) response with the returned string displayed as the fatal error. This was deprecated in MW 1.35 (Gerrit change 571297), and removed in MW 1.41 (Gerrit change 571297).
Handling hooks in MediaWiki 1.35 and later
MediaWiki version: | ≥ 1.35 |
MediaWiki 1.35 introduces the HookHandlers extension attribute. This includes per-hook interfaces for improved static validation and discovery of parameter documentation. It also enables dependency injection by introducing an intermediary class instance that accepts a number of specified services (instead of static callbacks that explicitly access services from global state).
The approach from MediaWiki 1.34 and earlier, of registering hook handlers directly as static methods, remains supported and is not deprecated. Extension authors may opt-in to the new system are welcome to do so. To learn more, see the MediaWiki core: Hook specification and the announcement on wikitech-l.
Changes to hook names
Prior to MediaWiki 1.35, hooks sometimes included characters that could not be used in a class or method name, such as colons and dashes. With the introduction of per-hook interfaces, the canonical names of these hooks have been changed to use underscores instead. For example, the interface for ApiFeedContributions::feedItem is ApiFeedContributions__feedItemHook. Hook handlers that are registered with the old names remain supported.
Registering hooks using HookHandlers
To adopt the new system, change your Hooks class to have regular methods instead of static methods and to be constructible.
This class is then registered once, via the HookHandlers attribute in extension.json , using the class
option as part of an ObjectFactory description where you can use the services
option.
For example, to register the BeforePageDisplay hook:
{
"HookHandlers": {
"main": {
"class": "MediaWiki\\Extension\\Example\\Hooks",
"services": [ "UserNameUtils" ]
}
},
"Hooks": {
"BeforePageDisplay": "main"
}
}
Handling hooks using interfaces
To use hook interfaces, extensions should define a Hooks class in their namespace and implement one or more hook interfaces. Hook interfaces are named with the hook name followed by the word "Hook".
namespace MediaWiki\Extension\MyExtension;
use MediaWiki\Hook\BeforePageDisplayHook;
use OutputPage;
use Skin;
class Hooks implements BeforePageDisplayHook {
public function onBeforePageDisplay( $out, $skin ): void { ... }
}
Convert an extension to the new hook system
Follow these steps for each hook handling method:
- identify the hook handler interface, and make the Hooks class implement this interface.
- update the method name and signature to be exactly the same as in the interface.
- change the Hooks section of extension.json to refer to the handler you specified in the HookHandlers section.
The process was demonstrated at the Wikimedia Hackathon 2021 :
Documentation
Currently, hooks in MediaWiki core have to be documented both in hook interface (in the source code repository) and here on MediaWiki.org. In some cases, one of these steps may not yet have been completed, so if a hook appears undocumented, check both.
Each hook provided by MediaWiki Core is defined in a hook interface. Typically, hook interfaces are located in a "Hook" sub-namespace inside the caller namespace. For example, Storage/Hook/PageContentSaveCompleteHook.php. You can find a list of hook interfaces in the generated MediaWiki PHP documentation.
To document a hook on-wiki, use {{MediaWikiHook }}.
Hook interface doc template
In hook interfaces, doc comments specify the status, purpose, parameters, and behaviour of the hook.
/**
* @stable for implementation
* @ingroup Hooks
*/
interface MyExampleHook {
/**
* This hook is called after/when...
* Use this hook to...
*
* @since x.xx
* @param string $name Description
* @return void This hook must not abort, it must return no value
*/
public function onMyExample( $name ): void;
}
/**
* @stable for implementation
* @ingroup Hooks
*/
interface AbortableExampleHook {
/**
* This hook is called before...
* Use this hook to...
*
* @since x.xx
* @param string $name Description
* @@return bool|void True or no return value to continue or false to abort
*/
public function oAbortableExample( $name );
}
Available hooks
Hooks grouped by function
Some of these hooks can be grouped into multiple functions.
- Sections: Article Management - Edit Page - Page Rendering - User Interface - File Management - Special Pages - User Management - Logging - Skinning Templates - API - Import/Export - Diffs - Miscellaneous
Function | Version | Hook | Description |
---|---|---|---|
Article Management | 1.23.0 | Article::MissingArticleConditions | Called when showing a page. |
1.21 | ArticleAfterFetchContentObject | (deprecated in 1.32) After fetching content of an article from the database. | |
1.16 | ArticleConfirmDelete | Occurs before writing the confirmation form for article deletion. | |
1.21 | ArticleContentViewCustom | (removed in 1.35) Allows to output the text of the article in a different format than wikitext | |
1.25 | ArticleDeleteAfterSuccess | Output after an article has been deleted | |
1.4.0 | ArticleDeleteComplete | (deprecated in 1.37) Occurs after the delete article request has been processed | |
1.4.0 | ArticleDelete | (deprecated in 1.37) Occurs whenever the software receives a request to delete an article | |
1.5.7 | ArticleEditUpdateNewTalk | Allows an extension to prevent user notification when a new message is added to their talk page. | |
1.6.0 | ArticleEditUpdatesDeleteFromRecentchanges | Occurs before saving to the database. If returning false old entries are not deleted from the recentchangeslist.
| |
1.14.0 | ArticleEditUpdates | (deprecated in 1.35) Called when edit updates (mainly link tracking) are made when an article has been changed. | |
1.8.0 | ArticleFromTitle | Called to determine the class to handle the article rendering, based on title | |
1.12.0 | ArticleMergeComplete | After merging to article using Special:Mergehistory | |
1.36 | ArticleParserOptions | This hook is called before parsing wikitext for an article, | |
1.18 | ArticlePrepareTextForEdit | Called when preparing text to be saved. | |
1.4.0 | ArticleProtectComplete | Occurs after the protect article request has been processed | |
1.4.0 | ArticleProtect | Occurs whenever the software receives a request to protect an article | |
1.6.0 | ArticlePurge | Allows an extension to cancel a purge. | |
1.12.0 | ArticleRevisionUndeleted | (deprecated in 1.35) Occurs after an article revision is restored | |
1.32 | ArticleRevisionViewCustom | Allows to output the text of an article revision in a different format than wikitext | |
1.13.0 | ArticleRevisionVisibilitySet | Called when changing visibility of one or more revisions of an article | |
1.12.0 | ArticleRollbackComplete | (deprecated in 1.35) Occurs after an article rollback is completed | |
1.21 | ArticleUndeleteLogEntry | Occurs when a log entry is generated but not yet saved | |
1.9.1 | ArticleUndelete | When one or more revisions of an article are restored | |
1.11.0 | ArticleUpdateBeforeRedirect | Occurs after a page is updated (usually on save), before the user is redirected back to the page | |
1.18 | ArticleViewFooter | After showing the footer section of an ordinary page view. | |
1.17 | CanonicalNamespaces | For extensions adding their own namespaces or altering the defaults. | |
1.25 | ChangeTagAfterDelete | Called after a change tag has been deleted (that is, removed from all revisions and log entries to which it was applied). | |
1.25 | ChangeTagCanCreate | Tell whether a change tag should be able to be created by users. | |
1.25 | ChangeTagCanDelete | Tell whether a change tag should be able to be deleted by users. | |
1.28 | ChangeTagsAfterUpdateTags | Can be used by extensions to take actions after change tags have been added or updated. | |
1.30 | ChangeTagsAllowedAdd | Called when checking if a user can add tags to a change. | |
1.25 | ChangeTagsListActive | Can be used by extensions to register active change tags. | |
1.21.0 | ContentHandlerDefaultModelFor | Called when deciding the default content model for a given title. | |
1.21.0 | ContentHandlerForModelID | Called when a ContentHandler is requested for a given content model name, but no entry for that model exists in $wgContentHandlers . | |
1.23 | ContentModelCanBeUsedOn | args = $modelId, Title $title, &$ok | |
1.21.0 | ConvertContent | Called when a conversion to another content model is requested. | |
1.29 | GetContentModels | Allows to add custom content handlers to the list of content models registered with the system. | |
1.39 | JsonValidateSave | Use this hook to add additional validations for JSON content pages. | |
1.19 | LanguageGetNamespaces | Provide custom ordering for namespaces or remove namespaces. | |
1.25.0 | MovePageIsValidMove | Specify whether a page can be moved for technical reasons. | |
1.35.0 | MultiContentSave | Occurs whenever the software receives a request to save an article | |
1.25 | MovePageCheckPermissions | Specify whether the user is allowed to move the page. | |
1.20 | NamespaceIsMovable | Called when determining if it is possible to move pages, for a particular namespace. This controls moves both to and from the given namespace. | |
1.13 | NewRevisionFromEditComplete | (deprecated in 1.35) Called when a revision was inserted due to an edit. | |
1.21 | PageContentInsertComplete | (deprecated in 1.35) Occurs after a new article is created | |
1.18 | PageContentLanguage | Allows changing the page content language and consequently all features depending on that (writing direction, LanguageConverter, ...). | |
1.21.0 | PageContentSaveComplete | (deprecated in 1.35) Occurs after the save article request has been processed | |
1.21.0 | PageContentSave | (deprecated in 1.35) (use MultiContentSave) Occurs whenever the software receives a request to save an article | |
1.37.0 | PageDelete | Occurs whenever the software receives a request to delete a page. | |
1.37.0 | PageDeleteComplete | Occurs after the delete page request has been processed. | |
1.32 | PageDeletionDataUpdates | Called when constructing a list of DeferrableUpdate to be executed when a page is deleted. | |
1.35 | PageMoveComplete | After an article has been moved, post commit | |
1.35 | PageMoveCompleting | After an article has been moved, pre commit | |
1.35 | PageSaveComplete | After an article has been updated. | |
1.37 | PageUndelete | Run before page undeletion. | |
1.40 | PageUndeleteComplete | Runs after a page undeletion. | |
