Extension:Scribunto/Lua reference manual
This manual documents Lua as it is used in MediaWiki with the Scribunto extension. Some parts are derived from the Lua 5.1 reference manual, which is available under the MIT license.
![]() | This page documents the latest version of the Scribunto extension. Some features may not be deployed yet. |
Introduction
Getting started
On a MediaWiki wiki with Scribunto enabled, create a page with a title starting with "Module:", for example "Module:Bananas". Into this new page, copy the following text:
local p = {} --p stands for package
function p.hello( frame )
return "Hello, world!"
end
return p
Save that, then on another (non-module) page, as on a sandbox page, write:
{{#invoke:Bananas|hello}}
Except that you should replace "Bananas" with whatever you called your module. This will call the "hello" function exported from that module. The {{#invoke:Bananas|hello}}
will be replaced with the text that the function returned, in this case, "Hello, world!"
It's generally a good idea to invoke Lua code from the context of a template. This means that from the perspective of a calling page, the syntax is independent of whether the template logic is implemented in Lua or in wikitext. It also avoids the introduction of additional complex syntax into the content namespace of a wiki.
Module structure
The module itself must return a Lua table containing the functions that may be called by {{#invoke:}}
.
Generally, as shown above, a local variable is declared holding a table, functions are added to this table, and the table is returned at the end of the module code.
Any functions that are not added to this table, whether local or global, will not be accessible by {{#invoke:}}
, but globals might be accessible from other modules loaded using require()
.
It is generally good style for the module to declare all functions and variables local.
Accessing parameters from wikitext
Functions called by {{#invoke:}}
will be passed a single parameter, that being a frame object. To access the parameters passed to the {{#invoke:}}
, code will typically use the args
table of that frame object. It's also possible to access the parameters passed to the template containing the {{#invoke:}}
by using frame:getParent()
and accessing that frame's args
.
This frame object is also used to access context-specific features of the wikitext parser, such as calling parser functions, expanding templates, and expanding arbitrary wikitext strings.
Returning text
The module function should usually return a single string; whatever values are returned will be passed through tostring() and then concatenated with no separator. This string is incorporated into the wikitext as the result of the {{#invoke:}}
.
At this point in the page parse, templates have already been expanded, parser functions and extension tags have already been processed, and pre-save transforms (i.e., signature tilde expansion, pipe trick, etc.) have already happened. Therefore the module cannot use these features in its output text. For example, if a module returns "Hello, [[world]]! {{welcome}}"
, the page will read "Hello, world! {{welcome}}".
On the other hand, subst is handled at an earlier stage of processing, so with {{subst:#invoke:}}
only other attempted substitutions will be processed. Since the failed substitution will remain in the wikitext, they will then be processed on the next edit. This should generally be avoided.
Module documentation
Scribunto allows modules to be documented by automatically associating the module with a wikitext documentation page; by default, the "/doc" subpage of the module is used for this purpose and is transcluded above the module source code on the module page. For example, the documentation for "Module:Bananas" would be at "Module:Bananas/doc".
This can be configured using the following Help:System message :
scribunto-doc-page-name
â Sets the name of the page used for documentation. The name of the module (without the Module: prefix) is passed as$1
. If in the module namespace, the pages specified here will be interpreted as wikitext rather than Lua source and may not be used with{{#invoke:}}
. The default is "Module:$1/doc", i.e. the /doc subpage of the module. Note that parser functions and other brace expansion may not be used in this message.scribunto-doc-page-does-not-exist
â Message displayed when the doc page does not exist. The name of the page is passed as$1
. The default is empty.scribunto-doc-page-show
â Message displayed when the doc page does exist. The name of the page is passed as$1
. The default is to transclude the documentation page.scribunto-doc-page-header
â Header displayed when viewing the documentation page itself. The name of the module (with Module: prefix) being documented is passed as$1
. The default simply displays a short explanation in italics.
Note that modules cannot be directly categorized and cannot have interwiki links directly added.
These could be placed on the documentation page inside <includeonly>...</includeonly>
tags, where they will be applied to the module when the documentation page is transcluded onto the module page.
Renaming or moving modules
To rename or move a module, use the Move Page link in the Tools sidebar. You will want to move both the module itself, as well as the subpage containing its documentation.
To manually create a module redirect, use the following syntax:
return require [[Module:Foo]]
Replace Foo
with the name of the module you'd like to redirect to.
Lua language
Tokens
A name (also called an identifier) in Lua can be any string of letters, digits, and underscores, not beginning with a digit. Names are case-sensitive; "foo", "Foo", and "FOO" are all different names.
The following keywords are reserved and may not be used as names:
and
break
do
else
elseif
end
false
for
function
if
in
local
nil
not
or
repeat
return
then
true
until
while
Names starting with an underscore followed by uppercase letters are reserved for internal Lua global variables.
Other tokens are:
#
%
(
)
*
+
,
-
--
.
..
...
/
:
;
<
<=
=
==
>
>=
[
]
^
{
}
~=
Comments
A comment starts with a --
anywhere outside a string. If the --
is immediately followed by an opening long bracket, the comment continues to the corresponding closing long bracket; otherwise the comment runs to the end of the current line.
-- A comment in Lua starts with a double-hyphen and runs to the end of the line.
--[[ Multi-line strings & comments
are adorned with double square brackets. ]]
--[=[ Comments like this can have other --[[comments]] nested. ]=]
--[==[ Comments like this can have other
--[===[ long --[=[comments]=] --nested
]===] multiple times, even if all of them are
--[[ not delimited with matching long brackets! ]===]
]==]
Data types
Lua is a dynamically-typed language, which means that variables and function arguments have no type, only the values assigned to them. All values carry a type.
Lua has eight basic data types, however only six are relevant to the Scribunto extension. The type()
function will return the type of a value.
The tostring()
function will convert a value to a string. The tonumber()
function will convert a value to a number if possible, and otherwise will return nil. There are no explicit functions to convert a value to other data types.
Numbers are automatically converted to strings when used where a string is expected, e.g. when used with the concatenation operator. Strings recognized by tonumber()
are automatically converted to numbers when used with arithmetic operators. When a boolean value is expected, all values other than nil and false are considered to be true.
nil
"nil"
is the data type of nil
, which exists to represent the absence of a value.
Nil may not be used as a key in a table, and there is no difference between an unassigned table key and a key assigned a nil value.
When converted to a string, the result is "nil"
. When converted to boolean, nil is considered false.
Note: Lua does make a distinction between nil
and nothing at all in some limited situations. For example, tostring(nil)
returns "nil"
, but tostring()
throws an error, as the first parameter is required. This distinction is particularly relevant to the select() function.
boolean
Boolean values are true
and false
.
When converted to a string, the result is "true" or "false".
Unlike many other languages, boolean values may not be directly converted to numbers. And unlike many other languages, only false and nil are considered false for boolean conversion; the number 0 and the empty string are both considered true.
string
Lua strings are considered a series of 8-bit bytes; it is up to the application to interpret them in any particular encoding.
String literals may be delimited by either single or double quotes ('
or "
); like JavaScript and unlike PHP, there is no difference between the two. The following escape sequences are recognized:
\a
(bell, byte 7)\b
(backspace, byte 8)\t
(horizontal tab, byte 9)\n
(newline, byte 10)\v
(vertical tab, byte 11)\f
(form feed, byte 12)\r
(carriage return, byte 13)\"
(double quote, byte 34)\'
(single quote, byte 39)\\
(backslash, byte 92)
A literal newline may also be included in a string by preceding it with a backslash. Bytes may also be specified using an escape sequence '\ddd', where ddd is the decimal value of the byte in the range 0â255. To include Unicode characters using escape sequences, the individual bytes for the UTF-8 encoding must be specified; in general, it will be more straightforward to enter the Unicode characters directly.
Literal strings can also be defined using long brackets.
An opening long bracket consists of an opening square bracket followed by zero or more equal signs followed by another opening square bracket, e.g. [[
, [=[
, or [=====[
.
The opening long bracket must be matched by the corresponding closing long bracket, e.g. ]]
, ]=]
, or ]=====]
.
As a special case, if an opening long bracket is immediately followed by a newline then the newline is not included in the string, but a newline just before the closing long bracket is kept.
Strings delimited by long brackets do not interpret escape sequences.
-- This long string
foo = [[
bar\tbaz
]]
-- is equivalent to this quote-delimited string
foo = 'bar\\tbaz\n'
Note that all strings are considered true when converted to boolean. This is unlike most other languages, where the empty string is usually considered false.
number
Lua has only one numeric type, which is typically represented internally as a 64-bit double-precision floating-point value. In this format, integers between -9007199254740991
(-253 + 1) and 9007199254740991
(253 - 1) may be represented exactly; larger numbers and numbers with a fractional part may suffer from round-off error.
Number constants are specified using a period (.
) as a decimal separator and without grouping separators, e.g. 123456.78
. Numbers may also be represented using E notation without spaces, e.g. 1.23e-10
, 123.45e20
, or 1.23E+5
. Integers may also be specified in hexadecimal notation using a 0x
prefix, e.g. 0x3A
.
A small number of number values are handled in a special way:
- Postive and negative infinity, which evalute to being greater or less than every other number, respectively (except the NaNs - see below). These can be accessed in two ways: through the
math
library, asmath.huge
and-math.huge
, or through numerical operations such as1/0
and-1/0
. - Lua occasionally distinguishes
0
and-0
(see the IEEE 754 for more information on signed zeros).0
and-0
are strictly equivalent for almost all purposes, but behave differently in a small number of numerical operations (e.g.1/0
returns infinity, while1/-0
returns negative infinity). The distinction also affects conversions from number to string (and vice-versa). - Positive and negative NaN (standing for Not a Number). No constant is provided for either of these, but
0/0
evaluates to negative NaN. Note that both NaNs have the unique quality that any comparison which involves them evaluates tofalse
(which means they do not even evaluate as being equal to themselves). The only practical distinction between the two is on type conversion to and from string (see below).
Note that all numbers (including 0
, -0
, the infinities and NaNs) are considered true when converted to boolean. This is unlike most other languages, where 0
is usually considered false. When converted to a string, finite numbers are represented in decimal, and E notation if 1014 or greater (e.g. "1e+14"
); the infinities are "inf"
and "-inf"
; and the NaNs are "nan"
and "-nan"
.
Known bug: the Lua interpreter will treat all instances of 0
and -0
as whichever of the two is encountered first when the script is compiled, which means that the return values of tostring(0)
, 1/-0
(and so on) are affected by where they occur in the code. This can cause unexpected results, particularly if all instances of 0
are converted to "-0"
on return. If necessary, this can be circumvented by generating zero values using tonumber("0")
and tonumber("-0")
, which doesn't seem to cause or be affected by the issue. See [1].
table
Lua tables are associative arrays, much like PHP arrays and JavaScript objects.
Tables are created using curly braces. The empty table is {}
. To populate fields on creation, a comma- and/or semicolon-separated list of field specifiers may be included in the braces. These take any of several forms:
[expression1] = expression2
uses the (first) value of expression1 as the key and the (first) value of expression2 as the value.name = expression
is equivalent to["name"] = expression
expression
is roughly equivalent to[i] = expression
, where i is an integer starting at 1 and incrementing with each field specification of this form. If this is the last field specifier and the expression has multiple values, all values are used; otherwise only the first is kept.
The fields in a table are accessed using bracket notation, e.g. table[key]
. String keys that are also valid names may also be accessed using dot notation, e.g. table.key
is equivalent to table['key']
. Calling a function that is a value in the table may use colon notation; for example, table:func( ... )
, which is equivalent to table['func']( table, ... )
or table.func( table, ... )
.
A sequence is a table with non-nil values for all positive integers from 1 to N and no value (nil) for all positive integers greater than N. Many Lua functions operate only on sequences, and ignore non-positive-integer keys.
Unlike many other languages such as PHP or JavaScript, any value except nil and NaN may be used as a key and no type conversion is performed. These are all valid and distinct:
-- Create table
t = {}
t["foo"] = "foo"
t.bar = "bar"
t[1] = "one"
t[2] = "two"
t[3] = "three"
t[12] = "the number twelve"
t["12"] = "the string twelve"
t[true] = "true"
t[tonumber] = "yes, even functions may be table keys"
t[t] = "yes, a table may be a table key too. Even in itself."
-- This creates a table roughly equivalent to the above
t2 = {
foo = "foo",
bar = "bar",
"one",
"two",
[12] = "the number twelve",
["12"] = "the string twelve",
"three",
[true] = "true",
[tonumber] = "yes, even functions may be table keys",
}
t2[t2] = "yes, a table may be a table key too. Even in itself."
Similarly, any value except nil may be stored as a value in a table. Storing nil is equivalent to deleting the key from the table, and accessing any key that has not been set will result in a nil value.
Note that tables are never implicitly copied in Lua; if a table is passed as an argument to the function and the function manipulates the keys or values in the table, those changes will be visible in the caller.
When converted to a string, the usual result is "table" but may be overridden using the __tostring
metamethod. Even the empty table is considered true as a boolean.
function
Functions in Lua are first-class values: they may be created anonymously, passed as arguments, assigned to variables, and so on.
Functions are created using the function
keyword, and called using parentheses. Syntactic sugar is available for named functions, local functions, and functions that act like member functions to a table. See Function declarations and Function calls below for details.
Lua functions are closures, meaning that they maintain a reference to the scope in which they are declared and can access and manipulate variables in that scope.
Like tables, if a function is assigned to a different variable or passed as an argument to another function, it is still the same underlying "function object" that will be called.
When converted to a string, the result is "function".
Unsupported types
The userdata type is used to hold opaque values for extensions to Lua written in other languages; for example, a userdata might be used to hold a C pointer or struct. To allow for use of Scribunto in hosting environments where custom-compiled code is not allowed, no such extensions are used.
The thread type represents the handles for coroutines, which are not available in Scribunto's sandbox.
Upvalues
There is a strict limit of max 60 unique upvalues acessed inside a function. An upvalue is a value declared outside of a function and used inside it. As upvalues do count:
- variables (a table with many elements counts as one upvalue)
- functions (only those directly called from function in question, not their dependencies)
A violation of the limit can be triggered by a use of such a variable or function, not by its mere presence or availability. Repeated access to same upvalue does not exhaust the limit further.
Metatables
Every table may have an associated table known as a metatable. The fields in the metatable are used by some operators and functions to specify different or fallback behavior for the table. The metatable for a table may be accessed using the getmetatable() function, and set with the setmetatable() function.
When being accessed for their meta functions, metatable fields are accessed as if with rawget().
Metatable fields that affect the table itself are:
- __index
- This is used when a table access
t[key]
would return nil. If the value of this field is a table, the access will be repeated in that table, i.e.__index[key]
(which may invoke that table's metatable's __index). If the value of this field is a function, the function will be called as__index( t, key )
. The rawget() function bypasses this metamethod. - __newindex
- This is used when assigning a key to a table
t[key] = value
whererawget( t, key )
would return nil. If the value of this field is a table, the assignment will be made to that table instead, i.e.__newindex[key] = value
(which may invoke that table's metatable's __newindex). If the value of this field is a function, the function will be called as__newindex( t, key, value )
. The rawset() function bypasses this metamethod. - __call
- This is used when function call syntax is used on a table,
t( ··· )
. The value must be a function, which is called as something like__call( t, ··· )
. - __mode
- This is used to make tables holding weak references. The value must be a string. By default, any value that is used as a key or as a value in a table will not be garbage collected. But if this metafield contains the letter 'k', keys may be garbage collected if there are no non-weak references, and if it contains 'v' values may be; in either case, both the corresponding key and value are removed from the table. Note that behavior is undefined if this field is altered after the table is used as a metatable.
Other metatable fields include:
Note: In Lua, all strings also share a single metatable, in which __index
refers to the string
table. This metatable is not accessible in Scribunto, nor is the referenced string
table; the string table available to modules is a copy.
Variables
Variables are places that store values. There are three kinds of variables in Lua: global variables, local variables, and table fields.
A name represents a global or local variable (or a function argument, which is just a kind of local variable). Variables are assumed to be global unless explicitly declared as local using the local
keyword. Any variable that has not been assigned a value is considered to have a nil value.
Global variables are stored in a standard Lua table called an environment; this table is often available as the global variable _G
. It is possible to set a metatable for this global variable table; the __index and __newindex metamethods will be called for accesses of and assignments to global variables just as they would for accesses of and assignments to fields in any other table.
The environment for a function may be accessed using the getfenv() function and changed using the setfenv() function; in Scribunto, these functions are severely restricted if they are available at all.
Local variables are lexically scoped; see Local variable declarations for details.
Expressions
An expression is something that has values: literals (numbers, strings, true, false, nil), anonymous function declarations, table constructors, variable references, function calls, the vararg expression, expressions wrapped in parentheses, unary operators applied to expressions, and expressions combined with binary operators.
Most expressions have one value; function calls and the vararg expression can have any number. Note that wrapping a function call or vararg expression in parentheses will lose all except the first value.
Expression lists are comma-separated lists of expressions. All except the last expression are forced to one value (dropping additional values, or using nil if the expression has no values); all values from the last expression are included in the values of the expression list.
Arithmetic operators
Lua supports the usual arithmetic operators: addition, subtraction, multiplication, division, modulo, exponentiation, and negation.
When all operands are numbers or strings for which tonumber() returns non-nil, the operations have their usual meaning.
If either operand is a table with an appropriate metamethod, the metamethod will be called.
Operator | Function | Example | Metamethod | Notes |
---|---|---|---|---|
+ | Addition | a + b | __add | |
- | Subtraction | a - b | __sub | |
* | Multiplication | a * b | __mul | |
/ | Division | a / b | __div | division by zero is not an error; NaN or infinity will be returned |
% | Modulo | a % b | __mod | defined as a % b == a - math.floor( a / b ) * b
|
^ | Exponentiation | a ^ b | __pow | non-integer exponents are allowed |
- | Negation | -a | __unm |
Relational operators
The relational operators in Lua are ==
, ~=
, <
, >
, <=
, and >=
. The result of a relational operator is always a boolean.
Equality (==
) first compares the types of its operands; if they are different, the result is false. Then it compares the values: nil, boolean, number, and string are compared in the expected manner. Functions are equal if they refer to the exact same function object; function() end == function() end
will return false, as it is comparing two different anonymous functions. Tables are by default compared in the same manner, but this may be changed using the __eq metamethod.
Inequality (~=
) is the exact negation of equality.
For the ordering operators, if both are numbers or both are strings, they are compared directly. Next, metamethods are checked:
a < b
uses__lt
a <= b
uses__le
if available, or if__lt
is available then it is considered equivalent tonot ( b < a )
a > b
is considered equivalent tob < a
a >= b
is considered equivalent tob <= a
If the necessary metamethods are not available, an error is raised.
Logical operators
The logical operators are and
, or
, and not
. All use the standard interpretation where nil and false are considered false and anything else is considered true.
For and
, if the left operand is considered false then it is returned and the second operand is not evaluated; otherwise the second operand is returned.
For or
, if the left operand is considered true then it is returned and the second operand is not evaluated; otherwise the second operand is returned.
For not
, the result is always true or false.
Note that and
and or
short circuit. For example, foo() or bar()
will only call bar()
if foo()
returns false or nil as its first value.
Concatenation operator
The concatenation operator is two dots, used as a .. b
. If both operands are numbers or strings, they are converted to strings and concatenated. Otherwise if a __concat metamethod is available, it is used. Otherwise, an error is raised.
Note that Lua strings are immutable and Lua does not provide any sort of "string builder", so a loop that repeatedly does a = a .. b
will have to create a new string for each iteration and eventually garbage-collect the old strings. If many strings need concatenating, it may be faster to use string.format() or to insert all the strings into a sequence and use table.concat() at the end.
Length operator
The length operator is #
, used as #a
. If a
is a string, it returns the length in bytes. If a
is a sequence table, it returns the length of the sequence.
If a
is a table that is not a sequence, the #a
may return 0 or any value N such that a[N] is not nil and a[N+1] is nil, even if there are non-nil values at higher indexes. For example,
-- This is not a sequence, because a[3] is nil and a[4] is not.
a = { 1, 2, nil, 4 }
-- This may output either 2 or 4.
-- And this may change even if the table is not modified.
mw.log( #a )
Operator precedence
Lua's operator precedence or order of operations, from highest to lowest:
- ^
- not # - (negation)
- * / %
- + - (subtraction)
- ..
- < > <= >= ~= ==
- and
- or
Within a precedence level, most binary operators are left-associative, i.e. a / b / c
is interpreted as (a / b) / c
. Exponentiation and concatenation are right-associative, i.e. a ^ b ^ c
is interpreted as a ^ (b ^ c)
.
Function calls
Lua function calls look like those in most other languages: a name followed by a list of arguments in parentheses:
func( expression-list )
As is usual with expression lists in Lua, the last expression in the list may supply multiple argument values.
If the function is called with fewer values in the expression list than there are arguments in the function definition, the extra arguments will have a nil value. If the expression list contains more values than there are arguments, the excess values are discarded. It is also possible for a function to take a variable number of arguments; see Function declarations for details.
Lua also allows direct calling of a function return value, i.e. func()()
. If an expression more complex than a variable access is needed to determine the function to be called, a parenthesized expression may be used in place of the variable access.
Lua has syntactic sugar for two common cases. The first is when a table is being used as an object, and the function is to be called as a method on the object. The syntax
table:name( expression-list )
is exactly equivalent to
table.name( table, expression-list )
The second common case is Lua's method of implementing named arguments by passing a table containing the name-to-value mappings as the only positional argument to the function. In this case, the parentheses around the argument list may be omitted. This also works if the function is to be passed a single literal string. For example, the calls
func{ arg1 = exp, arg2 = exp } func"string"
are equivalent to
func( { arg1 = exp, arg2 = exp } ) func( "string" )
These may be combined; the following calls are equivalent:
table:name{ arg1 = exp, arg2 = exp } table.name( table, { arg1 = exp, arg2 = exp } )
Function declarations
The syntax for function declaration looks like this:
function nameoptional ( var-listoptional )
block
end
All variables in var-list are local to the function, with values assigned from the expression list in the function call. Additional local variables may be declared inside the block.
When the function is called, the statements in block are executed after local variables corresponding to var-list are created and assigned values. If a return statement is reached, the block is exited and the values of the function call expression are those given by the return statement. If execution reaches the end of the function's block without encountering a return statement, the result of the function call expression has zero values.
Lua functions are lexical closures. A common idiom is to declare "private static" variables as locals in the scope where the function is declared. For example,
-- This returns a function that adds a number to its argument
function makeAdder( n )
return function( x )
-- The variable n from the outer scope is available here to be added to x
return x + n
end
end
local add5 = makeAdder( 5 )
mw.log( add5( 6 ) )
-- prints 11
A function may be declared to accept a variable number of arguments, by specifying ...
as the final item in the var-list:
Within the block, the varargs expression ...
may be used, with the result being all the extra values in the function call. For example,
local join = function ( separator, ... )
-- get the extra arguments as a new table
local args = { ... }
-- get the count of extra arguments, correctly
local n = select( '#', ... )
return table.concat( args, separator, 1, n )
end
join( ', ', 'foo', 'bar', 'baz' )
-- returns the string "foo, bar, baz"
The select() function is designed to work with the varargs expression; in particular, select( '#', ... )
should be used instead of #{ ... }
to count the number of values in the varargs expression, because { ... }
may not be a sequence.
Lua provides syntactic sugar to combine function declaration and assignment to a variable; see Function declaration statements for details.
Note that this will not work:
local factorial = function ( n )
if n <= 2 then
return n
else
return n * factorial( n - 1 )
end
end
Since the function declaration is processed before the local variable assignment statement is complete, "factorial" inside the function body refers to the (probably undefined) variable of that name in an outer scope. This problem may be avoided by declaring the local variable first and then assigning it in a subsequent statement, or by using the function declaration statement syntax.
Statements
A statement is the basic unit of execution: one assignment, control structure, function call, variable declaration, etc.
A chunk is a sequence of statements, optionally separated by semicolons. A chunk is basically considered the body of an anonymous function, so it can declare local variables, receive arguments, and return values.
A block is also a sequence of statements, just like a chunk. A block can be delimited to create a single statement: do block end
. These may be used to limit the scope of local variables, or to add a return
or break
in the middle of another block.
Assignments
variable-list = expression-list
The variable-list is a comma-separated list of variables; the expression-list is a comma-separated list of one or more expressions. All expressions are evaluated before any assignments are performed, so a, b = b, a
will swap the values of a and b.
Local variable declarations
local variable-list
local variable-list = expression-list
Local variables may be declared anywhere within a block or chunk. The first form, without an expression list, declares the variables but does not assign a value so all variables have nil as a value. The second form assigns values to the local variables, as described in Assignments above.
Note that visibility of the local variable begins with the statement after the local variable declaration. So a declaration like local x = x
declares a local variable x and assigns it the value of x from the outer scope. The local variable remains in scope until the end of the innermost block containing the local variable declaration.
Control structures
while exp do block end
The while statement repeats a block as long as an expression evaluates to a true value.
repeat block until exp
The repeat statement repeats a block until an expression evaluates to a true value. Local variables declared inside the block may be accessed in the expression.
for name = exp1, exp2, exp3 do block end
for name = exp1, exp2 do block end
This first form of the for loop will declare a local variable, and repeat the block for values from exp1 to exp2 adding exp3 on each iteration. Note that exp3 may be omitted entirely, in which case 1 is used, but non-numeric values such as nil
and false
are an error. All expressions are evaluated once before the loop is started.
This form of the for loop is roughly equivalent to
do
local var, limit, step = tonumber( exp1 ), tonumber( exp2 ), tonumber( exp3 )
if not ( var and limit and step ) then
error()
end
while ( step > 0 and var <= limit ) or ( step <= 0 and var >= limit ) do
local name = var
block
var = var + step
end
end
except that the variables var, limit, and step are not accessible anywhere else. Note that the variable name is local to the block; to use the value after the loop, it must be copied to a variable declared outside the loop.
for var-list in expression-list do block end
The second form of the for loop works with iterator functions. As in the first form, the expression-list is evaluated only once before beginning the loop.
This form of the for loop is roughly equivalent to
do
local func, static, var = expression-list
while true do
local var-list = func( static, var )
var = var1 -- ''var1'' is the first variable in ''var-list''
if var == nil then
break
end
block
end
end
except that again the variables func, static, and var are not accessible anywhere else. Note that the variables in var-list are local to the block; to use them after the loop, they must be copied to variables declared outside the loop.
Often the expression-list is a single function call that returns the three values. If the iterator function can be written so it only depends on the parameters passed into it, that would be the most efficient. If not, Programming in Lua suggests that a closure be preferred to returning a table as the static variable and updating its members on each iteration.
if exp1 then block1 elseif exp2 then block2 else block3 end
Executes block1 if exp1 returns true, otherwise executes block2 if exp2 returns true, and block3 otherwise. The else block3
portion may be omitted, and the elseif exp2 then block2
portion may be repeated or omitted as necessary.
return expression-list
The return statement is used to return values from a function or a chunk (which is just a function). The expression-list is a comma-separated list of zero or more expressions.
Lua implements tail calls: if expression-list consists of exactly one expression which is a function call, the current stack frame will be reused for the call to that function. This has implication for functions that deal with the call stack, such as getfenv()
and debug.traceback()
.