1.25 | PageViewUpdates | Called after a page view is seen by MediaWiki. Note this does not capture views made via external caches such as Squid . | |
1.16 | ProtectionForm::buildForm | (deprecated in 1.36) Called after all protection type fieldsets are made in the form. | |
1.16 | ProtectionForm::save | Called when a protection form is submitted. | |
1.16 | ProtectionForm::showLogExtract | Called after the protection log extract is shown. | |
1.36 | ProtectionFormAddFormFields | This hook is called after all protection type form fields are added. | |
1.8.0 | RecentChange_save | Called after a "Recent Change" is committed to the DB. | |
1.32 | RevisionDataUpdates | Called when constructing a list of DeferrableUpdate to be executed to record secondary data about a revision. | |
1.35 | RevisionFromEditComplete | Called when a revision was inserted due to an edit, file upload, import or page move. | |
1.11.0 | RevisionInsertComplete | (deprecated in 1.31) (use RevisionRecordInserted) Called after a revision is inserted into the DB | |
1.31 | RevisionRecordInserted | Called after a revision is inserted into the database. | |
1.35 | RevisionUndeleted | Called after an article revision is restored | |
1.35 | RollbackComplete | (deprecated in 1.36) Occurs after an article rollback is completed | |
1.15 | ListDefinedTags | Can be used by extensions to register change tags. | |
1.6.0 | SpecialMovepageAfterMove | Called after a page is moved. | |
1.14 | TitleArrayFromResult | Called when creating an TitleArray object from a database result. | |
1.24 | TitleExists | Called when determining whether a page exists at a given title. | |
1.22 | TitleGetEditNotices | Called when the edit notices for a page are being retrieved. | |
1.16 | TitleGetRestrictionTypes | Allows to modify the types of protection that can be applied. | |
1.20.0 | TitleIsAlwaysKnown | Allows overriding default behaviour for determining if a page exists. | |
1.19 | TitleIsMovable | Called when determining if it is possible to move a page. | |
1.4.0 | TitleMoveComplete | (deprecated in 1.35) Occurs whenever a request to move an article is completed | |
1.27 | TitleMoveCompleting | (deprecated in 1.35) Occurs whenever a request to move an article is completed, before the database transaction commits. | |
1.27 | TitleMoveStarting | Before moving an article (title), but just after the atomic DB section starts. | |
1.22.0 | TitleMove | Occurs before a requested pagemove is performed | |
1.22 | TitleQuickPermissions | Called from Title::checkQuickPermissions to allow skipping checking quick Title permissions (e.g., the 'delete' permission). | |
1.19 | TitleReadWhitelist | Called at the end of read permissions checks, just before adding the default error message if nothing allows the user to read the page. | |
1.18 | UndeleteForm::undelete | (removed in 1.38) Called un UndeleteForm::undelete, after checking that the site is not in read-only mode, that the Title object is not null and after a PageArchive object has been constructed but before performing any further processing.
| |
1.21 | WikiPageDeletionUpdates | (removed in 1.36) Manipulate the list of DeferrableUpdate s to be applied when a page is deleted. Called in WikiPage::getDeletionUpdates(). Note that updates specific to a content model should be provided by the respective Content's getDeletionUpdates() method.
| |
1.28 | WikiPageFactory | Override WikiPage class used for a title | |
Edit Page | 1.21.0 | AlternateEditPreview | Allows replacement of the edit preview |
1.6.0 | AlternateEdit | Used to replace the entire edit page, altogether. | |
1.9.1 | CustomEditor | When invoking the page editor. Return true to allow the normal editor to be used, or false if implementing a custom editor, e.g. for a special namespace, etc.
| |
1.21.0 | EditFilterMergedContent | Post-section-merge edit filter | |
1.6.0 | EditFilter | Perform checks on an edit | |
1.16 | EditFormInitialText | Allows modifying the edit form when editing existing pages | |
1.7.0 | EditFormPreloadText | Called when edit page for a new article is shown. This lets you fill the text-box of a new page with initial wikitext. | |
1.25 | EditPage::attemptSave:after | Called after an article save attempt | |
1.8.3 | EditPage::attemptSave | Called before an article is saved, that is before insertNewArticle() is called
| |
1.16 | EditPage::importFormData | Called when reading the data from the editform, after post | |
1.6.0 | EditPage::showEditForm:fields | Allows injection of form field into edit form. | |
1.6.0 | EditPage::showEditForm:initial | Allows injection of HTML into edit form | |
1.24 | EditPage::showReadOnlyForm:initial | Called just before the read only edit form is rendered | |
1.21.0 | EditPage::showStandardInputs:options | Allows injection of form fields into the editOptions area | |
1.13.0 | EditPageBeforeConflictDiff | Allows modifying the EditPage object and output when there's an edit conflict. | |
1.12.0 | EditPageBeforeEditButtons | Used to modify the edit buttons on the edit form | |
1.16 | EditPageBeforeEditToolbar | Allows modifying the edit toolbar above the textarea | |
1.16 | EditPageCopyrightWarning | Allow for site and per-namespace customisation of contribution/copyright notice. | |
1.29 | EditPageGetCheckboxesDefinition | Allows modifying the edit checkboxes in the edit form | |
1.21 | EditPageGetDiffContent | Allow modifying the wikitext that will be used in "Show changes". Note that it is preferable to implement diff handling for different data types using the ContentHandler facility. | |
1.21 | EditPageGetPreviewContent | Allow modifying the wikitext that will be previewed. Note that it is preferable to implement previews for different data types using the ContentHandler facility. | |
1.16 | EditPageNoSuchSection | When a section edit request is given for an non-existent section. | |
1.16 | EditPageTosSummary | Give a chance for site and per-namespace customisations of terms of service summary link that might exist separately from the copyright notice. | |
1.20 | FormatAutocomments | When an autocomment is formatted by the Linker. | |
1.19 | PlaceNewSection | Override placement of new sections. | |
1.35 | ParserPreSaveTransformComplete | Called from Parser::preSaveTransform() after processing is complete, giving the extension a chance to further modify the wikitext.
| |
Page Rendering | 1.27.0 | AfterBuildFeedLinks | Executed after all feed links are created. |
1.24 | AfterParserFetchFileAndTitle | Alter the HTML code of an image gallery. Called after an image gallery is formed by Parser , just before adding its HTML to parser output. | |
1.21 | ArticleContentViewCustom | (removed in 1.35) Allows to output the text of the article in a different format than wikitext | |
1.6.0 | ArticlePageDataAfter | Executes after loading the data of an article from the database. | |
1.6.0 | ArticlePageDataBefore | Executes before data is loaded for the article requested. | |
1.36 | ArticleParserOptions | This hook is called before parsing wikitext for an article, | |
1.32 | ArticleRevisionViewCustom | Allows to output the text of an article revision in a different format than wikitext | |
1.18 | ArticleViewFooter | After showing the footer section of an ordinary page view. | |
1.6.0 | ArticleViewHeader | Called after an articleheader is shown. | |
1.5.1 | ArticleViewRedirect | Allows an extension to prevent the display of a "Redirected From" link on a redirect page. | |
1.7 | BadImage | Used to determine if an image exists on the 'bad image list'. Return false to when setting $bad value.
| |
1.19 | BeforeDisplayNoArticleText | Before displaying noarticletext or noarticletext-nopermission messages. | |
1.24 | BeforeHttpsRedirect | (deprecated in 1.35) Called prior to forcing HTTP->HTTPS redirect. Use this hook to override how the redirect is output. | |
1.19 | BeforePageRedirect | Called prior to sending an HTTP redirect | |
1.18.0 | BeforeParserFetchFileAndTitle | Allows an extension to select a different version of an image to link to. | |
1.10.1 | BeforeParserFetchTemplateAndtitle | (deprecated in 1.36) Allows an extension to specify a version of a page to get for inclusion in a template. | |
1.36 | BeforeParserFetchTemplateRevisionRecord | This hook is called before a template is fetched by Parser. | |
1.10.1 | BeforeParserrenderImageGallery | (deprecated in 1.35) Allows an extension to modify an image gallery before it is rendered. | |
1.22 | CanIPUseHTTPS | (deprecated in 1.35) Called when checking if an IP address can use HTTPS | |
1.4.3 | CategoryPageView | Called before viewing a categorypage in CategoryPage::view
| |
1.25 | CategoryViewer::doCategoryQuery | Occurs after querying for pages to be displayed in a Category page | |
1.25 | CategoryViewer::generateLink | Before generating an output link allow extensions opportunity to generate a more specific or relevant link. | |
1.25 | ContentAlterParserOutput | Customise parser output for a given content object, called by AbstractContent::getParserOutput .
| |
1.24.0 | ContentGetParserOutput | Customise parser output for a given content object, called by AbstractContent::getParserOutput . May be used to override the normal model-specific rendering of page content.
| |
1.22.0 | GalleryGetModes | Allows extensions to add classes that can render different modes of a gallery. | |
1.12 | GetLinkColours | Modify the CSS class of an array of page links | |
1.28 | HtmlPageLinkRendererBegin | args = LinkRenderer $linkRenderer, LinkTarget $target, &$text, &$extraAttribs, &$query, &$ret | |
1.28 | HtmlPageLinkRendererEnd | args = LinkRenderer $linkRenderer, LinkTarget $target, $isKnown, &$text, &$attribs, &$ret | |
1.13 | ImageBeforeProduceHTML | Called before producing the HTML created by a wiki image insertion | |
1.11 | ImageOpenShowImageInlineBefore | Fired just before showing the image on an image page. | |
1.16 | ImagePageAfterImageLinks | Called after the image links section on an image page is built. | |
1.13 | ImagePageFileHistoryLine | Called when a file history line is constructed. | |
1.13 | ImagePageFindFile | Called when fetching the file associated with an image page. | |
1.16 | ImagePageShowTOC | Called when fetching the file associed with an image page. | |
1.10.0 | InternalParseBeforeLinks | Used to process the expanded wiki code after <nowiki> , HTML-comments, and templates have been treated. Suitable for syntax extensions that want to customise the treatment of internal link syntax, i.e. [[....]] .