The return statement must be the last statement in its block. If for some reason a return is needed in the middle of a block, an explicit block do return end
may be used.
break
The break statement is used to terminate the execution of a while, repeat, or for loop, skipping to the next statement after the loop.
The break statement must be the last statement in its block. If for some reason a break is needed in the middle of a block, an explicit block do break end
may be used.
Unlike some other languages, Lua does not have a "continue" statement for loops (i.e. a statement to move onto the next iteration without breaking the loop altogether).
It is straightforward to achieve the same effect by nesting a repeat ... until true
block immediately inside the main loop, which will only ever iterate once for each iteration of the main loop (as its condition is always true).
Using break
will only end the inner loop, which has the practical effect of causing the main loop to continue onto the next iteration.
If it is necessary to use break
on the main loop, simply declare a variable which is checked each time the inner loop completes, and set it when necessary.
Function calls as statements
A function call may be used as a statement; in this case, the function is being called only for any side effects it may have (e.g. mw.log() logs values) and any return values are discarded.
Function declaration statements
Lua provides syntactic sugar to make declaring a function and assigning it to a variable more natural. The following pairs of declarations are equivalent
-- Basic declaration function func( var-list ) block end func = function ( var-list ) block end
-- Local function local function func( var-list ) block end local func; func = function ( var-list ) block end
-- Function as a field in a table function table.func( var-list ) block end table.func = function ( var-list ) block end
-- Function as a method in a table function table:func( var-list ) block end table.func = function ( self, var-list ) block end
Note the colon notation here parallels the colon notation for function calls, adding an implicit argument named self
at the beginning of the arguments list.
Error handling
Errors may be "thrown" using the error() and assert() functions. To "catch" errors, use pcall() or xpcall(). Note that certain internal Scribunto errors cannot be caught in Lua code.
Garbage collection
Lua performs automatic memory management. This means that you have to worry neither about allocating memory for new objects nor about freeing it when the objects are no longer needed. Lua manages memory automatically by running a garbage collector from time to time to collect all dead objects (that is, objects that are no longer accessible from Lua) and objects that are only reachable via weak references. All memory used by Lua is subject to automatic management: tables, functions, strings, etc.
Garbage collection happens automatically, and cannot be configured from within Scribunto.
Standard libraries
The standard Lua libraries provide essential services and performance-critical functions to Lua. Only those portions of the standard libraries that are available in Scribunto are documented here.
Basic functions
_G
This variable holds a reference to the current global variable table; the global variable foo
may also be accessed as _G.foo
. Note, however, that there is nothing special about _G itself; it may be reassigned in the same manner as any other variable:
foo = 1
mw.log( foo ) -- logs "1"
_G.foo = 2
mw.log( foo ) -- logs "2"
_G = {} -- _G no longer points to the global variable table
_G.foo = 3
mw.log( foo ) -- still logs "2"
The global variable table may be used just like any other table. For example,
-- Call a function whose name is stored in a variable
_G[var]()
-- Log the names and stringified values of all global variables
for k, v in pairs( _G ) do
mw.log( k, v )
end
-- Log the creation of new global variables
setmetatable( _G, {
__newindex = function ( t, k, v )
mw.log( "Creation of new global variable '" .. k .. "'" )
rawset( t, k, v )
end
} )
_VERSION
A string containing the running version of Lua, e.g. "Lua 5.1".
assert
assert( v, message, ... )
If v
is nil or false, issues an error. In this case, message
is used as the text of the error: if nil (or unspecified), the text is "assertion failed!"; if a string or number, the text is that value; otherwise assert itself will raise an error.
If v
is any other value, assert returns all arguments including v
and message
.
A somewhat common idiom in Lua is for a function to return a "true" value in normal operation, and on failure return nil or false as the first value and an error message as the second value. Easy error checking can then be implemented by wrapping the call in a call to assert
:
-- This doesn't check for errors
local result1, result2, etc = func( ... )
-- This works the same, but does check for errors
local result1, result2, etc = assert( func( ... ) )
error
error( message, level )
Issues an error, with text message
.
error
normally adds some information about the location of the error. If level
is 1 or omitted, that information is the location of the call to error
itself; 2 uses the location of the call of the function that called error; and so on. Passing 0 omits inclusion of the location information.
getfenv
getfenv( f )
Note this function may not be available, depending on allowEnvFuncs
in the engine configuration.
Returns an environment (global variable table), as specified by f
:
- If 1, nil, or omitted, returns the environment of the function calling
getfenv
. Often this will be the same as _G. - Integers 2â10 return the environment of functions higher in the call stack. For example, 2 returns the environment for the function that called the current function, 3 returns the environment for the function calling that function, and so on. An error will be raised if the value is higher than the number of function calls in the stack, or if the targeted stack level returned with a tail call.
- Passing a function returns the environment that will be used when that function is called.
The environments used by all standard library functions and Scribunto library functions are protected. Attempting to access these environments using getfenv
will return nil instead.
getmetatable
getmetatable( table )
Returns the metatable of a table. Any other type will return nil.
If the metatable has a __metatable
field, that value will be returned instead of the actual metatable.
ipairs
ipairs( t )
Returns three values: an iterator function, the table t
, and 0. This is intended for use in the iterator form of for
:
for i, v in ipairs( t ) do
-- process each index-value pair
end
This will iterate over the pairs ( 1, t[1] ), ( 2, t[2] ), and so on, stopping when t[i] would be nil.
The standard behavior may be overridden by providing an __ipairs
metamethod. If that metamethod exists, the call to ipairs will return the three values returned by __ipairs( t )
instead.
next
next( table, key )
This allows for iterating over the keys in a table. If key
is nil or unspecified, returns the "first" key in the table and its value; otherwise, it returns the "next" key and its value. When no more keys are available, returns nil. It is possible to check whether a table is empty using the expression next( t ) == nil
.
Note that the order in which the keys are returned is not specified, even for tables with numeric indexes. To traverse a table in numerical order, use a numerical for or ipairs.
Behavior is undefined if, when using next for traversal, any non-existing key is assigned a value. Assigning a new value (including nil) to an existing field is allowed.
pairs
pairs( t )
Returns three values: an iterator function (next or a work-alike), the table t
, and nil. This is intended for use in the iterator form of for
:
for k, v in pairs( t ) do
-- process each key-value pair
end
This will iterate over the key-value pairs in t
just as next would; see the documentation for next for restrictions on modifying the table during traversal.
The standard behavior may be overridden by providing a __pairs metamethod. If that metamethod exists, the call to pairs will return the three values returned by __pairs( t )
instead.
pcall
pcall( f, ... )
Calls the function f
with the given arguments in protected mode. This means that if an error is raised during the call to f
, pcall will return false and the error message raised. If no error occurs, pcall will return true and all values returned by the call.
In pseudocode, pcall
might be defined something like this:
function pcall( f, ... )
try
return true, f( ... )
catch ( message )
return false, message
end
end
rawequal
rawequal( a, b )
This is equivalent to a == b
except that it ignores any __eq metamethod.
rawget
rawget( table, k )
This is equivalent to table[k]
except that it ignores any __index metamethod.
rawset
rawset( table, k, v )
This is equivalent to table[k] = v
except that it ignores any __newindex metamethod.
select
select( index, ... )
If index
is a number, returns all arguments in ...
from that index onwards. If index
is the string "#"
, returns the number of arguments in ...
.
Note: unlike tables, lists of arguments (including the vararg expression ...
) treat nil
as a distinct value (see documentation for # and unpack for the problem with nil
in tables). For example:
select(2, "foo", "bar")
returns"bar"
.select(2, "foo", nil, "bar", nil)
returnsnil, "bar", nil
.select("#", "foo", "bar")
returns2
.select("#", "foo", "bar", nil)
returns3
.
In other words, select
is roughly like the following (except that it also handles any nil
arguments after the final non-nil argument):
function select( index, ... )
local t = { ... }
local maxindex = table.maxn( t )
if index == "#" then
return maxindex
else
return unpack( t, index, maxindex )
end
end
setmetatable
setmetatable( table, metatable )
Sets the metatable of a table. metatable
may be nil, but must be explicitly provided.
If the current metatable has a __metatable field, setmetatable
will throw an error.
tonumber
tonumber( value, base )
Tries to convert value
to a number. If it is already a number or a string convertible to a number, then tonumber
returns this number; otherwise, it returns nil.
The optional base
(default 10) specifies the base to interpret the numeral. The base may be any integer between 2 and 36, inclusive. In bases above 10, the letter 'A' (in either upper or lower case) represents 10, 'B' represents 11, and so forth, with 'Z' representing 35.
In base 10, the value may have a decimal part, be expressed in E notation, and may have a leading "0x" to indicate base 16. In other bases, only unsigned integers are accepted.
tostring
tostring( value )
Converts value
to a string. See Data types above for details on how each type is converted.
The standard behavior for tables may be overridden by providing a __tostring metamethod. If that metamethod exists, the call to tostring will return the single value returned by __tostring( value )
instead.
type
type( value )
Returns the type of value
as a string: "nil"
, "number"
, "string"
, "boolean"
, "table"
, or "function"
.
unpack
unpack( table, i, j )
Returns values from the given table, something like table[i], table[i+1], ···, table[j]
would do if written out manually. If nil or not given, i
defaults to 1 and j
defaults to #table
.
If the table does not have a value for a particular key, unpack will return nil for that value. For example, unpack({"foo", [3] = "bar"}, 1, 4)
returns "foo", nil, "bar", nil
.
Note that results are not deterministic if table
is not a sequence and j
is nil or unspecified; see Length operator for details.
xpcall
xpcall( f, errhandler )
This is much like pcall
, except that the error message is passed to the function errhandler
before being returned.
In pseudocode, xpcall
might be defined something like this:
function xpcall( f, errhandler )
try
return true, f()
catch ( message )
message = errhandler( message )
return false, message
end
end
Debug library
debug.traceback
debug.traceback( message, level )
Returns a string with a traceback of the call stack. An optional message string is appended at the beginning of the traceback. An optional level number tells at which stack level to start the traceback.
Math library
math.abs
math.abs( x )
Returns the absolute value of x
.
math.acos
math.acos( x )
Returns the arc cosine of x
(given in radians).
math.asin
math.asin( x )
Returns the arc sine of x
(given in radians).
math.atan
math.atan( x )
Returns the arc tangent of x
(given in radians).
math.atan2
math.atan2( y, x )
Returns the arc tangent of y/x
(given in radians), using the signs of both parameters to find the quadrant of the result.
math.ceil
math.ceil( x )
Returns the smallest integer larger than or equal to x
.
math.cos
math.cos( x )
Returns the cosine of x
(given in radians).
math.cosh
math.cosh( x )
Returns the hyperbolic cosine of x
.
math.deg
math.deg( x )
Returns the angle x
(given in radians) in degrees.
math.exp
math.exp( x )
Returns the value .
math.floor
math.floor( x )
Returns the largest integer smaller than or equal to x
.
math.fmod
math.fmod( x, y )
Returns the remainder of the division of x
by y
that rounds the quotient towards zero. For example, math.fmod( 10, 3 )
yields 1
.
math.frexp
math.frexp( x )
Returns two values m
and e
such that:
- If
x
is finite and non-zero: ,e
is an integer, and the absolute value ofm
is in the range - If
x
is zero:m
ande
are 0 - If
x
is NaN or infinite:m
isx
ande
is not specified
math.huge
The value representing positive infinity; larger than or equal to any other numerical value.
math.ldexp
math.ldexp( m, e )
Returns (e
should be an integer).
math.log
math.log( x )
Returns the natural logarithm of x
.
math.log10
math.log10( x )
Returns the base-10 logarithm of x
.
math.max
math.max( x, ... )
Returns the maximum value among its arguments.
Behavior with NaNs is not specified. With the current implementation, NaN will be returned if x
is NaN, but any other NaNs will be ignored.
math.min
math.min( x, ... )
Returns the minimum value among its arguments.
Behavior with NaNs is not specified. With the current implementation, NaN will be returned if x
is NaN, but any other NaNs will be ignored.
math.modf
math.modf( x )
Returns two numbers, the integral part of x
and the fractional part of x
. For example, math.modf( 1.25 )
yields 1, 0.25
.
math.pi
The value of .
math.pow
math.pow( x, y )
Equivalent to x^y
.
math.rad
math.rad( x )
Returns the angle x
(given in degrees) in radians.
math.random
math.random( m, n )
Returns a pseudo-random number.
The arguments m
and n
may be omitted, but if specified must be convertible to integers.
- With no arguments, returns a real number in the range
- With one argument, returns an integer in the range
- With two arguments, returns an integer in the range
Note that incorrect output may be produced if m
or n
are less than â2147483648 or greater than 2147483647, or if n - m
is greater than 2147483646.
math.randomseed
math.randomseed( x )
Sets x
as the seed for the pseudo-random generator.
Note that using the same seed will cause math.random
to output the same sequence of numbers.
math.sin
math.sin( x )
Returns the sine of x
(given in radians).
math.sinh
math.sinh( x )
Returns the hyperbolic sine of x
.
math.sqrt
math.sqrt( x )
Returns the square root of x
. Equivalent to x^0.5
.
math.tan
math.tan( x )
Returns the tangent of x
(given in radians).
math.tanh
math.tanh( x )
Returns the hyperbolic tangent of x
.
Operating system library
os.clock
os.clock()
Returns an approximation of the amount in seconds of CPU time used by the program.
os.date
os.date( format, time )
- Language library's formatDate may be used for more comprehensive date formatting
Returns a string or a table containing date and time, formatted according to format
. If the format is omitted or nil, "%c" is used.
If time
is given, it is the time to be formatted (see os.time()
). Otherwise the current time is used.
If format
starts with '!', then the date is formatted in UTC rather than the server's local time. After this optional character, if format is the string "*t", then date returns a table with the following fields:
- year (full)
- month (1â12)
- day (1â31)
- hour (0â23)
- min (0â59)
- sec (0â60, to allow for leap seconds)
- wday (weekday, Sunday is 1)
- yday (day of the year)
- isdst (daylight saving flag, a boolean; may be absent if the information is not available)
If format is not "*t", then date returns the date as a string, formatted according to the same rules as the C function strftime.
os.difftime
os.difftime( t2, t1 )
Returns the number of seconds from t1
to t2
.
os.time
os.time( table )
Returns a number representing the current time.
When called without arguments, returns the current time. If passed a table, the time encoded in the table will be parsed. The table must have the fields "year", "month", and "day", and may also include "hour" (default 12), "min" (default 0), "sec" (default 0), and "isdst".
Package library
require
require( modulename )
Loads the specified module.
First, it looks in package.loaded[modulename]
to see if the module is already loaded. If so, returns package.loaded[modulename]
.
Otherwise, it calls each loader in the package.loaders
sequence to attempt to find a loader for the module. If a loader is found, that loader is called. The value returned by the loader is stored into package.loaded[modulename]
and is returned.
See the documentation for package.loaders
for information on the loaders available.
For example, if you have a module "Module:Giving" containing the following:
local p = {}
p.someDataValue = 'Hello!'
return p
You can load this in another module with code such as this:
local giving = require( "Module:Giving" )
local value = giving.someDataValue -- value is now 'Hello!'
package.loaded
This table holds the loaded modules. The keys are the module names, and the values are the values returned when the module was loaded.
package.loaders
This table holds the sequence of searcher functions to use when loading modules. Each searcher function is called with a single argument, the name of the module to load. If the module is found, the searcher must return a function that will actually load the module and return the value to be returned by require. Otherwise, it must return nil.
Scribunto provides two searchers:
- Look in
package.preload[modulename]
for the loader function - Look in the modules provided with Scribunto for the module name, and if that fails look in the Module: namespace. The "Module:" prefix must be provided.
Note that the standard Lua loaders are not included.
package.preload
This table holds loader functions, used by the first searcher Scribunto includes in package.loaders.
package.seeall
package.seeall( table )
Sets the __index metamethod for table
to _G.
String library
In all string functions, the first character is at position 1, not position 0 as in C, PHP, and JavaScript. Indexes may be negative, in which case they count from the end of the string: position -1 is the last character in the string, -2 is the second-last, and so on.
Warning: The string library assumes one-byte character encodings. It cannot handle Unicode characters. To operate on Unicode strings, use the corresponding methods in the Scribunto Ustring library.
string.byte
string.byte( s, i, j )
If the string is considered as an array of bytes, returns the byte values for s[i]
, s[i+1]
, ···, s[j]
.
The default value for i
is 1;
the default value for j
is i
.
Identical to mw.ustring.byte().
string.char
string.char( ... )
Receives zero or more integers. Returns a string with length equal to the number of arguments, in which each character has the byte value equal to its corresponding argument.
local value = string.char( 0x48, 0x65, 0x6c, 0x6c, 0x6f, 0x21 ) --value is now 'Hello!'
See mw.ustring.char() for a similar function that uses Unicode codepoints rather than byte values.
string.find
string.find( s, pattern, init, plain )
Looks for the first match of pattern
in the string s
. If it finds a match, then find
returns the offsets in s
where this occurrence starts and ends; otherwise, it returns nil. If the pattern has captures, then in a successful match the captured values are also returned after the two indices.
A third, optional numerical argument init
specifies where to start the search; its default value is 1 and can be negative. A value of true as a fourth, optional argument plain
turns off the pattern matching facilities, so the function does a plain "find substring" operation, with no characters in pattern
being considered "magic".
Note that if plain
is given, then init
must be given as well.
See mw.ustring.find() for a similar function extended as described in Ustring patterns and where the init
offset is in characters rather than bytes.
string.format
string.format( formatstring, ... )
Returns a formatted version of its variable number of arguments following the description given in its first argument (which must be a string).
The format string uses a limited subset of the printf
format specifiers:
- Recognized flags are '-', '+', ' ', '#', and '0'.
- Integer field widths up to 99 are supported. '*' is not supported.
- Integer precisions up to 99 are supported. '*' is not supported.
- Length modifiers are not supported.
- Recognized conversion specifiers are 'c', 'd', 'i', 'o', 'u', 'x', 'X', 'e', 'E', 'f', 'g', 'G', 's', '%', and the non-standard 'q'.
- Positional specifiers (e.g. "%2$s") are not supported.
The conversion specifier 'q' is like 's', but formats the string in a form suitable to be safely read back by the Lua interpreter: the string is written between double quotes, and all double quotes, newlines, embedded zeros, and backslashes in the string are correctly escaped when written.
Conversion between strings and numbers is performed as specified in Data types; other types are not automatically converted to strings. Strings containing NUL characters (byte value 0) are not properly handled.
Identical to mw.ustring.format().
string.gmatch
string.gmatch( s, pattern )
Returns an iterator function that, each time it is called, returns the next captures from pattern
over string s
. If pattern
specifies no captures, then the whole match is produced in each call.
For this function, a '^
' at the start of a pattern is not magic, as this would prevent the iteration. It is treated as a literal character.
See mw.ustring.gmatch() for a similar function for which the pattern is extended as described in Ustring patterns.
string.gsub
string.gsub( s, pattern, repl, n )
Returns a copy of s
in which all (or the first n
, if given) occurrences of the pattern
have been replaced by a replacement string specified by repl
, which can be a string, a table, or a function. gsub
also returns, as its second value, the total number of matches that occurred.
If repl
is a string, then its value is used for replacement. The character %
works as an escape character: any sequence in repl
of the form %d
,
with d between 1 and 9, stands for the value of the d-th captured substring. The sequence %0
stands for the whole match, and the sequence %%
stands for a single %
.
If repl
is a table, then the table is queried for every match, using the first capture as the key; if the pattern specifies no captures, then the whole match is used as the key.
If repl
is a function, then this function is called every time a match occurs, with all captured substrings passed as arguments, in order; if the pattern specifies no captures, then the whole match is passed as a sole argument.
If the value returned by the table query or by the function call is a string or a number, then it is used as the replacement string; otherwise, if it is false or nil, then there is no replacement (that is, the original match is kept in the string).
See mw.ustring.gsub() for a similar function in which the pattern is extended as described in Ustring patterns.
string.len
string.len( s )
Returns the length of the string, in bytes. Is not confused by ASCII NUL characters. Equivalent to #s
.
See mw.ustring.len() for a similar function using Unicode codepoints rather than bytes.
string.lower
string.lower( s )
Returns a copy of this string with all ASCII uppercase letters changed to lowercase. All other characters are left unchanged.
See mw.ustring.lower() for a similar function in which all characters with uppercase to lowercase definitions in Unicode are converted.
string.match
string.match( s, pattern, init )
Looks for the first match of pattern
in the string. If it finds one, then match
returns the captures from the pattern; otherwise it returns nil. If pattern
specifies no captures, then the whole match is returned.
A third, optional numerical argument init
specifies where to start the search; its default value is 1 and can be negative.
See mw.ustring.match() for a similar function in which the pattern is extended as described in Ustring patterns and the init
offset is in characters rather than bytes.
string.rep
string.rep( s, n )
Returns a string that is the concatenation of n
copies of the string s
. Identical to mw.ustring.rep().
string.reverse
string.reverse( s )
Returns a string that is the string s
reversed (bytewise).
string.sub
string.sub( s, i, j )
Returns the substring of s
that starts at i
and continues until j
; i
and j
can be negative. If j
is nil or omitted, it will continue until the end of the string.
In particular, the call string.sub(s,1,j)
returns a prefix of s
with length j
, and string.sub(s, -i)
returns a suffix of s
with length i
.
See mw.ustring.sub() for a similar function in which the offsets are characters rather than bytes.
string.ulower
string.ulower( s )
An alias for mw.ustring.lower().
string.upper
string.upper( s )
Returns a copy of this string with all ASCII lowercase letters changed to uppercase. All other characters are left unchanged.
See mw.ustring.upper() for a similar function in which all characters with lowercase to uppercase definitions in Unicode are converted.
string.uupper
string.uupper( s )
An alias for mw.ustring.upper().
Patterns
Note that Lua's patterns are similar to regular expressions, but are not identical. In particular, note the following differences from regular expressions and PCRE:
- The quoting character is percent (
%
), not backslash (\
). - Dot (
.
) always matches all characters, including newlines. - No case-insensitive mode.
- No alternation (the
|
operator). - Quantifiers (
*
,+
,?
, and-
) may only be applied to individual characters or character classes, not to capture groups. - The only non-greedy quantifier is
-
, which is equivalent to PCRE's*?
quantifier. - No generalized finite quantifier (e.g. the
{n,m}
quantifier in PCRE). - The only zero-width assertions are
^
,$
, and the%f[set]
"frontier" pattern; assertions such as PCRE's\b
or(?=···)
are not present. - Patterns themselves do not recognize character escapes such as "\ddd". However, since patterns are strings these sort of escapes may be used in the string literals used to create the pattern-string.
Also note that a pattern cannot contain embedded zero bytes (ASCII NUL, "\0"
). Use %z
instead.
Also see Ustring patterns for a similar pattern-matching scheme using Unicode characters.
Character class
A character class is used to represent a set of characters. The following combinations are allowed in describing a character class:
x
|
(where x is not one of the magic characters ^$()%.[]*+-? ) represents the character x itself.
|
---|---|
.
|
(a dot) Represents all characters. |
%a
|
Represents all ASCII letters. |
%c
|
Represents all ASCII control characters. |
%d
|
Represents all digits. |
%l
|
Represents all ASCII lowercase letters. |
%p
|
Represents all punctuation characters. |
%s
|
Represents all ASCII space characters. |
%u
|
Represents all ASCII uppercase letters. |
%w
|
Represents all ASCII alphanumeric characters. |
%x
|
Represents all hexadecimal digits. |
%z
|
Represents ASCII NUL, the zero byte. |
%A
|
All characters not in %a .
|
%C
|
All characters not in %c .
|
%D
|
All characters not in %d .
|
%L
|
All characters not in %l .
|
%P
|
All characters not in %p .
|
%S
|
All characters not in %s .
|
%U
|
All characters not in %u .
|
%W
|
All characters not in %w .
|
%X
|
All characters not in %x .
|
%Z
|
All characters not in %z .
|
%y
|
(where y is any non-alphanumeric character) represents the character y . This is the standard way to escape the magic characters. Any punctuation character (even the non magic) can be preceded by a '% ' when used to represent itself in a pattern.
|
[set]
|
Represents the class which is the union of all characters in set. A range of characters can be specified by separating the end characters of the range with a ' The interaction between ranges and classes is not defined. Therefore, patterns like |
[^set]
|
Represents the complement of set, where set is interpreted as above. |
Pattern items
A pattern item can be
- a single character class, which matches any single character in the class;
- a single character class followed by '
*
', which matches 0 or more repetitions of characters in the class. These repetition items will always match the longest possible sequence; - a single character class followed by '
+
', which matches 1 or more repetitions of characters in the class. These repetition items will always match the longest possible sequence; - a single character class followed by '
-
', which also matches 0 or more repetitions of characters in the class. Unlike '*
', these repetition items will always match the shortest possible sequence; - a single character class followed by '
?
', which matches 0 or 1 occurrence of a character in the class; %n
, for n between 1 and 9; such item matches a substring equal to the n-th captured string (see below);%bxy
, where x and y are two distinct characters; such item matches strings that start with x, end with y, and where the x and y are balanced. This means that, if one reads the string from left to right, counting +1 for an x and -1 for a y, the ending y is the first y where the count reaches 0. For instance, the item%b()
matches expressions with balanced parentheses.%f[set]
, a frontier pattern; such item matches an empty string at any position such that the next character belongs to set and the previous character does not belong to set. The set set is interpreted as previously described. The beginning and the end of the subject are handled as if they were the character '\0'.
Note that frontier patterns were present but undocumented in Lua 5.1, and officially added to Lua in 5.2. The implementation in Lua 5.2.1 is unchanged from that in 5.1.0.
Pattern
A pattern is a sequence of pattern items.
A ^
at the beginning of a pattern anchors the match at the beginning of the subject string. A $
at the end of a pattern anchors the match at the end of the subject string. At other positions, ^
and $
have no special meaning and represent themselves.
Captures
A pattern can contain sub-patterns enclosed in parentheses; they describe captures. When a match succeeds, the substrings of the subject string that match captures are stored ("captured") for future use. Captures are numbered according to their left parentheses. For instance, in the pattern (a*(.)%w(%s*))
, the part of the string matching a*(.)%w(%s*)
is stored as the first capture (and therefore has number 1); the character matching .
is captured with number 2, and the part matching %s*
has number 3.
Capture references can appear in the pattern string itself, and refer back to text that was captured earlier in the match. For example, ([a-z])%1
will match any pair of identical lowercase letters, while ([a-z])([a-z])([a-z])[a-z]%3%2%1
will match any 7-letter palindrome.
As a special case, the empty capture ()
captures the current string position (a number). For instance, if we apply the pattern "()aa()"
on the string "flaaap"
, there will be two captures: 3 and 5.
Known limitations: Unlike Ustring library patterns, String library patterns may not contain more than 32 captures. If the pattern has more, then the String function will throw an error. Because the Ustring library has its own maximum of 10,000 bytes for patterns (unlike the String library), it is therefore impossible to use a pattern which exceeds both limits, as it will be incompatible with both libraries.
Table library
Most functions in the table library assume that the table represents a sequence.