| |
1.20 | InternalParseBeforeSanitize | (deprecated in 1.35) This hook is called during Parser's internalParse method just before the parser removes unwanted/dangerous HTML tags and after nowiki/noinclude/includeonly/onlyinclude and other processings.
| |
1.13.0 | LinkerMakeExternalImage | Called before external image HTML is returned. Used for modifying external image HTML. | |
1.13.0 | LinkerMakeExternalLink | Called before the HTML for external links is returned. Used for modifying external link HTML. | |
1.23.0 | LinkerMakeMediaLinkFile | Called before the HTML for media links is returned. Used for modifying media link HTML. | |
1.6.0 | OutputPageBeforeHTML | Called every time wikitext is added to the OutputPage, after it is parsed but before it is added. Called after the page has been rendered, but before the HTML is displayed. | |
1.8.0 | OutputPageParserOutput | Called after parse, before the HTML is added to the output. | |
1.6.0 | PageRenderingHash | Alter the parser cache option hash key. | |
1.20 | ParserAfterParse | Called from Parser::parse() just after the call to Parser::internalParse() returns.
| |
1.5.0 | ParserAfterStrip | (removed in 1.36) Before version 1.14.0, used to process raw wiki code after text surrounded by <nowiki> tags have been protected but before any other wiki text has been processed. In version 1.14.0 and later, runs immediately after ParserBeforeStrip.
| |
1.5.0 | ParserAfterTidy | Used to add some final processing to the fully-rendered page output. | |
1.26 | ParserAfterUnstrip | Called after the first unstripGeneral() in Parser::internalParseHalfParsed()
| |
1.6.0 | ParserBeforeInternalParse | Replaces the normal processing of stripped wiki text with custom processing. Used primarily to support alternatives (rather than additions) to the core MediaWiki markup syntax. | |
1.35 | ParserBeforePreprocess | Called at the beginning of Parser::preprocess()
| |
1.5.0 | ParserBeforeStrip | (removed in 1.36) Used to process the raw wiki code before any internal processing is applied. | |
1.5.0 | ParserBeforeTidy | (removed in 1.36) Used to process the nearly-rendered html code for the page (but before any html tidying occurs). | |
1.26 | ParserCacheSaveComplete | Modify ParserOutput safely after it has been saved to cache. | |
1.6.0 | ParserClearState | Called at the end of Parser::clearState()
| |
1.21.0 | ParserCloned | Called when the Parser object is cloned. | |
1.28 | ParserFetchTemplate | (deprecated in 1.35) Called when the parser fetches a template | |
1.6.0 | ParserGetVariableValueSwitch | Assigns a value to a user defined variable. | |
1.6.0 | ParserGetVariableValueTs | Use this to change the value of the time for the {{LOCAL...}} magic word. | |
1.6.0 | ParserGetVariableValueVarCache | (deprecated in 1.35) Use this to change the value of the variable cache or return false to not use it. | |
1.35 | ParserFetchTemplateData | Fetches template data for an array of template titles | |
1.22 | ParserLimitReportFormat | Called for each row in the parser limit report that needs formatting. | |
1.22 | ParserLimitReportPrepare | Called at the end of Parser:parse() when the parser will include comments about size of the text parsed.
| |
1.39 | ParserLogLinterData | Report lints from Parsoid to the Linter extension | |
1.12 | ParserMakeImageParams | Alter the parameters used to generate an image before it is generated | |
1.38 | ParserModifyImageHTML | This hook is called for each image added to parser output, with its associated HTML as returned from Linker::makeImageLink(). | |
1.30 | ParserOptionsRegister | Allows registering additional parser options | |
1.31 | ParserOutputPostCacheTransform | Called from ParserOutput::getText() to do post-cache transforms.
| |
1.27 | ParserOutputStashForEdit | Called when an edit stash parse finishes, before the output is cached. | |
1.35 | ParserPreSaveTransformComplete | Called from Parser::preSaveTransform() after processing is complete, giving the extension a chance to further modify the wikitext.
| |
1.19 | ParserSectionCreate | (deprecated in 1.35) Called each time the parser creates a document section from wikitext. | |
1.26 | RejectParserCacheValue | Return false to reject an otherwise usable cached value from the Parser cache. | |
1.26 | ResourceLoaderForeignApiModules | Called from ResourceLoaderForeignApiModule. Use this to add dependencies to mediawiki.ForeignApi module when you wish to override its behaviour. See the module docs for more information. | |
1.17 | ResourceLoaderGetConfigVars | Called right before ResourceLoaderStartUpModule::getConfig returns, to set static (not request-specific) configuration variables. Can not depend on current page, current user or current request; see below.
| |
1.18 | ResourceLoaderGetStartupModules | Run once the startup module is being generated. | |
1.38 | ResourceLoaderExcludeUserOptions | Exclude a user option from the preloaded data for client-side mw.user.options. | |
1.29 | ResourceLoaderJqueryMsgModuleMagicWords | Called in ResourceLoaderJqueryMsgModule to allow adding magic words for jQueryMsg. The key is an all-caps magic word, and the value is a string; values depend only on the ResourceLoaderContext | |
1.17.0 | ResourceLoaderRegisterModules | Allows registering modules with ResourceLoader | |
1.35 | ResourceLoaderSiteModulePages | Allows to change which wiki pages comprise the `site` module in given skin.
| |
1.35 | ResourceLoaderSiteStylesModulePages | Allows to change which wiki pages comprise the 'site.styles' module in given skin.
| |
1.19 | ResourceLoaderTestModules | (deprecated in 1.33) Add new javascript test suites. This is called after the addition of MediaWiki core test suites. | |
1.24.0 | SidebarBeforeOutput | Directly before the sidebar is output | |
1.16 | ShowMissingArticle | Called when generating the output for a non-existent page. | |
User Interface | 1.18 | ActionBeforeFormDisplay | Before executing the HTMLForm object |
1.18 | ActionModifyFormFields | Before creating an HTMLForm object for a page action; allows to change the fields on the form that will be generated. | |
1.20 | AfterFinalPageOutput | Nearly at the end of OutputPage::output() .
| |
1.9.1 | AjaxAddScript | Called in output page just before the initialisation | |
1.5.7 | ArticleEditUpdateNewTalk | Before updating user_newtalk when a user talk page was changed.
| |
1.6.0 | ArticlePurge | Executes before running &action=purge
| |
1.32 | ArticleShowPatrolFooter | Can be used to hide the [mark as patrolled] link in certain circumstances | |
1.18.0 | BeforeWelcomeCreation | Allows an extension to change the message displayed upon a successful login. | |
1.32 | ContentSecurityPolicyDefaultSource | Modify the allowed CSP load sources. This affects all directives except for the script directive. | |
1.32 | ContentSecurityPolicyDirectives | Modify the content security policy directives. | |
1.32 | ContentSecurityPolicyScriptSource | Modify the allowed CSP script sources. | |
1.4.0 | EmailUserComplete | Occurs after an email has been sent from one user to another | |
1.4.0 | EmailUser | Occurs whenever the software receives a request to send an email from one user to another | |
1.41 | EmailUserAuthorizeSend | Called when checking whether a user is allowed to send emails | |
1.41 | EmailUserSendEmail | Called before sending an email, when all other checks have succeeded. | |
1.21 | GetHumanTimestamp | Pre-emptively override the human-readable timestamp generated by MWTimestamp::getHumanTimestamp() . Return false in this hook to use the custom output.
| |
1.31 | GetLangPreferredVariant | Allows fetching the language variant code from cookies or other such alternative storage. | |
1.22 | GetNewMessagesAlert | Disable or modify the new messages alert before it is shown | |
1.16 | GetPreferences | Modify user preferences. | |
1.22 | GetRelativeTimestamp | Pre-emptively override the relative timestamp generated by MWTimestamp::getRelativeTimestamp(). Return false in this hook to use the custom output. | |
1.32 | HistoryPageToolLinks | Allows adding links to the revision history page subtitle. | |
1.21 | HistoryRevisionTools | (deprecated in 1.35) Override or extend the revision tools available from the page history view, i.e. undo, rollback, etc. | |
1.35 | HistoryTools | Use this hook to override or extend the revision tools available from the page history view, i.e. undo, rollback, etc. | |
1.24 | LanguageSelector | Hook to change the language selector available on a page. | |
1.6.0 | MarkPatrolledComplete | Called after an edit is marked patrolled | |
1.6.0 | MarkPatrolled | Called before an edit is marked patrolled | |
1.14 | MakeGlobalVariablesScript | Right before OutputPage->getJSVars returns the vars.
| |
1.32 | OutputPageAfterGetHeadLinksArray | Allows extensions to modify the HTML metadata in the <head> element
| |
1.17 | OutputPageBodyAttributes | Called when OutputPage::headElement() is creating the body tag.
| |
1.14 | OutputPageCheckLastModified | When checking if the page has been modified since the last visit | |
1.13 | OutputPageMakeCategoryLinks | Called when links are about to be generated for the page's categories. | |
1.10 | PageHistoryBeforeList | When a history page list is about to be constructed. | |
1.10 | PageHistoryLineEnding | Right before the end <li> is added to a history line.