The functions table.foreach()
, table.foreachi()
, and table.getn()
may be available but are deprecated; use a for loop with pairs(), a for loop with ipairs(), and the length operator instead. The function table.setn()
is completely obsolete, however, and will throw an error if used.
table.concat
table.concat( table, sep, i, j )
Given an array where all elements are strings or numbers, returns table[i] .. sep .. table[i+1] ··· sep .. table[j]
.
The default value for sep
is an empty string, the default for i
is 1, and the default for j
is the length of the table. If i
is greater than j
, it returns an empty string.
table.insert
table.insert( table, value )
table.insert( table, pos, value )
Inserts element value
at position pos
in table
, shifting up other elements to open space, if necessary. The default value for pos
is the length of the table plus 1, so that a call table.insert(t, x)
inserts x
at the end of table t
.
Elements up to #table
are shifted; see Length operator for caveats if the table is not a sequence.
Note: when using the pos
parameter, value
should not be nil
. Attempting to insert an explicit nil
value into the middle of a table will result in undefined behaviour, which may delete elements in the table unpredictably.
table.maxn
table.maxn( table )
Returns the largest positive numerical index of the given table, or zero if the table has no positive numerical indices.
To do this, it iterates over the whole table. This is roughly equivalent to
function table.maxn( table )
local maxn, k = 0, nil
repeat
k = next( table, k )
if type( k ) == 'number' and k > maxn then
maxn = k
end
until not k
return maxn
end
table.remove
table.remove( table, pos )
Removes from table
the element at position pos
,
shifting down other elements to close the space, if necessary. Returns the value of the removed element. The default value for pos
is the length of the table, so that a call table.remove( t )
removes the last element of table t
.
Elements up to #table
are shifted; see Length operator for caveats if the table is not a sequence.
table.sort
table.sort( table, comp )
Sorts table elements in a given order, in-place, from table[1]
to table[#table]
.
If comp
is given, then it must be a function that receives two table elements, and returns true when the first is less than the second (so that not comp(a[i+1],a[i])
will be true after the sort).
If comp
is not given, then the standard Lua operator <
is used instead.
The sort algorithm is not stable; that is, elements considered equal by the given order may have their relative positions changed by the sort.
Scribunto libraries
All Scribunto libraries are located in the table mw
.
Base functions
mw.addWarning
mw.addWarning( text )
Adds a warning which is displayed above the preview when previewing an edit. text
is parsed as wikitext.
mw.allToString
mw.allToString( ... )
Calls tostring() on all arguments, then concatenates them with tabs as separators.
mw.clone
mw.clone( value )
Creates a deep copy of a value. All tables (and their metatables) are reconstructed from scratch. Functions are still shared, however.
mw.getCurrentFrame
mw.getCurrentFrame()
Returns the current frame object, typically the frame object from the most recent #invoke
.
mw.incrementExpensiveFunctionCount
mw.incrementExpensiveFunctionCount()
Adds one to the "expensive parser function" count, and throws an exception if it exceeds the limit (see $wgExpensiveParserFunctionLimit
).
mw.isSubsting
mw.isSubsting()
Returns true if the current #invoke
is being substed, false otherwise. See Returning text above for discussion on differences when substing versus not substing.
mw.loadData
mw.loadData( module )
Sometimes a module needs large tables of data; for example, a general-purpose module to convert units of measure might need a large table of recognized units and their conversion factors. And sometimes these modules will be used many times in one page. Parsing the large data table for every {{#invoke:}}
can use a significant amount of time. To avoid this issue, mw.loadData()
is provided.
mw.loadData
works like require()
, with the following differences:
- The loaded module is evaluated only once per page, rather than once per
{{#invoke:}}
call. - The loaded module is not recorded in
package.loaded
. - The value returned from the loaded module must be a table. Other data types are not supported.
- The returned table (and all subtables) may contain only booleans, numbers, strings, and other tables. Other data types, particularly functions, are not allowed.
- The returned table (and all subtables) may not have a metatable.
- All table keys must be booleans, numbers, or strings.
- The table actually returned by
mw.loadData()
has metamethods that provide read-only access to the table returned by the module. Since it does not contain the data directly,pairs()
andipairs()
will work but other methods, including#value
,next()
, and the functions in the Table library, will not work correctly.
The hypothetical unit-conversion module mentioned above might store its code in "Module:Convert" and its data in "Module:Convert/data", and "Module:Convert" would use local data = mw.loadData( 'Module:Convert/data' )
to efficiently load the data.
mw.loadJsonData
mw.loadJsonData( page )
This is the same as mw.loadData()
above, except it loads data from JSON pages rather than Lua tables.
The JSON content must be an array or object.
See also mw.text.jsonDecode()
.
mw.dumpObject
mw.dumpObject( object )
Serializes object
to a human-readable representation, then returns the resulting string.
mw.log
mw.log( ... )
Passes the arguments to mw.allToString(), then appends the resulting string to the log buffer.
In the debug console, the function print()
is an alias for this function.
mw.logObject
mw.logObject( object )
mw.logObject( object, prefix )
Calls mw.dumpObject() and appends the resulting string to the log buffer. If prefix
is given, it will be added to the log buffer followed by an equals sign before the serialized string is appended (i.e. the logged text will be "prefix = object-string").
Frame object
The frame object is the interface to the parameters passed to {{#invoke:}}
, and to the parser.
Note that there is no frame library, and there is no global variable named frame
. A frame object is typically obtained by being passed as a parameter to the function called by {{#invoke:}}
, and can also be obtained from mw.getCurrentFrame()
.
frame.args
A table for accessing the arguments passed to the frame. For example, if a module is called from wikitext with
{{#invoke:module|function|arg1|arg2|name=arg3}}
then frame.args[1]
will return "arg1"
, frame.args[2]
will return "arg2"
, and frame.args['name']
(or frame.args.name
) will return "arg3"
. It is also possible to iterate over arguments using pairs( frame.args )
or ipairs( frame.args )
.
However, due to how Lua implements table iterators, iterating over arguments will return them in an unspecified order, and there's no way to know the original order as they appear in wikitext.
Note that values in this table are always strings; tonumber()
may be used to convert them to numbers, if necessary. Keys, however, are numbers even if explicitly supplied in the invocation: {{#invoke:module|function|1|2=2}}
gives string values "1"
and "2"
indexed by numeric keys 1
and 2
.
As in MediaWiki template invocations, named arguments will have leading and trailing whitespace removed from both the name and the value before they are passed to Lua, whereas unnamed arguments will not have whitespace stripped.
For performance reasons, frame.args
uses a metatable, rather than directly containing the arguments. Argument values are requested from MediaWiki on demand. This means that most other table methods will not work correctly, including #frame.args
, next( frame.args )
, and the functions in the Table library.
If preprocessor syntax such as template invocations and triple-brace arguments are included within an argument to #invoke
, they will not be expanded, after being passed to Lua, until their values are being requested in Lua. If certain special tags written in XML notation, such as <pre>
, <nowiki>
, <gallery>
and <ref>
, are included as arguments to #invoke
, then these tags will be converted to "strip markers" â special strings which begin with a delete character (ASCII 127), to be replaced with HTML after they are returned from #invoke.
frame:callParserFunction
frame:callParserFunction( name, args )
frame:callParserFunction( name, ... )
frame:callParserFunction{ name = string, args = table }
- Note the use of named arguments.
Call a parser function, returning an appropriate string. This is preferable to frame:preprocess
, but whenever possible, native Lua functions or Scribunto library functions should be preferred to this interface.
The following calls are approximately equivalent to the indicated wikitext:
-- {{ns:0}}
frame:callParserFunction( 'ns', { 0 } )
frame:callParserFunction( 'ns', 0 )
frame:callParserFunction{ name = 'ns', args = { 0 } }
-- {{#tag:nowiki|some text}}
frame:callParserFunction( '#tag', { 'nowiki', 'some text' } )
frame:callParserFunction( '#tag', 'nowiki', 'some text' )
frame:callParserFunction( '#tag:nowiki', 'some text' )
frame:callParserFunction{ name = '#tag', args = { 'nowiki', 'some text' } }
-- {{#tag:ref|some text|name=foo|group=bar}}
frame:callParserFunction( '#tag', { 'ref',
'some text', name = 'foo', group = 'bar'
} )
Note that, as with frame:expandTemplate(), the function name and arguments are not preprocessed before being passed to the parser function.
frame:expandTemplate
frame:expandTemplate{ title = title, args = table }
- Note the use of named arguments.
This is equivalent to a call to frame:callParserFunction() with function name 'msg'
(see Help:Magic words#Transclusion modifiers) and with title
prepended to args
.
This is transclusion. The call:
frame:expandTemplate{ title = 'template', args = { 'arg1', 'arg2', name = 'arg3' } }
does roughly the same thing from Lua that {{template|arg1|arg2|name=arg3}}
does in wikitext. As in transclusion, if the passed title does not contain a namespace prefix it will be assumed to be in the Template: namespace.
Note that the title and arguments are not preprocessed before being passed into the template:
-- This is roughly equivalent to wikitext like {{template|{{!}}}}
frame:expandTemplate{ title = 'template', args = { '|' } }
frame:callParserFunction{ 'msg', { 'template', '|' } }
-- This is roughly equivalent to wikitext like {{template|{{((}}!{{))}}}}
frame:expandTemplate{ title = 'template', args = { '{{!}}' } }
frame:callParserFunction{ 'msg', { 'template', '{{!}}' } }
frame:extensionTag
frame:extensionTag( name, content, args )
frame:extensionTag{ name = string, content = string, args = table_or_string }
This is equivalent to a call to frame:callParserFunction() with function name '#tag'
(see Help:Magic_words#Miscellaneous) and with name
and content
prepended to args
.
-- These are equivalent
frame:extensionTag( 'ref', 'some text', { name = 'foo', group = 'bar' } )
frame:extensionTag{ name = 'ref', content = 'some text', args = { name = 'foo', group = 'bar' } }
frame:callParserFunction( '#tag', { 'ref' ,
'some text', name = 'foo', group = 'bar'
} )
-- These are equivalent
frame:extensionTag{ name = 'ref', content = 'some text', args = { 'some other text' } }
frame:callParserFunction( '#tag', { 'ref',
'some text', 'some other text'
} )
frame:getParent
frame:getParent()
Called on the frame created by {{#invoke:}}
, returns the frame for the page that called {{#invoke:}}
. Called on that frame, returns nil.
For instance, if the template {{Example}}
contains the code {{#invoke:ModuleName|FunctionName|A|B}}
, and a page transcludes that template with the code {{Example|C|D}}
, then in Module:ModuleName, calling frame.args[1]
and frame.args[2]
returns "A"
and "B"
, and calling frame:getParent().args[1]
and frame:getParent().args[2]
returns "C"
and "D"
, with frame
being the first argument in the function call.
frame:getTitle
frame:getTitle()
Returns the title associated with the frame as a string. For the frame created by {{#invoke:}}
, this is the title of the module invoked.
frame:newChild
frame:newChild{ title = title, args = table }
- Note the use of named arguments.
Create a new Frame object that is a child of the current frame, with optional arguments and title.
This is mainly intended for use in the debug console for testing functions that would normally be called by {{#invoke:}}
. The number of frames that may be created at any one time is limited.
frame:preprocess
frame:preprocess( string )
frame:preprocess{ text = string }
This expands wikitext in the context of the frame, i.e. templates, parser functions, and parameters such as {{{1}}}
are expanded, and returns the expanded text. Certain special tags written in XML-style notation, such as <pre>
, <nowiki>
, <gallery>
and <ref>
, will be replaced with "strip markers" â special strings which begin with a delete character (ASCII 127), to be replaced with HTML after they are returned from #invoke
.
If you are expanding a single template, use frame:expandTemplate
instead of trying to construct a wikitext string to pass to this method. It's faster and less prone to error if the arguments contain pipe characters or other wikimarkup.
If you are expanding a single parser function, use frame:callParserFunction
for the same reasons.
frame:getArgument
frame:getArgument( arg )
frame:getArgument{ name = arg }
Gets an object for the specified argument, or nil if the argument is not provided.
The returned object has one method, object:expand()
, that returns the expanded wikitext for the argument.
frame:newParserValue
frame:newParserValue( text )
frame:newParserValue{ text = text }
Returns an object with one method, object:expand()
, that returns the result of frame:preprocess( text )
.
frame:newTemplateParserValue
frame:newTemplateParserValue{ title = title, args = table }
- Note the use of named arguments.
Returns an object with one method, object:expand()
, that returns the result of frame:expandTemplate
called with the given arguments.
frame:argumentPairs
frame:argumentPairs()
Same as pairs( frame.args )
. Included for backwards compatibility.
Hash library
mw.hash.hashValue
mw.hash.hashValue( algo, value )
Hashes a string value with the specified algorithm. Valid algorithms may be fetched using mw.hash.listAlgorithms().
mw.hash.listAlgorithms
mw.hash.listAlgorithms()
Returns a list of supported hashing algorithms, for use in mw.hash.hashValue().
HTML library
mw.html
is a fluent interface for building complex HTML from Lua. A mw.html object can be created using mw.html.create
.
Functions documented as mw.html.name
are available on the global mw.html
table; functions documented as mw.html:name
and html:name
are methods of an mw.html object (see mw.html.create
).
A basic example could look like this:
local div = mw.html.create( 'div' )
div
:attr( 'id', 'testdiv' )
:css( 'width', '100%' )
:wikitext( 'Some text' )
:tag( 'hr' )
return tostring( div )
-- Output: <div id="testdiv" style="width:100%;">Some text<hr /></div>
mw.html.create
mw.html.create( tagName, args )
Creates a new mw.html object containing a tagName
html element. You can also pass an empty string or nil as tagName
in order to create an empty mw.html object.
args
can be a table with the following keys:
args.selfClosing
: Force the current tag to be self-closing, even if mw.html doesn't recognize it as self-closingargs.parent
: Parent of the current mw.html instance (intended for internal usage)
mw.html:node
html:node( builder )
Appends a child mw.html (builder
) node to the current mw.html instance. If a nil parameter is passed, this is a no-op. A (builder
) node is a string representation of an html element.
mw.html:wikitext
html:wikitext( ... )
Appends an undetermined number of wikitext strings to the mw.html object.