| |
1.26 | PageHistoryPager::doBatchLookups | Allow performing batch lookups for prefetching information needed for display | |
1.13 | PageHistoryPager::getQueryInfo | When a history pager query parameter set is constructed. | |
1.10 | RawPageViewBeforeOutput | Called before displaying a page with action=raw . Returns true if display is allowed, false if display is not allowed.
| |
1.16 | ShowSearchHitTitle | Customise display of search hit title/link. | |
1.21 | ShowSearchHit | Customise display of search hit. | |
1.7 | SiteNoticeAfter | Used to modify the site notice after it has been created from $wgSiteNotice or interface messages.
| |
1.7 | SiteNoticeBefore | Used to modify the sitenotice before it has been created from $wgSiteNotice. Return false to suppress or modify default site notice. | |
1.6.0 | SpecialMovepageAfterMove | Called after a page is moved. | |
1.19.0 | SpecialSearchCreateLink | Called when making the message to create a page or go to an existing page | |
1.6.0 | SpecialSearchNogomatch | Called when the 'Go' feature is triggered and the target doesn't exist. Full text search results are generated after this hook is called | |
1.19.0 | SpecialSearchPowerBox | The equivalent of SpecialSearchProfileForm for the advanced form | |
1.34 | UndeletePageToolLinks | Add one or more links to edit page subtitle when a page has been previously deleted. | |
1.4.0 | UnwatchArticleComplete | Occurs after the unwatch article request has been processed | |
1.4.0 | UnwatchArticle | Occurs whenever the software receives a request to unwatch an article | |
1.5.7 | UserClearNewTalkNotification | Called when clearing the "You have new messages!" message, return false to not delete it | |
1.4.0 | UserLoginComplete | Occurs after a user has successfully logged in | |
1.4.0 | UserLogoutComplete | Occurs after a user has successfully logged out | |
1.4.0 | UserLogout | Occurs when the software receives a request to log out | |
1.5.7 | UserRetrieveNewTalks | (deprecated in 1.35) Called when retrieving "You have new messages!" message(s) | |
1.19 | UserToolLinksEdit | Called when generating a list of user tool links, eg "Foobar (talk | contribs | block)"
| |
1.4.0 | WatchArticleComplete | Occurs after the watch article request has been processed | |
1.4.0 | WatchArticle | Occurs whenever the software receives a request to watch an article | |
File Management | 1.19 | BitmapHandlerCheckImageArea | Called by BitmapHandler::normaliseParams() , after all normalisations have been performed, except for the $wgMaxImageArea check.
|
1.18 | BitmapHandlerTransform | Before a file is transformed, gives extension the possibility to transform it themselves. | |
1.13 | FileDeleteComplete | When a file is deleted. | |
1.20 | FileTransformed | When a file is transformed and moved into storage. | |
1.13 | FileUndeleteComplete | When a file is undeleted. | |
1.11 | FileUpload | Fires when a local file upload occurs. | |
1.18 | GetMetadataVersion | Allows to modify the image metadata version currently in use. | |
1.23.0 | GetExtendedMetadata | Allows including additional file metadata information in the imageinfo API. | |
1.24 | HTMLFileCache::useFileCache | Override whether a page should be cached in file cache. | |
1.10.0 | IsFileCacheable | Allow an extension to disable file caching on pages. | |
1.22 | IsUploadAllowedFromUrl | Allows overriding the result of UploadFromUrl::isAllowedUrl() .
| |
1.16.0 | ImgAuthBeforeStream | Executed before file is streamed to user, but only when using img_auth | |
1.34 | ImgAuthModifyHeaders | Executed just before a file is streamed to a user, allowing headers to be modified beforehand. | |
1.13 | LocalFile::getHistory | Called before file history query performed. | |
1.19 | LocalFilePurgeThumbnails | Called before thumbnails for a local file are purged. | |
1.21 | ThumbnailBeforeProduceHTML | Called before an image HTML is about to be rendered (by ThumbnailImage:toHtml method). | |
1.16 | UploadCreateFromRequest | When UploadBase::createFromRequest has been called.
| |
1.9.0 | UploadForm:BeforeProcessing | Called just before the file data (for example description) are processed, so extensions have a chance to manipulate them. | |
1.31 | UploadForm:getInitialPageText | After the initial page text for file uploads is generated, to allow it to be altered. | |
1.16 | UploadFormInitDescriptor | Occurs after the descriptor for the upload form as been assembled. | |
1.16 | UploadFormSourceDescriptors | Occurs after the standard source inputs have been added to the descriptor. | |
1.28 | UploadStashFile | Occurs before a file is stashed (uploaded to stash). | |
1.6.4 | UploadComplete | Called when a file upload has completed. | |
1.17 | UploadVerifyFile | Called when a file is uploaded, to allow extra file verification to take place | |
1.28 | UploadVerifyUpload | (preferred) Can be used to reject a file upload. Unlike 'UploadVerifyFile' it provides information about upload comment and the file description page, but does not run for uploads to stash. | |
1.23 | ValidateExtendedMetadataCache | Called to validate the cached metadata in FormatMetadata::getExtendedMeta (return false means cache will be invalidated and the GetExtendedMetadata hook will called again).
| |
1.18 | XMPGetInfo | Called when obtaining the list of XMP tags to extract. | |
1.18 | XMPGetResults | Called just before returning the results array of parsing xmp data. | |
Special pages | 1.32 | AncientPagesQuery | Allows modifying the query used by Special:AncientPages. |
1.9.1 | BookInformation | Hook to allow extensions to insert additional HTML to a list of book sources e.g. for API-interacting plugins and so on. | |
1.23 | ChangesListInitRows | Batch process change list rows prior to rendering. | |
1.12 | ChangesListInsertArticleLink | Override or augment link to article in RC list. | |
1.23 | ChangesListSpecialPageFilters | Called after building form options on pages inheriting from ChangesListSpecialPage (in core: RecentChanges, RecentChangesLinked and Watchlist). | |
1.24 | ChangesListSpecialPageQuery | Called when building SQL query on pages inheriting from ChangesListSpecialPage (in core: RecentChanges, RecentChangesLinked and Watchlist). | |
1.29 | ChangesListSpecialPageStructuredFilters | Called to allow extensions to register filters for pages inheriting from ChangesListSpecialPage (in core: RecentChanges, RecentChangesLinked, and Watchlist). | |
1.13 | ContribsPager::getQueryInfo | Called before the contributions query is about to run. | |
1.20 | ContribsPager::reallyDoQuery | Called before really executing the query for Special:Contributions. | |
1.13 | ContributionsLineEnding | Called before an HTML line for Special:Contributions is finished. | |
1.11 | ContributionsToolLinks | Change tool links above Special:Contributions. | |
1.24 | DeletedContribsPager::reallyDoQuery | Called before really executing the query for Special:DeletedContributions. | |
1.24 | DeletedContributionsLineEnding | Called before an HTML line for Special:DeletedContributions is finished. | |
1.17 | EmailUserCC | Occurs before sending the copy of the email to the author. | |
1.17 | EmailUserForm | Occurs after building the email user form object. | |
1.16 | EmailUserPermissionsErrors | Retrieve permissions errors for emailing a user. | |
1.25 | EnhancedChangesList::getLogText | Allows altering, removing or adding to the links of a group of changes in EnhancedChangesList. | |
1.26 | EnhancedChangesListModifyBlockLineData | Modify data used to build a non-grouped entry in Special:RecentChanges. | |
1.26 | EnhancedChangesListModifyLineData | Modify data used to build a grouped recent change inner line in Special:RecentChanges. | |
1.7 | FetchChangesList | Allows extension to modify a recent changes list for a user. | |
1.20 | GitViewers | Called when generating the list of git viewers for Special:Version, use this to change the list. | |
1.30 | NewPagesLineEnding | Called before an HTML line for Special:NewPages is finished. | |
1.23 | LonelyPagesQuery | Allows modifying the query used by Special:LonelyPages. | |
1.14 | OldChangesListRecentChangesLine | Customise entire Recent Changes line. | |
1.23 | PreferencesFormPreSave | Allows last minute changes to a user's preferences (via User#setOption ) before they're saved and gives a possibility to check which options were modified.
| |
1.40 | PreferencesGetIcon | Add icons that will be displayed in the mobile layout of Special:Preferences. | |
1.40 | PreferencesGetLayout | Indicate whether the preferences will have a mobile or desktop layout. | |
1.19 | PreferencesGetLegend | Override the text used for the <legend> of a preferences section.
| |
1.26 | RandomPageQuery | Modify the query used by Special:Random. | |
1.20 | RedirectSpecialArticleRedirectParams | Lets you alter the set of parameter names such as "oldid" that are preserved when using redirecting special pages such as Special:MyPage and Special:MyTalk. | |
1.27 | ShortPagesQuery | Allow extensions to modify the query used by Special:ShortPages. | |
1.24.0 | SpecialBlockModifyFormFields | Add or modify block fields of Special:Block. | |
1.27 | SpecialContributions::getForm::filters | Called with a list of filters to render on Special:Contributions. | |
1.28.0 | SpecialContributions::formatRow::flags | Called before rendering a Special:Contributions row. | |
1.5.0 | SpecialContributionsBeforeMainOutput | Before the form on Special:Contributions | |
1.40 | SpecialCreateAccountBenefits | Replace the default signup page content about the benefits of registering an account ("Wikipedia is made by people like you...") on Special:CreateAccount. | |
1.38 | SpecialExportGetExtraPages | Add extra pages to the list of pages to export. | |
1.13.0 | SpecialListusersDefaultQuery | Called right before the end of UsersPager::getDefaultQuery() .
| |
1.13.0 | SpecialListusersFormatRow | Called right before the end of UsersPager::formatRow() .
| |
1.13.0 | SpecialListusersHeaderForm | Called before adding the submit button in UsersPager::getPageHeader() .
| |
1.13.0 | SpecialListusersHeader | Called before closing the <fieldset> in UsersPager::getPageHeader()
| |
1.13.0 | SpecialListusersQueryInfo | Called right before the end of UsersPager::getQueryInfo()
| |
1.34 | SpecialMuteModifyFormFields | Allows modifying HTMLForm fields for Special:Mute | |
1.34 | SpecialMuteSubmit | (deprecated in 1.34) Used only for instrumentation on SpecialMute | |
1.17 | SpecialNewpagesConditions | Called when building the SQL query for Special:NewPages. | |
1.18 | SpecialNewPagesFilters | Called after building form options at Special:NewPages. | |
1.7.0 | SpecialPage_initList | Called after the Special Page list is populated | |
1.20 | SpecialPageAfterExecute | Called after SpecialPage::execute() .
| |
1.20 | SpecialPageBeforeExecute | Called before SpecialPage::execute() .
| |
1.24 | SpecialPageBeforeFormDisplay | Before executing the HTMLForm object | |
1.18 | SpecialPasswordResetOnSubmit | Called when executing a form submission on Special:PasswordReset. | |
1.16 | SpecialRandomGetRandomTitle | Modify the selection criteria for Special:Random | |
1.13 | SpecialRecentChangesPanel | Called when building form options in SpecialRecentChanges. | |
1.22 | SpecialResetTokensTokens | Called when building token list for SpecialResetTokens. | |
1.27 | SpecialSearchGoResult | Called before the 'go' feature of SpecialSearch redirects a user. May provide it's own url to redirect to. | |
1.18 | SpecialSearchProfileForm | Allows modification of search profile forms | |
1.16 | SpecialSearchProfiles | Allows modification of search profiles. | |
1.21 | SpecialSearchResultsAppend | Called after all search results HTML has been output. Note that in some cases, this hook will not be called (no results, too many results, SpecialSearchResultsPrepend returned false, etc). | |
1.21 | SpecialSearchResultsPrepend | SpecialSearchResultsPrepend: Called immediately before returning HTML on the search results page. Useful for including an external search provider. To disable the output of MediaWiki search output, return false. | |
1.13 | SpecialSearchResults | Called before search result display when there are matches | |
1.18 | SpecialSearchSetupEngine | Allows passing custom data to search engine | |
1.16 | SpecialStatsAddExtra | Can be used to add extra statistic at the end of Special:Statistics. | |
1.29 | SpecialTrackingCategories::generateCatLink | Called for each category link on Special:TrackingCategories | |
1.29 | SpecialTrackingCategories::preprocess | Called after LinkBatch on Special:TrackingCategories | |
1.16 | SpecialUploadComplete | Called after successfully uploading a file from Special:Upload | |
1.21 | SpecialVersionVersionUrl | Called when building the URL for Special:Version. | |
1.22 | SpecialWatchlistGetNonRevisionTypes | Allows extensions to register the value they have inserted to rc_type field of recentchanges for non-revision changes so they can be included in the watchlist. | |
1.26 | SpecialWhatLinksHereLinks | Called every time a list of links is built for a list item for Special:WhatLinksHere. | |
1.18 | UndeleteForm::showHistory | Called in UndeleteForm::showHistory , after a PageArchive object has been created but before any further processing is done.
| |
1.18 | UndeleteForm::showRevision | Called in UndeleteForm::showRevision , after a PageArchive object has been created but before any further processing is done.
| |
1.18 | UndeleteForm::undelete | Called in UndeleteForm::undelete , after checking that the site is not in read-only mode, that the Title object is not null and after a PageArchive object has been constructed but before performing any further processing.
| |
1.9 | UndeleteShowRevision | (deprecated in 1.35) Called when showing a revision in Special:Undelete. | |
1.9.0 | UploadForm:initial | Called just before the upload form is generated | |
1.28 | UsersPagerDoBatchLookups | Give extensions providing user group data from an alternate source a chance to add their data into the cache array so that things like global user groups are displayed correctly in Special:ListUsers. | |
1.18 | WantedPages::getQueryInfo | Called in WantedPagesPage::getQueryInfo() , can be used to alter the SQL query which gets the list of wanted pages.
| |
1.24 | WatchlistEditorBeforeFormRender | Occurs before building the Special:EditWatchlist form, used to manipulate the list of pages or preload data based on that list. | |
1.17 | WatchlistEditorBuildRemoveLine | Occurs when building remove lines in Special:Watchlist/edit. | |
1.24.0 | WhatLinksHereProps | Allows extensions to annotate WhatLinksHere entries. | |
1.40 | ContributeCards | Fired on Special:Contribute page to allow extensions to add "card" entry points. | |
User Management | 1.13 | AbortAutoblock | Allow extension to cancel an autoblock. |
1.20 | AbortEmailNotification | Can be used to cancel email notifications for an edit. | |
1.22 | AbortTalkPageEmailNotification | Disable email notifications of edits to users' talk pages. | |
1.5.0 | AddNewAccount | (deprecated in 1.27) Called after a user account is created | |
1.27 | AuthManagerLoginAuthenticateAudit | A login attempt either succeeded or failed for a reason other than misconfiguration or session loss. No return data is accepted; this hook is for auditing only. | |
1.13 | AuthPluginAutoCreate | (deprecated in 1.27) Called when creating a local account for an user logged in from an external authentication method. | |
1.9.1 | AuthPluginSetup | (deprecated in 1.27) Update or replace authentication plugin object ($wgAuth). | |
1.12 | AutopromoteCondition | Check autopromote condition for user. | |
1.32 | BeforeResetNotificationTimestamp | Allows prevention of clearing of notification timestamp when a user views a page in their watchlist. | |
1.4.0 | BlockIpComplete | Occurs after the request to block (or change block settings of) an IP or user has been processed | |
1.4.0 | BlockIp | Occurs whenever the software receives a request to block (or change the block settings of) an IP address or user | |
1.27 | ChangeAuthenticationDataAudit | Called when user changes authentication data. | |
1.29.0 | ChangeUserGroups | Called before a user's group memberships are changed | |
1.16 | ConfirmEmailComplete | Called after a user's email has been confirmed successfully. | |
1.31 | DeleteUnknownPreferences | Called by the cleanupPreferences.php maintenance script to build a WHERE clause with which to delete preferences that are not known about. | |
1.7 | EmailConfirmed | Replace default way to check whether user's email is confirmed. | |
1.19 | ExemptFromAccountCreationThrottle | To add an exemption from the account creation throttle. | |
1.37 | GetAllBlockActions | Add an action that can be blocked via a partial block. | |
1.40 | GetBlockErrorMessageKey | Allows extensions to override the message that will be displayed to the user. | |
1.6.0 | GetBlockedStatus | (removed in 1.35) Fired after the user's getBlockStatus is set | |
1.34 | GetUserBlock | Modify the block found by the block manager. | |
1.12 | getUserPermissionsErrors | Add a permissions error when permissions errors are checked for. | |
1.12 | getUserPermissionsErrorsExpensive | Same as getUserPermissionsErrors as but called only if expensive checks are enabled. | |
1.13 | GetAutoPromoteGroups | When determining which autopromote groups a user is entitled to be in. | |
1.16 | InvalidateEmailComplete | Called after a user's email has been invalidated successfully. | |
1.9 | IsTrustedProxy | Allows an extension to set an IP as trusted or not. | |
1.12 | isValidEmailAddr | Override the result of Sanitizer::validateEmail() .
| |
1.11 | isValidPassword | Override the result of User::isValidPassword() .
| |
1.26.0 | LocalUserCreated | Called immediately after a local user has been created and saved to the database. | |
1.37 | LoadUserOptions | This hook is called when user options/preferences are being loaded from the database. | |
1.26 | PasswordPoliciesForUser | Alter the effective password policy for a user. | |
1.18 | PerformRetroactiveAutoblock | Called before a retroactive autoblock is applied to a user. | |
1.39 | PermissionErrorAudit | Called after permission checks to allow logging. | |
1.9 | PingLimiter | Allows extensions to override the results of User::pingLimiter() .
| |
1.11 | PrefsEmailAudit | Called when user changes his email address. | |
1.23 | ResetPasswordExpiration | Allow extensions to set a default password expiration. | |
1.40 | RenameUserAbort | Allow other extensions to abort a user rename | |
1.40 | RenameUserComplete | After a sucessful user rename | |
1.40 | RenameUserPreRename | Before renaming an user | |
1.40 | RenameUserWarning | Get warnings when renaming an user | |
1.37 | SaveUserOptions | Called just before saving user preferences. This hook replaces UserSaveOptions . Hook handlers can either add or manipulate options, or reset one back to its default to block changing it. Hook handlers are also allowed to abort the process by returning false , e.g. to save to a global profile instead. Compare to the UserSaveSettings hook, which is called after the preferences have been saved.