Note that this stops at the first nil item.
mw.html:newline
html:newline()
Appends a newline to the mw.html object.
mw.html:tag
html:tag( tagName, args )
Appends a new child node with the given tagName
to the builder, and returns a mw.html instance representing that new node. The args
parameter is identical to that of mw.html.create
Note that contrarily to other methods such as html:node()
, this method doesn't return the current mw.html instance, but the mw.html instance of the newly inserted tag.
Make sure to use html:done()
to go up to the parent mw.html instance, or html:allDone()
if you have nested tags on several levels.
mw.html:attr
html:attr( name, value )
html:attr( table )
Set an HTML attribute with the given name
and value
on the node. Alternatively a table holding name->value pairs of attributes to set can be passed. In the first form, a value of nil causes any attribute with the given name to be unset if it was previously set.
mw.html:getAttr
html:getAttr( name )
Get the value of a html attribute previously set using html:attr()
with the given name
.
mw.html:addClass
html:addClass( class )
Adds a class name to the node's class attribute. If a nil parameter is passed, this is a no-op.
mw.html:css
html:css( name, value )
html:css( table )
Set a CSS property with the given name
and value
on the node. Alternatively a table holding name->value pairs of properties to set can be passed. In the first form, a value of nil causes any property with the given name to be unset if it was previously set.
mw.html:cssText
html:cssText( css )
Add some raw css
to the node's style attribute. If a nil parameter is passed, this is a no-op.
mw.html:done
html:done()
Returns the parent node under which the current node was created. Like jQuery.end, this is a convenience function to allow the construction of several child nodes to be chained together into a single statement.
mw.html:allDone
html:allDone()
Like html:done()
, but traverses all the way to the root node of the tree and returns it.
Language library
Language codes are described at language code. Many of MediaWiki's language codes are similar to IETF language tags, but not all MediaWiki language codes are valid IETF tags or vice versa.
Functions documented as mw.language.name
are available on the global mw.language
table; functions documented as mw.language:name
and lang:name
are methods of a language object (see mw.language.new
or mw.language.getContentLanguage
).
mw.language.fetchLanguageName
mw.language.fetchLanguageName( code, inLanguage )
The full name of the language for the given language code: native name (language autonym) by default, name translated in target language if a value is given for inLanguage.
mw.language.fetchLanguageNames
mw.language.fetchLanguageNames()
mw.language.fetchLanguageNames( inLanguage )
mw.language.fetchLanguageNames( inLanguage, include )
Fetch the list of languages known to MediaWiki, returning a table mapping language code to language name.
By default the name returned is the language autonym; passing a language code for inLanguage returns all names in that language.
By default, only language names known to MediaWiki are returned; passing 'all'
for include will return all available languages (from Extension:CLDR ), while passing 'mwfile'
will include only languages having customized messages included with MediaWiki core or enabled extensions. To explicitly select the default, 'mw'
may be passed.
mw.language.getContentLanguage
mw.language.getContentLanguage()
mw.getContentLanguage()
Returns a new language object for the wiki's default content language.
mw.language.getFallbacksFor
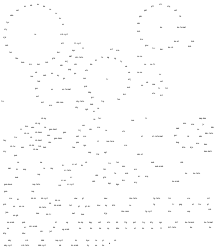
mw.language.getFallbacksFor( code )
Returns a list of MediaWiki's fallback language codes for the specified code.
mw.language.isKnownLanguageTag
mw.language.isKnownLanguageTag( code )
Returns true if a language code is known to MediaWiki.
A language code is "known" if it is a "valid built-in code" (i.e. it returns true for mw.language.isValidBuiltInCode
) and returns a non-empty string for mw.language.fetchLanguageName
.
mw.language.isSupportedLanguage
mw.language.isSupportedLanguage( code )
Checks whether any localisation is available for that language code in MediaWiki.
A language code is "supported" if it is a "valid" code (returns true for mw.language.isValidCode
), contains no uppercase letters, and has a message file in the currently-running version of MediaWiki.
It is possible for a language code to be "supported" but not "known" (i.e. returning true for mw.language.isKnownLanguageTag
). Also note that certain codes are "supported" despite mw.language.isValidBuiltInCode
returning false.
mw.language.isValidBuiltInCode
mw.language.isValidBuiltInCode( code )
Returns true if a language code is of a valid form for the purposes of internal customisation of MediaWiki.
The code may not actually correspond to any known language.
A language code is a "valid built-in code" if it is a "valid" code (i.e. it returns true for mw.language.isValidCode
); consists of only ASCII letters, numbers, and hyphens; and is at least two characters long.
Note that some codes are "supported" (i.e. returning true from mw.language.isSupportedLanguage
) even though this function returns false.
mw.language.isValidCode
mw.language.isValidCode( code )
Returns true if a language code string is of a valid form, whether or not it exists. This includes codes which are used solely for customisation via the MediaWiki namespace.
The code may not actually correspond to any known language.
A language code is valid if it does not contain certain unsafe characters (colons, single- or double-quotes, slashs, backslashs, angle brackets, ampersands, or ASCII NULs) and is otherwise allowed in a page title.
mw.language.new
mw.language.new( code )
mw.getLanguage( code )
Creates a new language object. Language objects do not have any publicly accessible properties, but they do have several methods, which are documented below.
There is a limit on the number of distinct language codes that may be used on a page. Exceeding this limit will result in errors.
mw.language:getCode
lang:getCode()
Returns the language code for this language object.
mw.language:toBcp47Code
lang:toBcp47Code()
Returns the standard BCP-47 language code for this language object. This is the code string which is appropriate to use in HTML, for example as the value of a lang
attribute.
mw.language:getFallbackLanguages
lang:getFallbackLanguages()
Returns a list of MediaWiki's fallback language codes for this language object. Equivalent to mw.language.getFallbacksFor( lang:getCode() )
.
mw.language:isRTL
lang:isRTL()
Returns true if the language is written right-to-left, false if it is written left-to-right.
mw.language:lc
lang:lc( s )
Converts the string to lowercase, honoring any special rules for the given language.
When the Ustring library is loaded, the mw.ustring.lower() function is implemented as a call to mw.language.getContentLanguage():lc( s )
.
mw.language:lcfirst
lang:lcfirst( s )
Converts the first character of the string to lowercase, as with lang:lc()
.
mw.language:uc
lang:uc( s )
Converts the string to uppercase, honoring any special rules for the given language.
When the Ustring library is loaded, the mw.ustring.upper()
function is implemented as a call to mw.language.getContentLanguage():uc( s )
.
mw.language:ucfirst
lang:ucfirst( s )
Converts the first character of the string to uppercase, as with lang:uc().
mw.language:caseFold
lang:caseFold( s )
Converts the string to a representation appropriate for case-insensitive comparison. Note that the result may not make any sense when displayed.
mw.language:formatNum
lang:formatNum( n )
lang:formatNum( n, options )
Formats a number with grouping and decimal separators appropriate for the given language. Given 123456.78, this may produce "123,456.78", "123.456,78", or even something like "ÙĄÙąÙŁÙŹÙ€Ù„ÙŠÙ«Ù§Ùš" depending on the language and wiki configuration.
The options
is a table of options, which can be:
noCommafy
: Set true to omit grouping separators and use a dot (.
) as the decimal separator. Digit transformation may still occur, which may include transforming the decimal separator.
mw.language:formatDate
lang:formatDate( format, timestamp, local )
Formats a date according to the given format string. If timestamp
is omitted, the default is the current time. The value for local
must be a boolean or nil; if true, the time is formatted in the wiki's local time rather than in UTC.
The format string and supported values for timestamp
are identical to those for the #time parser function from Extension:ParserFunctions .
Note however that backslashes may need to be doubled in a Lua string literal, since Lua also uses backslash as an escape character while wikitext does not:
-- This string literal contains a newline, not the two characters "\n", so it is not equivalent to {{#time:\n}}.
lang:formatDate( '\n' )
-- This is equivalent to {{#time:\n}}, not {{#time:\\n}}.
lang:formatDate( '\\n' )
-- This is equivalent to {{#time:\\n}}, not {{#time:\\\\n}}.
lang:formatDate( '\\\\n' )
mw.language:formatDuration
lang:formatDuration( seconds )
lang:formatDuration( seconds, chosenIntervals )
Breaks a duration in seconds into more human-readable units, e.g. 12345 to 3 hours, 25 minutes and 45 seconds, returning the result as a string.
chosenIntervals
, if given, is a table with values naming the interval units to use in the response. These include 'millennia
', 'centuries
', 'decades
', 'years
', 'weeks
', 'days
', 'hours
', 'minutes
', and 'seconds
'.
mw.language:parseFormattedNumber
lang:parseFormattedNumber( s )
This takes a number as formatted by lang:formatNum() and returns the actual number. In other words, this is basically a language-aware version of tonumber()
.
mw.language:convertPlural
lang:convertPlural( n, ... )
lang:convertPlural( n, forms )
lang:plural( n, ... )
lang:plural( n, forms )
This chooses the appropriate grammatical form from forms
(which must be a sequence table) or ...
based on the number n
. For example, in English you might use n .. ' ' .. lang:plural( n, 'sock', 'socks' )
or n .. ' ' .. lang:plural( n, { 'sock', 'socks' } )
to generate grammatically-correct text whether there is only 1 sock or 200 socks.
The necessary values for the sequence are language-dependent, see localization of magic words and translatewiki's FAQ on PLURAL for some details.
mw.language:convertGrammar
lang:convertGrammar( word, case )
lang:grammar( case, word )
- Note the different parameter order between the two aliases.
convertGrammar
matches the order of the method of the same name on MediaWiki's Language object, whilegrammar
matches the order of the parser function of the same name, documented at Help:Magic words#Localisation.
This chooses the appropriate inflected form of word
for the given inflection code case
.
The possible values for word
and case
are language-dependent, see Special:MyLanguage/Help:Magic words#Localisation and translatewiki:Grammar for some details.
mw.language:gender
lang:gender( what, masculine, feminine, neutral )
lang:gender( what, { masculine, feminine, neutral } )
Chooses the string corresponding to the gender of what
, which may be "male", "female", or a registered user name.
mw.language:getArrow
lang:getArrow( direction )
Returns a Unicode arrow character corresponding to direction
:
- forwards: Either "â" or "â" depending on the directionality of the language.
- backwards: Either "â" or "â" depending on the directionality of the language.
- left: "â"
- right: "â"
- up: "â"
- down: "â"
mw.language:getDir
lang:getDir()
Returns "ltr" or "rtl", depending on the directionality of the language.
mw.language:getDirMark
lang:getDirMark( opposite )
Returns a string containing either U+200E (the left-to-right mark) or U+200F (the right-to-left mark), depending on the directionality of the language and whether opposite
is a true or false value.
mw.language:getDirMarkEntity
lang:getDirMarkEntity( opposite )
Returns "‎" or "‏", depending on the directionality of the language and whether opposite
is a true or false value.
mw.language:getDurationIntervals
lang:getDurationIntervals( seconds )
lang:getDurationIntervals( seconds, chosenIntervals )
Breaks a duration in seconds into more human-readable units, e.g. 12345 to 3 hours, 25 minutes and 45 seconds, returning the result as a table mapping unit names to numbers.
chosenIntervals
, if given, is a table with values naming the interval units to use in the response. These include 'millennia
', 'centuries
', 'decades
', 'years
', 'weeks
', 'days
', 'hours
', 'minutes
', and 'seconds
'.
Those unit keywords are also the keys used in the response table. Only units with a non-zero value are set in the response, unless the response would be empty in which case the smallest unit is returned with a value of 0.
Message library
This library is an interface to the localisation messages and the MediaWiki: namespace.
Functions documented as mw.message.name
are available on the global mw.message
table; functions documented as mw.message:name
and msg:name
are methods of a message object (see mw.message.new
).
mw.message.new
mw.message.new( key, ... )
Creates a new message object for the given message key
.
The remaining parameters are passed to the new object's params()
method.
The message object has no properties, but has several methods documented below.
mw.message.newFallbackSequence
mw.message.newFallbackSequence( ... )
Creates a new message object for the given messages (the first one that exists will be used).
The message object has no properties, but has several methods documented below.
mw.message.newRawMessage
mw.message.newRawMessage( msg, ... )
Creates a new message object, using the given text directly rather than looking up an internationalized message. The remaining parameters are passed to the new object's params()
method.
The message object has no properties, but has several methods documented below.
mw.message.rawParam
mw.message.rawParam( value )
Wraps the value so that it will not be parsed as wikitext by msg:parse()
.
mw.message.numParam
mw.message.numParam( value )
Wraps the value so that it will automatically be formatted as by lang:formatNum()
. Note this does not depend on the Language library actually being available.
mw.message.getDefaultLanguage
mw.message.getDefaultLanguage()
Returns a Language object for the default language.
mw.message:params
msg:params( ... )
msg:params( params )
Add parameters to the message, which may be passed as individual arguments or as a sequence table. Parameters must be numbers, strings, or the special values returned by mw.message.numParam() or mw.message.rawParam(). If a sequence table is used, parameters must be directly present in the table; references using the __index metamethod will not work.
Returns the msg
object, to allow for call chaining.
mw.message:rawParams
msg:rawParams( ... )
msg:rawParams( params )
Like :params(), but has the effect of passing all the parameters through mw.message.rawParam() first.
Returns the msg
object, to allow for call chaining.
mw.message:numParams
msg:numParams( ... )
msg:numParams( params )
Like :params(), but has the effect of passing all the parameters through mw.message.numParam() first.