| |
1.27.0 | SecuritySensitiveOperationStatus | Affect the return value from AuthManager::securitySensitiveOperationStatus()
| |
1.27.0 | SessionCheckInfo | Validate session info as it's being loaded from storage | |
1.27.0 | SessionMetadata | Add metadata to a session being saved | |
1.39 | TempUserCreatedRedirect | This hook is called after an action causes a temporary user to be created. The handler may modify the redirect URL. | |
1.21 | UpdateUserMailerFormattedPageStatus | Occurs before a notification email gets sent. | |
1.29.0 | UnblockUserComplete | Occurs after the request to unblock an IP or user has been processed | |
1.29.0 | UnblockUser | Occurs whenever the software receives a request to unblock an IP address or user | |
1.14 | User::mailPasswordInternal | Before creation and mailing of a user's new temporary password. | |
1.18 | UserAddGroup | Called when adding a group to an user. | |
1.13 | UserArrayFromResult | Called when creating an UserArray object from a database result. | |
1.6.0 | userCan | To interrupt/advise the "user can do X to Y article" check | |
1.12 | UserCanSendEmail | Allows overriding the permission check in User::canSendEmail() .
| |
1.38 | UserEditCountUpdate | This is called from a deferred update on edit or move and provides collected user edit count information. | |
1.11 | UserEffectiveGroups | Dynamically add or remove to the default user groups provided by the database table user_groups . | |
1.13 | UserGetAllRights | After calculating a list of all available rights. | |
1.18 | UserGetDefaultOptions | Called after fetching the core default user options. | |
1.13 | UserGetEmailAuthenticationTimestamp | Called when getting the timestamp of email authentification. | |
1.13 | UserGetEmail | Called when getting an user email address. | |
1.18 | UserGetLanguageObject | Called when getting user's interface language object. | |
1.14 | UserGetReservedNames | Allows to modify $wgReservedUsernames at run time. | |
1.32.0 | UserGetRightsRemove | Called in User::getRights() to dynamically remove rights
| |
1.11.0 | UserGetRights | Called in User::getRights() to dynamically add rights
| |
1.26 | UserGroupsChanged | Called after user groups are changed. | |
1.16 | UserIsBlockedFrom | Check if a user is blocked from a specific page (for specific block exemptions). | |
1.14 | UserIsBlockedGlobally | (deprecated in 1.40) Runs before User::mBlockedGlobally is set; can be used to change the blocked status of an IP address or a user
| |
1.28 | UserIsBot | Occurs when determining whether a user is a bot account. | |
1.26.0 | UserIsLocked | Fired to check if a user account is locked | |
1.22 | UserIsEveryoneAllowed | Check if all users are allowed some user right; return false if a UserGetRights hook might remove the named right.
| |
1.26 | UserIsHidden | (removed in 1.35) Check if the user's name should be hidden. See User::isHidden() .
| |
1.14 | UserLoadAfterLoadFromSession | Called to authenticate users on external/environmental means; occurs after session is loaded. | |
1.13 | UserLoadDefaults | Called when loading a default user. | |
1.15 | UserLoadFromDatabase | Called when loading a user from the database. | |
1.16 | UserLoadOptions | When user options/preferences are being loaded from the database. | |
1.27.0 | UserLoggedIn | Called after a user is logged in | |
1.27 | UserMailerSplitTo | Called in UserMailer::send() to give extensions a chance to split up an email with multiple of the To: field into separate emails.
| |
1.27 | UserMailerTransformContent | Allow transformation of content, such as encrypting/signing. | |
1.27 | UserMailerTransformMessage | Called in UserMailer::send() to change email after it has gone through the MIME transform. Extensions can block sending the email by returning false and setting $error.
| |
1.41 | UserPrivilegedGroups | Use this hook to extend what MediaWiki considers to be a "privileged group" beyond the values set in $wgPrivilegedGroups | |
1.18 | UserRemoveGroup | Called when removing a group from an user. | |
1.22 | UserRequiresHTTPS | (deprecated in 1.35) Allows extensions to override whether users need to be redirected to HTTPS. | |
1.24 | UserResetAllOptions | Allows changing the behaviour when a user's preferences are reset. For instance, certain preferences can be preserved. | |
1.16 | UserSaveOptions | Called just before saving user preferences. Hook handlers can either add or manipulate options, or reset one back to its default to block changing it. Hook handlers are also allowed to abort the process by returning false , e.g. to save to a global profile instead. Compare to the UserSaveSettings hook, which is called after the preferences have been saved.
| |
1.13 | UserSaveSettings | Called directly after user preferences (user_properties in the database) have been saved. Compare to the UserSaveOptions hook, which is called before. | |
1.33 | UserSendConfirmationMail | Called just before a confirmation email is sent to a user. Hook handlers can modify the email that will be sent. | |
1.13 | UserSetCookies | (deprecated in 1.27) Called when setting user cookies. | |
1.13 | UserSetEmailAuthenticationTimestamp | Called when setting the timestamp of email authentication. | |
1.13 | UserSetEmail | Called when changing user email address. | |
Logging | 1.23 | GetLogTypesOnUser | Add log types where the target is a userpage. |
1.26.0 | LogException | Called before an exception (or PHP error) is logged. | |
1.25 | LogEventsListGetExtraInputs | When getting extra inputs to display on Special:Log for a specific log type. | |
1.30 | LogEventsListLineEnding | Called before an HTML line for Special:Log is finished. | |
1.19 | LogEventsListShowLogExtract | Called before the result of LogEventsList::showLogExtract() is added to OutputPage.
| |
1.12 | LogLine | Processes a single log entry on Special:Log. | |
1.33 | ManualLogEntryBeforePublish | Lets extensions tag log entries when actions are performed. | |
1.29 | OtherAutoblockLogLink | Get links to the autoblock log from extensions which autoblocks users and/or IP addresses too.. | |
1.16 | OtherBlockLogLink | Get links to the block log from extensions which blocks users and/or IP addresses too. | |
1.24 | SpecialLogAddLogSearchRelations | Add log relations to the current log. | |
Skinning / Templates | 1.27.0 | AuthChangeFormFields | Allows modification of AuthManager-based forms |
1.23.0 | BaseTemplateAfterPortlet | (deprecated in 1.35) (SkinTemplate.php) After rendering of portlets. | |
1.18 | BaseTemplateToolbox | (deprecated in 1.35) Called by BaseTemplate when building the toolbox array and returning it for the skin to output. | |
1.7.0 | BeforePageDisplay | Allows last minute changes to the output page, e.g. adding of CSS or JavaScript by extensions. | |
1.25.0 | LoginFormValidErrorMessages | Allows to add additional error messages (SpecialUserLogin.php). | |
1.7.0 | PersonalUrls | (SkinTemplate.php) Called after the list of personal URLs (links at the top in Monobook) has been populated. | |
1.24.0 | PostLoginRedirect | (SpecialUserlogin.php) Modify the post login redirect behaviour. | |
1.19 | RequestContextCreateSkin | Called when creating a skin instance. | |
1.35 | SkinAddFooterLinks | Add items to the footer for skins using SkinAddFooterLinks. | |
1.11.0 | SkinAfterBottomScripts | (Skin.php) At the end of Skin::bottomScripts()
| |
1.14 | SkinAfterContent | Allows extensions to add text after the page content and article metadata. | |
1.35 | SkinAfterPortlet | Occurs whenever a page is rendered and allows to add HTML after portlets have been put out. | |
1.14 | SkinBuildSidebar | At the end of Skin::buildSidebar() .
| |
1.16 | SkinCopyrightFooter | Allow for site and per-namespace customisation of copyright notice. | |
1.25 | SkinEditSectionLinks | Modify the section edit links. Called when section headers are created. | |
1.17 | SkinGetPoweredBy | (deprecated in 1.37) Called when generating the code used to display the "Powered by MediaWiki" icon. | |
1.36 | SkinPageReadyConfig | Allows skins to change the `mediawiki.page.ready` module configuration. | |
1.24 | SkinPreloadExistence | Modify the CSS class of an array of page links. | |
1.12.0 | SkinSubPageSubtitle | (Skin.php) Called before the list of subpage links on top of a subpage is generated | |
1.6.0 | SkinTemplateBuildNavUrlsNav_urlsAfterPermalink | (deprecated in 1.35) Called after the permalink has been entered in navigation URL array. | |
1.23.0 | SkinTemplateGetLanguageLink | Called after building the data for a language link from which the actual html is constructed. | |
1.18.0 | SkinTemplateNavigation::SpecialPage | (usage discouraged)
Called on special pages after the special tab is added but before variants have been added | |
1.18.0 | SkinTemplateNavigation::Universal | (usage discouraged)
Called on both content and special pages after variants have been added | |
1.16.0 | SkinTemplateNavigation | Called on content pages only after tabs have been added, but before variants have been added. See the other two SkinTemplateNavigation hooks for other points tabs can be modified at. | |
1.10 | SkinTemplateOutputPageBeforeExec | (deprecated in 1.35) Allows further setup of the template engine after all standard setup has been performed but before the skin has been rendered. | |
1.6.0 | SkinTemplatePreventOtherActiveTabs | (deprecated in 1.35) Called to enable/disable the inclusion of additional tabs to the skin. | |
1.12 | SkinTemplateTabAction | (deprecated in 1.35) Override SkinTemplate::tabAction() .
| |
1.13 | SkinTemplateToolboxEnd | (deprecated in 1.35) Called by SkinTemplate skins after toolbox links have been rendered (useful for adding more). | |
API | 1.14.0 | APIAfterExecute | Use this hook to extend core API modules |
1.23.0 | ApiBeforeMain | Called before ApiMain is executed | |
1.20 | ApiCheckCanExecute | Called during ApiMain::checkCanExecute() .
| |
1.29 | ApiDeprecationHelp | Add messages to the 'deprecation-help' warning generated from ApiBase::addDeprecation() .
| |
1.25 | ApiFormatHighlight | Use to syntax-highlight API pretty-printed output. When highlighting, add output to $context->getOutput() and return false .
| |
1.14.0 | APIGetAllowedParams | Use this hook to modify a module's parameters | |
1.25 | APIGetDescriptionMessages | Allows to modify a module's help message. | |
1.25 | APIGetParamDescriptionMessages | Allows to modify a module's parameter descriptions. | |
1.25 | APIHelpModifyOutput | Allows to modify an API module's help output. | |
1.25 | ApiMain::moduleManager | Can be used to conditionally register API modules. | |
1.20 | ApiMain::onException | Called by ApiMain::executeActionWithErrorHandling() when an exception is thrown during API action execution.
| |
1.28 | ApiMakeParserOptions | Allows extensions to adjust the parser options before parsing. | |
1.32 | ApiMaxLagInfo | Called right before giving out information about max lag in API. | |
1.25.0 | ApiOpenSearchSuggest | Called when constructing the OpenSearch results. Hooks can alter or append to the array. | |
1.33 | ApiOptions | Called by action=options before applying changes to user preferences. | |
1.32 | ApiParseMakeOutputPage | Called when preparing the OutputPage object for ApiParse. This is mainly intended for calling OutputPage::addContentOverride() or OutputPage::addContentOverrideCallback() .
| |
1.25 | ApiQuery::moduleManager | Called when ApiQuery has finished initialising its module manager. | |
1.14.0 | APIQueryAfterExecute | Use this hook to extend core API query modules | |
1.28 | ApiQueryBaseAfterQuery | Called for (some) API query modules after the database query has returned. | |
1.28 | ApiQueryBaseBeforeQuery | Called for (some) API query modules before a database query is made. | |
1.28 | ApiQueryBaseProcessRow | Called for (some) API query modules as each row of the database result is processed. | |
1.14.0 | APIQueryGeneratorAfterExecute | Use this hook to extend core API query modules | |
1.13.0 | APIQueryInfoTokens | (removed in 1.36) Use this hook to add custom tokens to prop=info | |
1.14.0 | APIQueryRecentChangesTokens | (removed in 1.36) Use this hook to add custom tokens to list=recentchanges | |
1.13.0 | APIQueryRevisionsTokens | (removed in 1.36) Use this hook to add custom tokens to prop=revisions | |
1.18 | APIQuerySiteInfoGeneralInfo | Used to add extra information to the SiteInfo general information output. | |
1.22 | APIQuerySiteInfoStatisticsInfo | Used to add extra information to the SiteInfo statistics information output. | |
1.24 | ApiQueryTokensRegisterTypes | Use this hook to add additional token types to action=query&meta=tokens. Note that most modules will probably be able to use the 'csrf' token instead of creating their own token types. | |
1.15 | APIQueryUsersTokens | (removed in 1.36) Use this hook to add custom token to list=users. | |
1.29 | ApiQueryWatchlistExtractOutputData | Extract row data for ApiQueryWatchlist. | |
1.29 | ApiQueryWatchlistPrepareWatchedItemQueryServiceOptions | Populate the options to be passed from ApiQueryWatchlist to WatchedItemQueryService. | |
1.17 | ApiRsdServiceApis | Add or remove APIs from the RSD services list. | |
1.20 | ApiTokensGetTokenTypes | (removed in 1.36) Use this hook to extend action=tokens with new token types. | |
1.29 | ApiValidatePassword | This will allow for checking passwords against the wiki's password. | |
1.23.0 | AddNewAccountApiForm | (deprecated in 1.27) Allows modifying the internal login form when creating an account via the API. | |
1.23.0 | AddNewAccountApiResult | (deprecated in 1.27) Modifies the API output when an account is created via the API. | |
Import/Export | 1.17.0 | AfterImportPage | When a page import is completed |
1.17.0 | ImportHandleLogItemXMLTag | When parsing a XML tag in a log item | |
1.17.0 | ImportHandlePageXMLTag | When parsing a XML tag in a page | |
1.17.0 | ImportHandleRevisionXMLTag | When parsing a XML tag in a page revision | |
1.17.0 | ImportHandleToplevelXMLTag | When parsing a top level XML tag | |
1.31 | ImportHandleUnknownUser | When a user doesn't exist locally, this hook is called to give extensions an opportunity to auto-create it. If the auto-creation is successful, return false .
| |
1.17.0 | ImportHandleUploadXMLTag | When parsing a XML tag in a file upload | |
1.27 | ImportLogInterwikiLink | Hook to change the interwiki link used in log entries and edit summaries for transwiki imports. | |
1.27 | ImportSources | Called when reading from the $wgImportSources configuration variable.
| |
1.16.0 | ModifyExportQuery | Modify the query used by the exporter. | |
1.15.0 | WikiExporter::dumpStableQuery | Get the SELECT query for "stable" revisions dumps | |
1.16.0 | XmlDumpWriterOpenPage | Called at the end of XmlDumpWriter::openPage , to allow extra metadata to be added.
| |
1.16.0 | XmlDumpWriterWriteRevision | Called at the end of a revision in an XML dump, to add extra metadata. | |
Diffs | 1.14 | AbortDiffCache | Can be used to cancel the caching of a diff |
1.17 | ArticleContentOnDiff | Before showing the article content below a diff. | |
1.29 | DifferenceEngineAfterLoadNewText | Called in DifferenceEngine::loadNewText() after the new revision's content has been loaded into the class member variable.
| |
1.29 | DifferenceEngineLoadTextAfterNewContentIsLoaded | Called in DifferenceEngine::loadText() after the new revision's content has been loaded into the class member variable $differenceEngine->mNewContent but before checking if the variable's value is null .
| |
1.29 | DifferenceEngineMarkPatrolledLink | Allow extensions to change the markpatrolled link, which is shown both on the diff header as well as on the bottom of a page, usually wrapped in a <span> element which has class="patrollink" .
| |
1.29 | DifferenceEngineMarkPatrolledRCID | Allows extensions to possibly change the rcid parameter. | |
1.29 | DifferenceEngineNewHeader | Allows extensions to change the $newHeader variable, which contains information about the new revision, such as the revision's author, whether. | |
1.29 | DifferenceEngineOldHeaderNoOldRev | Change the $oldHeader variable in cases when there is no old revision. | |
1.29 | DifferenceEngineOldHeader | Allows extensions to change the $oldHeader variable, which contains information about the old revision, such as the revision's author, whether the revision was marked as a minor edit or not, etc. | |
1.29 | DifferenceEngineRenderRevisionAddParserOutput | Allows extensions to change the parser output. Return false to not add parser output via OutputPage's addParserOutput method.
| |
1.29 | DifferenceEngineRenderRevisionShowFinalPatrolLink | An extension can hook into this hook point and return false to not show the final "mark as patrolled" link on the bottom of a page.
| |
1.29 | DifferenceEngineShowDiffPageMaybeShowMissingRevision | Called in DifferenceEngine::showDiffPage() when revision data cannot be loaded.
| |
1.29 | DifferenceEngineShowDiffPage | Add additional output via the available OutputPage object into the diff view. | |
1.29 | DifferenceEngineShowDiff | Allows extensions to affect the diff text which eventually gets sent to the OutputPage object. | |
1.29 | DifferenceEngineShowEmptyOldContent | Allows extensions to change the diff table body (without header) in cases when there is no old revision or the old and new revisions are identical. | |
1.35 | DifferenceEngineViewHeader | Called before displaying a diff. | |
1.21 | DiffRevisionTools | (deprecated in 1.35) Override or extend the revision tools available from the diff view, i.e. undo, etc. | |
1.35 | DiffTools | Use this hook to override or extend the revision tools available from the diff view, i.e. undo, etc. | |
1.7 | DiffViewHeader | (deprecated in 1.35) Called before diff display. | |
1.25.0 | GetDifferenceEngine | Allows custom difference engine extensions such as Extension:WikEdDiff . | |
1.21 | EditPageGetDiffContent | Allow modifying the wikitext that will be used in "Show changes". Note that it is preferable to implement diff handling for different data types using the ContentHandler facility. | |
1.15 | NewDifferenceEngine | Called when a new DifferenceEngine object is made. | |
1.32 | GetSlotDiffRenderer | Replace or wrap the standard SlotDiffRenderer for some content type. | |
1.41 | TextSlotDiffRendererTablePrefix | Allows to change the HTML that is included in a prefix container directly before the diff table | |
Miscellaneous | 1.19.0 | AlternateUserMailer | Called before mail is sent so that mail could be logged (or something else) instead of using PEAR or PHP's mail() .
|
1.6.0 | ArticleEditUpdatesDeleteFromRecentchanges | (deprecated in 1.35) Occurs before saving to the database. | |
1.19 | BacklinkCacheGetConditions | Allows to set conditions for query when links to certain title. | |
1.19 | BacklinkCacheGetPrefix | Allows to set prefix for a spefific link table. | |
1.16 | BeforeInitialize | Occurs before anything is initialised in MediaWiki::performRequest() .