Returns the msg
object, to allow for call chaining.
mw.message:inLanguage
msg:inLanguage( lang )
Specifies the language to use when processing the message. lang
may be a string or a table with a getCode()
method (i.e. a Language object).
The default language is the one returned by mw.message.getDefaultLanguage()
.
Returns the msg
object, to allow for call chaining.
mw.message:useDatabase
msg:useDatabase( bool )
Specifies whether to look up messages in the MediaWiki: namespace (i.e. look in the database), or just use the default messages distributed with MediaWiki.
The default is true.
Returns the msg
object, to allow for call chaining.
mw.message:plain
msg:plain()
Substitutes the parameters and returns the message wikitext as-is. Template calls and parser functions are intact.
mw.message:exists
msg:exists()
Returns a boolean indicating whether the message key exists.
mw.message:isBlank
msg:isBlank()
Returns a boolean indicating whether the message key has content. Returns true if the message key does not exist or the message is the empty string.
mw.message:isDisabled
msg:isDisabled()
Returns a boolean indicating whether the message key is disabled. Returns true if the message key does not exist or if the message is the empty string or the string "-".
Site library
mw.site.currentVersion
A string holding the current version of MediaWiki.
mw.site.scriptPath
The value of $wgScriptPath
.
mw.site.server
The value of $wgServer
.
mw.site.siteName
The value of $wgSitename
.
mw.site.stylePath
The value of $wgStylePath
.
mw.site.namespaces
Table holding data for all namespaces, indexed by number.
The data available is:
- id: Namespace number.
- name: Local namespace name.
- canonicalName: Canonical namespace name.
- displayName: Set on namespace 0, the name to be used for display (since the name is often the empty string).
- hasSubpages: Whether subpages are enabled for the namespace.
- hasGenderDistinction: Whether the namespace has different aliases for different genders.
- isCapitalized: Whether the first letter of pages in the namespace is capitalized.
- isContent: Whether this is a content namespace.
- isIncludable: Whether pages in the namespace can be transcluded.
- isMovable: Whether pages in the namespace can be moved.
- isSubject: Whether this is a subject namespace.
- isTalk: Whether this is a talk namespace.
- defaultContentModel: The default content model for the namespace, as a string.
- aliases: List of aliases for the namespace.
- subject: Reference to the corresponding subject namespace's data.
- talk: Reference to the corresponding talk namespace's data.
- associated: Reference to the associated namespace's data.
A metatable is also set that allows for looking up namespaces by name (localized or canonical). For example, both mw.site.namespaces[4]
and mw.site.namespaces.Project
will return information about the Project namespace.
mw.site.contentNamespaces
Table holding just the content namespaces, indexed by number. See mw.site.namespaces for details.
mw.site.subjectNamespaces
Table holding just the subject namespaces, indexed by number. See mw.site.namespaces for details.
mw.site.talkNamespaces
Table holding just the talk namespaces, indexed by number. See mw.site.namespaces for details.
mw.site.stats
Table holding site statistics. Available statistics are:
- pages: Number of pages in the wiki.
- articles: Number of articles in the wiki.
- files: Number of files in the wiki.
- edits: Number of edits in the wiki.
- users: Number of users in the wiki.
- activeUsers: Number of active users in the wiki.
- admins: Number of users in group 'sysop' in the wiki.
mw.site.stats.pagesInCategory
mw.site.stats.pagesInCategory( category, which )
- This function is expensive
Gets statistics about the category. If which
has the special value "*
", the result is a table with the following properties:
- all: Total pages, files, and subcategories.
- subcats: Number of subcategories.
- files: Number of files.
- pages: Number of pages.
If which
is one of the above keys ("all", "subcats", "files", "pages"), the result is a number with the corresponding value.
Each new category queried will increment the expensive function count.
mw.site.stats.pagesInNamespace
mw.site.stats.pagesInNamespace( ns )
Returns the number of pages in the given namespace (specify by number).
mw.site.stats.usersInGroup
mw.site.stats.usersInGroup( group )
Returns the number of users in the given group.
mw.site.interwikiMap
mw.site.interwikiMap( filter )
Returns a table holding data about available interwiki prefixes. If filter
is the string "local", then only data for local interwiki prefixes is returned. If filter
is the string "!local", then only data for non-local prefixes is returned. If no filter is specified, data for all prefixes is returned. A "local" prefix in this context is one that is for the same project. For example, on the English Wikipedia, other-language Wikipedias are considered local, while Wiktionary and such are not.
Keys in the table returned by this function are interwiki prefixes, and the values are subtables with the following properties:
- prefix - the interwiki prefix.
- url - the URL that the interwiki points to. The page name is represented by the parameter $1.
- isProtocolRelative - a boolean showing whether the URL is protocol-relative.
- isLocal - whether the URL is for a site in the current project.
- isCurrentWiki - whether the URL is for the current wiki.
- isTranscludable - whether pages using this interwiki prefix are transcludable. This requires scary transclusion, which is disabled on Wikimedia wikis.
- isExtraLanguageLink - whether the interwiki is listed in
$wgExtraInterlanguageLinkPrefixes
. - displayText - for links listed in $wgExtraInterlanguageLinkPrefixes, this is the display text shown for the interlanguage link. Nil if not specified.
- tooltip - for links listed in $wgExtraInterlanguageLinkPrefixes, this is the tooltip text shown when users hover over the interlanguage link. Nil if not specified.
Text library
The text library provides some common text processing functions missing from the String library and the Ustring library. These functions are safe for use with UTF-8 strings.
mw.text.decode
mw.text.decode( s )
mw.text.decode( s, decodeNamedEntities )
Replaces HTML entities in the string with the corresponding characters.
If boolean decodeNamedEntities
is omitted or false, the only named entities recognized are <
(<), >
(>), &
(&), "
(") and
(the non-breaking space, U+00A0).
Otherwise, the list of HTML5 named entities to recognize is loaded from PHP's get_html_translation_table
function.
Known bugs: Approximately 600 of around 2,200 named entities in the HTML5 standard do not get decoded, even when decodeNamedEntities
is used; this includes approximately 40 of around 250 entities which are also included in HTML4.
This occurs because PHP's get_html_translation_table
function returns only one mapping for each character, so for example
is not decoded since PHP returns only →
as the mapping for →
.
â
mw.text.encode
mw.text.encode( s )
mw.text.encode( s, charset )
Replaces characters in a string with HTML entities.
Five characters are replaced with the appropriate named entities: <
, >
, &
, "
and the non-breaking space (U+00A0).
All others are replaced with numeric entities.
If charset
is supplied, it should be a string as appropriate to go inside brackets in a Ustring pattern, i.e. the "set" in [set]
. The default charset contains six characters: <
, >
, &
, "
, '
and the non-breaking space (U+00A0).
mw.text.jsonDecode
mw.text.jsonDecode( s )
mw.text.jsonDecode( s, flags )
Decodes a JSON string. flags
is 0 or a combination (use +
) of the flags mw.text.JSON_PRESERVE_KEYS
and mw.text.JSON_TRY_FIXING
.
Normally JSON's zero-based arrays are renumbered to Lua one-based sequence tables; to prevent this, pass mw.text.JSON_PRESERVE_KEYS
.
To relax certain requirements in JSON, such as no terminal comma in arrays or objects, pass mw.text.JSON_TRY_FIXING
. This is not recommended.
Limitations:
- Decoded JSON arrays may not be Lua sequences if the array contains null values.
- JSON objects will drop keys having null values.
- It is not possible to directly tell whether the input was a JSON array or a JSON object with sequential integer keys.
- A JSON object having sequential integer keys beginning with 1 will decode to the same table structure as a JSON array with the same values, despite these not being at all equivalent, unless
mw.text.JSON_PRESERVE_KEYS
is used.
mw.text.jsonEncode
mw.text.jsonEncode( value )
mw.text.jsonEncode( value, flags )
Encode a JSON string. Errors are raised if the passed value cannot be encoded in JSON. flags
is 0 or a combination (use +
) of the flags mw.text.JSON_PRESERVE_KEYS
and mw.text.JSON_PRETTY
.
Normally Lua one-based sequence tables are encoded as JSON zero-based arrays; when mw.text.JSON_PRESERVE_KEYS
is set in flags
, zero-based sequence tables are encoded as JSON arrays.
Limitations:
- Empty tables are always encoded as empty arrays (
[]
), not empty objects ({}
). - Sequence tables cannot be encoded as JSON objects without adding a "dummy" element.
- To produce objects or arrays with nil values, a tricky implementation of the
__pairs
metamethod is required. - A Lua table having sequential integer keys beginning with 0 will encode as a JSON array, the same as a Lua table having integer keys beginning with 1, unless
mw.text.JSON_PRESERVE_KEYS
is used. - When both a number and the string representation of that number are used as keys in the same table, behavior is unspecified.
mw.text.killMarkers
mw.text.killMarkers( s )
Removes all MediaWiki strip markers from a string.
mw.text.listToText
mw.text.listToText( list )
mw.text.listToText( list, separator, conjunction )
Joins a list, prose-style. In other words, it's like table.concat()
but with a different separator before the final item.
The default separator is taken from MediaWiki:comma-separator in the wiki's content language, and the default conjunction is MediaWiki:and concatenated with MediaWiki:word-separator.
Examples, using the default values for the messages:
-- Returns the empty string
mw.text.listToText( {} )
-- Returns "1"
mw.text.listToText( { 1 } )
-- Returns "1 and 2"
mw.text.listToText( { 1, 2 } )
-- Returns "1, 2, 3, 4 and 5"
mw.text.listToText( { 1, 2, 3, 4, 5 } )
-- Returns "1; 2; 3; 4 or 5"
mw.text.listToText( { 1, 2, 3, 4, 5 }, '; ', ' or ' )
mw.text.nowiki
mw.text.nowiki( s )
Replaces various characters in the string with HTML entities to prevent their interpretation as wikitext. This includes:
- The following characters:
"
,&
,'
,<
,=
,>
,[
,]
,{
,|
,}
- The following characters at the start of the string or immediately after a newline:
#
,*
,:
,;
, space, tab (\t
) - Blank lines will have one of the associated newline or carriage return characters escaped
----
at the start of the string or immediately after a newline will have the first-
escaped__
will have one underscore escaped://
will have the colon escaped- A whitespace character following
ISBN
,RFC
, orPMID
will be escaped
mw.text.split
mw.text.split( s, pattern, plain )
Splits the string into substrings at boundaries matching the Ustring pattern pattern
. If plain
is specified and true, pattern
will be interpreted as a literal string rather than as a Lua pattern (just as with the parameter of the same name for mw.ustring.find()
). Returns a table containing the substrings.
For example, mw.text.split( 'a b\tc\nd', '%s' )
would return a table { 'a', 'b', 'c', 'd' }
.
If pattern
matches the empty string, s
will be split into individual characters.
mw.text.gsplit
mw.text.gsplit( s, pattern, plain )
Returns an iterator function that will iterate over the substrings that would be returned by the equivalent call to mw.text.split()
.
mw.text.tag
mw.text.tag( name, attrs, content )
mw.text.tag{ name = string, attrs = table, content = string|false }
- Note the use of named arguments.
Generates an HTML-style tag for name
.
If attrs
is given, it must be a table with string keys. String and number values are used as the value of the attribute; boolean true results in the key being output as an HTML5 valueless parameter; boolean false skips the key entirely; and anything else is an error.
If content
is not given (or is nil), only the opening tag is returned. If content
is boolean false, a self-closed tag is returned. Otherwise it must be a string or number, in which case that content is enclosed in the constructed opening and closing tag. Note the content is not automatically HTML-encoded; use mw.text.encode() if needed.
For properly returning extension tags such as <ref>
, use frame:extensionTag() instead.
mw.text.trim
mw.text.trim( s )
mw.text.trim( s, charset )
Remove whitespace or other characters from the beginning and end of a string.
If charset
is supplied, it should be a string as appropriate to go inside brackets in a Ustring pattern, i.e. the "set" in [set]
. The default charset is ASCII whitespace, %s
, which is equivalent to "\t\r\n\f\v "
.
mw.text.truncate
mw.text.truncate( text, length )
mw.text.truncate( text, length, ellipsis )
mw.text.truncate( text, length, ellipsis, adjustLength )
Truncates text
to the specified length in code points, adding ellipsis
if truncation was performed. If length is positive, the end of the string will be truncated; if negative, the beginning will be removed. If adjustLength
is given and true, the resulting string including ellipsis will not be longer than the specified length.
The default value for ellipsis
is taken from MediaWiki:ellipsis in the wiki's content language.
Examples, using the default "..." ellipsis:
-- Returns "foobarbaz"
mw.text.truncate( "foobarbaz", 9 )
-- Returns "fooba..."
mw.text.truncate( "foobarbaz", 5 )
-- Returns "...arbaz"
mw.text.truncate( "foobarbaz", -5 )
-- Returns "foo..."
mw.text.truncate( "foobarbaz", 6, nil, true )
-- Returns "foobarbaz", because that's shorter than "foobarba..."
mw.text.truncate( "foobarbaz", 8 )
mw.text.unstripNoWiki
mw.text.unstripNoWiki( s )
Replaces MediaWiki <nowiki> strip markers with the corresponding text. Other types of strip markers are not changed.
mw.text.unstrip
mw.text.unstrip( s )
Equivalent to mw.text.killMarkers( mw.text.unstripNoWiki( s ) )
.
This no longer reveals the HTML behind special page transclusion, <ref> tags, and so on as it did in earlier versions of Scribunto.
Title library
mw.title.equals
mw.title.equals( a, b )
Test for whether two titles are equal. Note that fragments are ignored in the comparison.
mw.title.compare
mw.title.compare( a, b )
Returns -1, 0, or 1 to indicate whether the title a
is less than, equal to, or greater than title b
.