| |
1.36 | BeforeRevertedTagUpdate | This hook is called before scheduling a RevertedTagUpdateJob. | |
1.21.0 | CategoryAfterPageAdded | Called after a page is added to a category | |
1.21.0 | CategoryAfterPageRemoved | Called after a page is removed from a category | |
1.19.0 | Collation::factory | Allows extensions to register new collation names, to be used with $wgCategoryCollation | |
1.8.0 | DisplayOldSubtitle | Allows extensions to modify the displaying of links to other revisions when browsing through revisions. | |
1.17 | ExtensionTypes | Called when generating the extensions credits, use this to change the tables headers. | |
1.37 | GetActionName | Use this hook to override the action name depending on request parameters. | |
1.13 | GetCacheVaryCookies | Get cookies that should vary cache options. | |
1.18 | GetCanonicalURL | Allows to modify fully-qualified URLs used for IRC and e-mail notifications. | |
1.18.0 | GetDefaultSortkey | Allows to override what the default sortkey is, which is used to order pages in a category. | |
1.21.0 | GetDoubleUnderscoreIDs | Hook for modifying the list of magic words | |
1.6.0 | GetFullURL | Used to modify fully-qualified URLs used in redirects/export/offsite data | |
1.6.0 | GetInternalURL | Used to modify fully-qualified URLs (useful for squid cache purging) | |
1.17 | GetIP | Modify the ip of the current user (called only once). | |
1.6.0 | GetLocalURL | Used to modify local URLs as output into page links | |
1.19 | GetLocalURL::Article | Allows to modify local URLs specifically pointing to article paths without any fancy queries or variants. | |
1.19 | GetLocalURL::Internal | Allows to modify local URLs to internal pages. | |
1.35 | GetMagicVariableIDs | Use this hook to modify the list of magic variables. | |
1.35 | HtmlCacheUpdaterAppendUrls | This hook is used to declare extra URLs to purge from HTTP caches. | |
1.35 | HtmlCacheUpdaterVaryUrls | This hook is used to add variants of URLs to purge from HTTP caches. | |
1.20 | InfoAction | When building information to display on the action=info page.
| |
1.13 | InitializeArticleMaybeRedirect | Called when checking if title is a redirect. | |
1.18 | InterwikiLoadPrefix | (deprecated in 1.36) This hook is called when resolving whether a given prefix is an interwiki or not. | |
1.18 | IRCLineURL | When constructing the URL to use in an IRC notification. | |
1.19 | Language::getMessagesFileName | Use to change the path of a localisation file. | |
1.18 | LanguageGetTranslatedLanguageNames | Provide translated language names. | |
1.22.0 | LanguageLinks | Manipulate a page's language links. | |
1.14.0 | LinkBegin | (removed in 1.36) Used when generating internal and interwiki links in Linker::link()
| |
1.14.0 | LinkEnd | (removed in 1.36) Used when generating internal and interwiki links in Linker::link() , just before the function returns a value.
| |
1.12 | LinksUpdate | At the beginning of LinksUpdate::doUpdate() just before the actual update.
| |
1.21 | LinksUpdateAfterInsert | Occurs right after new links have been inserted into the links table. | |
1.12 | LinksUpdateComplete | At the end of LinksUpdate::doUpdate() when updating has completed.
| |
1.11 | LinksUpdateConstructed | At the end of LinksUpdate() is construction.
| |
1.10.1 | LoadExtensionSchemaUpdates | Fired when MediaWiki is updated to allow extensions to register updates for the database schema. | |
1.24 | LocalisationCacheRecacheFallback | Called for each language when merging fallback data into the cache. | |
1.16 | LocalisationCacheRecache | Called when loading the localisation data into cache. | |
1.23 | LocalisationChecksBlacklist | When fetching the blacklist of localisation checks. | |
1.24 | LocalisationIgnoredOptionalMessages | args = array &$ignoredMessageKeys, array &$optionalMessageKeys | |
1.6.0 | MagicWordwgVariableIDs | (deprecated in 1.35) Tells MediaWiki that one or more magic word IDs should be treated as variables. | |
1.18 | MaintenanceRefreshLinksInit | Before executing the refreshLinks.php maintenance script. | |
1.33 | MaintenanceUpdateAddParams | Allow extensions to add params to the update.php maintenance script. | |
1.23 | MathMLChanged | Is called before the MathML property is changed can be used e.g. for compression, normalisation or introduction of custom hyperlinks etc. | |
1.12.0 | MediaWikiPerformAction | Override MediaWiki::performAction()
| |
1.32 | MediaWikiPHPUnitTest::endTest | Occurs when a MediaWiki PHPUnit test has ended. | |
1.32 | MediaWikiPHPUnitTest::startTest | Occurs when a MediaWiki PHPUnit test has started. | |
1.27.0 | MediaWikiServices | Called when a global MediaWikiServices instance is initialised. | |
1.23 | MessageCache::get | Allows changing a message key, to customise it before the translation is accessed. | |
1.41 | MessageCacheFetchOverrides | Allows changing message keys, to customise it before the translation is accessed | |
1.15 | MessageCacheReplace | When a message page is changed. Useful for updating caches. | |
1.5.7 | MessagesPreLoad | Occurs when loading a message from the database | |
1.24 | MimeMagicGuessFromContent | Allows MW extensions guess the MIME by content. | |
1.24 | MimeMagicImproveFromExtension | Allows MW extensions to further improve the MIME type detected by considering the file extension. | |
1.24 | MimeMagicInit | Before processing the list mapping MIME types to media types and the list mapping MIME types to file extensions. As an extension author, you are encouraged to submit patches to MediaWiki's core to add new MIME types to mime.types. | |
1.13 | OpenSearchUrls | Called when constructing the OpenSearch description XML. Hooks can alter or append to the array of URLs for search & suggestion formats. | |
1.25 | OpportunisticLinksUpdate | Allows performing updates when a page is re-rendered. | |
1.20 | ParserTestGlobals | Allows to define globals for parser tests. | |
1.6.0 | ParserTestParser | Called when creating a new instance of Parser for parser tests | |
1.10 | ParserTestTables | (deprecated in 1.36) Alter the list of tables to duplicate when parser tests are run. Use when page save hooks require the presence of custom tables to ensure that tests continue to run properly. | |
1.12 | PrefixSearchBackend | (deprecated in 1.27) Override the title prefix search used for OpenSearch and AJAX search suggestions. | |
1.25 | PrefixSearchExtractNamespace | Called if core was not able to extract a namespace from the search string so that extensions can attempt it. | |
1.10 | RawPageViewBeforeOutput | Called before displaying a page with action=raw. Returns true if display is allowed, false if display is not allowed.
| |
1.30 | RecentChangesPurgeRows | Called when old recentchanges rows are purged, after deleting those rows but within the same transaction. | |
1.27 | RequestHasSameOriginSecurity | Called to determine if the request is somehow flagged to lack same-origin security. | |
1.8.0 | RecentChange_save | Called after a "Recent Change" is committed to the DB | |
1.16 | SearchableNamespaces | An option to modify which namespaces are searchable. | |
1.21 | SearchAfterNoDirectMatch | If there was no match for the exact result. This runs before lettercase variants are attempted, whereas SearchGetNearMatch runs after. | |
1.28 | SearchDataForIndex | Allows to provide custom content fields when indexing a document. | |
1.40 | SearchDataForIndex2 | Allows to provide custom content fields when indexing a document. | |
1.16 | SearchGetNearMatchBefore | Perform exact-title-matches in "go" searches before the normal operations. | |
1.16 | SearchGetNearMatchComplete | A chance to modify exact-title-matches in "go" searches. | |
1.12 | SearchGetNearMatch | An extra chance for exact-title-matches in "go" searches. | |
1.28 | SearchIndexFields | Add fields to search index mapping. | |
1.20 | SearchResultInitFromTitle | Set the revision used when displaying a page in search results. | |
1.35 | SearchResultProvideDescription | Called when generating search results in order to fill the "description" field in an extension. | |
1.35 | SearchResultProvideThumbnail | This hook is called when generating search results in order to fill the thumbnail field in an extension.
| |
1.28 | SearchResultsAugment | Allows extension to add its code to the list of search result augmentors. | |
1.25 | SecondaryDataUpdates | (deprecated in 1.32) Allows modification of the list of DataUpdates to perform when page content is modified. | |
1.24 | SelfLinkBegin | Called when rendering a self link on a page. | |
1.23 | SendWatchlistEmailNotification | Can be used to cancel watchlist email notifications (enotifwatchlist) for an edit. | |
1.14 | SetupAfterCache | Called in Setup.php, after cache objects are set. | |
1.15 | SoftwareInfo | Called by Special:Version for returning information about the software. | |
1.18 | TestCanonicalRedirect | Called when about to force a redirect to a canonical URL for a title when we have no other parameters on the URL. | |
1.22.0 | TitleSquidURLs | To modify/provide alternate URLs to send HTTP PURGE requests. | |
1.30.0 | UnitTestsAfterDatabaseSetup | Called right after MediaWiki's test infrastructure has finished creating/duplicating core tables for unit tests. | |
1.30.0 | UnitTestsBeforeDatabaseTeardown | Called right before MediaWiki's test infrastructure begins tearing down tables for unit tests. | |
1.17.0 | UnitTestsList | Add tests that should be run as part of the unit test suite. | |
1.24.0 | UserMailerChangeReturnPath | Called to generate a VERP return address when UserMailer sends an email, with a bounce handling extension. | |
1.29 | WatchedItemQueryServiceExtensions | Add a WatchedItemQueryServiceExtension. | |
1.19 | WebRequestPathInfoRouter | While building the PathRouter to parse the REQUEST_URI. | |
1.22 | WebResponseSetCookie | Use to modify the cookie being set from WebResponse::setcookie() .
| |
1.20 | wfShellWikiCmd | Called when generating a shell-escaped command line string to run a cli script. | |
1.6.0 | wgQueryPages | Runs for every Special page that extends the QueryPage class (fired when including the file QueryPage.php). It is only useful in maintenance/updateSpecialPages.php and in QueryPage Api. |
Alphabetical list of hooks
For a complete list of hooks, use the category , which should be kept more up to date.
See also
$wgHooks
- Category:Hook extensions
- Manual:Tag extensions
- Manual:Parser functions
- Hooks.md — specification of the hooks system
- List of hook interfaces in MediaWiki Core
- Extension:Examples — contains examples of hooks
- mw.hook — the JavaScript/front-end system of hooks