This compares titles by interwiki prefix (if any) as strings, then by namespace number, then by the unprefixed title text as a string. These string comparisons use Lua's standard <
operator.
mw.title.getCurrentTitle
mw.title.getCurrentTitle()
Returns the title object for the current page.
mw.title.new
mw.title.new( text, namespace )
mw.title.new( id )
- This function is expensive when called with an ID
Creates a new title object.
If a number id
is given, an object is created for the title with that page_id. The title referenced will be counted as linked from the current page. If the page_id does not exist, returns nil. The expensive function count will be incremented if the title object created is not for a title that has already been loaded.
If a string text
is given instead, an object is created for that title (even if the page does not exist). If the text string does not specify a namespace, namespace
(which may be any key found in mw.site.namespaces
) will be used. If the text is not a valid title, nil is returned.
mw.title.makeTitle
mw.title.makeTitle( namespace, title, fragment, interwiki )
Creates a title object with title title
in namespace namespace
, optionally with the specified fragment
and interwiki
prefix. namespace
may be any key found in mw.site.namespaces
. If the resulting title is not valid, returns nil.
Note that, unlike mw.title.new()
, this method will always apply the specified namespace. For example, mw.title.makeTitle( 'Template', 'Module:Foo' )
will create an object for the page Template:Module:Foo, while mw.title.new( 'Module:Foo', 'Template' )
will create an object for the page Module:Foo.
Note also that functionality for interwiki titles is limited to interwiki
/ isExternal
/ isLocal
and URL-related methods; other methods might not behave as expected.
Title objects
A title object has a number of properties and methods. Most of the properties are read-only.
Note that fields ending with text
return titles as string values whereas the fields ending with title
return title objects.
- id: The page_id.
0
if the page does not exist. This may be expensive. - interwiki: The interwiki prefix, or the empty string if none.
- namespace: The namespace number.
- fragment: The fragment (aka section/anchor linking), or the empty string. May be assigned.
- nsText: The text of the namespace for the page.
- subjectNsText: The text of the subject namespace for the page.
- talkNsText: The text of the talk namespace for the page, or
nil
if this title cannot have a talk page. (added in MediaWiki 1.42.0-wmf.15, refs T180911) - text: The title of the page, without the namespace or interwiki prefixes.
- prefixedText: The title of the page, with the namespace and interwiki prefixes.
- fullText: The title of the page, with the namespace and interwiki prefixes and the fragment. Interwiki is not returned if equal to the current.
- rootText: If this is a subpage, the title of the root page without prefixes. Otherwise, the same as
title.text
. - baseText: If this is a subpage, the title of the page it is a subpage of without prefixes. Otherwise, the same as
title.text
. - subpageText: If this is a subpage, just the subpage name. Otherwise, the same as
title.text
. - canTalk: Whether the page for this title could have a talk page.
- exists: Whether the page exists. Alias for
file.exists
for Media-namespace titles. For File-namespace titles this checks the existence of the file description page, not the file itself. This may be expensive. - file, fileExists: See #File metadata below.
- isContentPage: Whether this title is in a content namespace.
- isExternal: Whether this title has an interwiki prefix.
- isLocal: Whether this title is in this project. For example, on the English Wikipedia, any other Wikipedia is considered "local" while Wiktionary and such are not.
- isRedirect: Whether this is the title for a page that is a redirect. This may be expensive.
- isSpecialPage: Whether this is the title for a possible special page (i.e. a page in the Special: namespace).
- isSubpage: Whether this title is a subpage of some other title.
- isTalkPage: Whether this is a title for a talk page.
- isSubpageOf( title2 ): Whether this title is a subpage of the given title.
- inNamespace( ns ): Whether this title is in the given namespace. Namespaces may be specified by anything that is a key found in
mw.site.namespaces
. - inNamespaces( ... ): Whether this title is in any of the given namespaces. Namespaces may be specified by anything that is a key found in
mw.site.namespaces
. - hasSubjectNamespace( ns ): Whether this title's subject namespace is in the given namespace. Namespaces may be specified by anything that is a key found in
mw.site.namespaces
. - contentModel: The content model for this title, as a string. This may be expensive.
- basePageTitle: The same as
mw.title.makeTitle( title.namespace, title.baseText )
. - rootPageTitle: The same as
mw.title.makeTitle( title.namespace, title.rootText )
. - talkPageTitle: The same as
mw.title.makeTitle( mw.site.namespaces[title.namespace].talk.id, title.text )
, ornil
if this title cannot have a talk page. - subjectPageTitle: The same as
mw.title.makeTitle( mw.site.namespaces[title.namespace].subject.id, title.text )
. - redirectTarget: Returns a title object of the target of the redirect page if the page is a redirect and the page exists, returns
false
otherwise. - protectionLevels: The page's protection levels. This is a table with keys corresponding to each action (e.g.,
"edit"
and"move"
). The table values are arrays, the first item of which is a string containing the protection level. If the page is unprotected, either the table values or the array items will benil
. This is expensive. - cascadingProtection: The cascading protections applicable to the page. This is a table with keys
"restrictions"
(itself a table with keys likeprotectionLevels
has) and"sources"
(an array listing titles where the protections cascade from). If no protections cascade to the page,"restrictions"
and"sources"
will be empty. This is expensive. - subPageTitle( text ): The same as
mw.title.makeTitle( title.namespace, title.text .. '/' .. text )
. - partialUrl(): Returns
title.text
encoded as it would be in a URL. - fullUrl( query, proto ): Returns the full URL (with optional query table/string) for this title. proto may be specified to control the scheme of the resulting url:
"http"
,"https"
,"relative"
(the default), or"canonical"
. - localUrl( query ): Returns the local URL (with optional query table/string) for this title.
- canonicalUrl( query ): Returns the canonical URL (with optional query table/string) for this title.
- getContent(): Returns the (unparsed) content of the page, or
nil
if there is no page. The page will be recorded as a transclusion. - pageLang: A language object for the title's page content language, which defaults to the wiki's content language. This is expensive.
Title objects may be compared using relational operators. tostring( title )
will return title.prefixedText
.
Note that accessing any expensive field on a title object records a "link" to the page (as shown on Special:WhatLinksHere, for example). Using the title object's getContent()
method or accessing the redirectTarget
field records it as a "transclusion", and accessing the title object's file
or fileExists
fields records it as a "file link".
File metadata
Title objects representing a page in the File or Media namespace will have a property called file
. This is expensive. This is a table, structured as follows:
- exists: Whether the file exists. It will be recorded as an image usage. The
fileExists
property on a Title object exists for backwards compatibility reasons and is an alias for this property. If this isfalse
, all other file properties will benil
. - width: The width of the file. If the file contains multiple pages, this is the width of the first page.
- height: The height of the file. If the file contains multiple pages, this is the height of the first page.
- pages: If the file format supports multiple pages, this is a table containing tables for each page of the file; otherwise, it is
nil
. The # operator can be used to get the number of pages in the file. Each individual page table contains a width and height property. - size: The size of the file in bytes.
- mimeType: The MIME type of the file.
- length: The length (duration) of the media file in seconds. Zero for media types which do not support length.
Expensive properties
The properties id
, isRedirect
, exists
, and contentModel
require fetching data about the title from the database. For this reason, the expensive function count is incremented the first time one of them is accessed for a page other than the current page. Subsequent accesses of any of these properties for that page will not increment the expensive function count again.
Other properties marked as expensive will always increment the expensive function count the first time they are accessed for a page other than the current page.
URI library
mw.uri.encode
mw.uri.encode( s, enctype )
Percent-encodes the string. The default type, "QUERY"
, encodes spaces using '+' for use in query strings; "PATH"
encodes spaces as %20; and "WIKI"
encodes spaces as '_'.
Note that the "WIKI" format is not entirely reversible, as both spaces and underscores are encoded as '_'.
mw.uri.decode
mw.uri.decode( s, enctype )
Percent-decodes the string. The default type, "QUERY"
, decodes '+' to space; "PATH"
does not perform any extra decoding; and "WIKI"
decodes '_' to space.
mw.uri.anchorEncode
mw.uri.anchorEncode( s )
Encodes a string for use in a MediaWiki URI fragment.
mw.uri.buildQueryString
mw.uri.buildQueryString( table )
Encodes a table as a URI query string. Keys should be strings; values may be strings or numbers, sequence tables, or boolean false.
mw.uri.parseQueryString
mw.uri.parseQueryString( s, i, j )
Decodes the query string s
to a table. Keys in the string without values will have a value of false; keys repeated multiple times will have sequence tables as values; and others will have strings as values.
The optional numerical arguments i
and j
can be used to specify a substring of s
to be parsed, rather than the entire string. i
is the position of the first character of the substring, and defaults to 1. j
is the position of the last character of the substring, and defaults to the length of the string. Both i
and j
can be negative, as in string.sub.
mw.uri.canonicalUrl
mw.uri.canonicalUrl( page, query )
Returns a URI object for the canonical URL for a page, with optional query string/table.
mw.uri.fullUrl
mw.uri.fullUrl( page, query )
Returns a URI object for the full URL for a page, with optional query string/table.
mw.uri.localUrl
mw.uri.localUrl( page, query )
Returns a URI object for the local URL for a page, with optional query string/table.
mw.uri.new
mw.uri.new( s )
Constructs a new URI object for the passed string or table. See the description of URI objects for the possible fields for the table.
mw.uri.validate
mw.uri.validate( table )
Validates the passed table (or URI object). Returns a boolean indicating whether the table was valid, and on failure a string explaining what problems were found.
URI object
The URI object has the following fields, some or all of which may be nil:
- protocol: String protocol/scheme
- user: String user
- password: String password
- host: String host name
- port: Integer port
- path: String path
- query: A table, as from mw.uri.parseQueryString
- fragment: String fragment.
The following properties are also available:
- userInfo: String user and password
- hostPort: String host and port
- authority: String user, password, host, and port
- queryString: String version of the query table
- relativePath: String path, query string, and fragment
tostring()
will give the URI string.
Methods of the URI object are:
mw.uri:parse
uri:parse( s )
Parses a string into the current URI object. Any fields specified in the string will be replaced in the current object; fields not specified will keep their old values.
mw.uri:clone
uri:clone()
Makes a copy of the URI object.
mw.uri:extend
uri:extend( parameters )
Merges the parameters table into the object's query table.
Ustring library
The ustring library is intended to be a direct reimplementation of the standard String library, except that the methods operate on characters in UTF-8 encoded strings rather than bytes.
Most functions will raise an error if the string is not valid UTF-8; exceptions are noted.
mw.ustring.maxPatternLength
The maximum allowed length of a pattern, in bytes.
mw.ustring.maxStringLength
The maximum allowed length of a string, in bytes.
mw.ustring.byte
mw.ustring.byte( s, i, j )
Returns individual bytes; identical to string.byte().
mw.ustring.byteoffset
mw.ustring.byteoffset( s, l, i )
Returns the byte offset of a character in the string. The default for both l
and i
is 1. i
may be negative, in which case it counts from the end of the string.
The character at l
== 1 is the first character starting at or after byte i
; the character at l
== 0 is the first character starting at or before byte i
. Note this may be the same character. Greater or lesser values of l
are calculated relative to these.
mw.ustring.char
mw.ustring.char( ... )
Much like string.char(), except that the integers are Unicode codepoints rather than byte values.
local value = mw.ustring.char( 0x41f, 0x440, 0x438, 0x432, 0x435, 0x442, 0x21 ) -- value is now 'ĐŃĐžĐČĐ”Ń!'
mw.ustring.codepoint
mw.ustring.codepoint( s, i, j )
Much like string.byte(), except that the return values are codepoints and the offsets are characters rather than bytes.
mw.ustring.find
mw.ustring.find( s, pattern, init, plain )
Much like string.find(), except that the pattern is extended as described in Ustring patterns and the init
offset is in characters rather than bytes.
mw.ustring.format
mw.ustring.format( format, ... )
Identical to string.format(). Widths and precisions for strings are expressed in bytes, not codepoints.
mw.ustring.gcodepoint
mw.ustring.gcodepoint( s, i, j )
Returns three values for iterating over the codepoints in the string. i
defaults to 1, and j
to -1. This is intended for use in the iterator form of for
:
for codepoint in mw.ustring.gcodepoint( s ) do
-- block
end
mw.ustring.gmatch
mw.ustring.gmatch( s, pattern )
Much like string.gmatch(), except that the pattern is extended as described in Ustring patterns.
Known bugs: When used with a lone frontier pattern (%f[set]
), the function will get stuck in an infinite loop. For example, the following loop never terminates:
for capture in mw.ustring.gmatch( "foo bar", "%f[%w]" ) do
-- block
end
mw.ustring.gsub
mw.ustring.gsub( s, pattern, repl, n )
Much like string.gsub(), except that the pattern is extended as described in Ustring patterns.
Known bugs: When repl
is a table, it is possible to use numbers as keys instead of strings (e.g. to replace instances of
in a string, the value at key "5"
or [5]
would be used); as such, the output is not predictable if they have different (non-nil) values.
This is not an issue for string.gsub(), which ignores any numbers as keys.
["5"]
mw.ustring.isutf8
mw.ustring.isutf8( s )
Returns true if the string is valid UTF-8, false if not.
mw.ustring.len
mw.ustring.len( s )
Returns the length of the string in codepoints, or nil if the string is not valid UTF-8.
See string.len() for a similar function that uses byte length rather than codepoints.
mw.ustring.lower
mw.ustring.lower( s )
Much like string.lower(), except that all characters with lowercase to uppercase definitions in Unicode are converted.
If the Language library is also loaded, this will instead call lc() on the default language object.
mw.ustring.match
mw.ustring.match( s, pattern, init )
Much like string.match(), except that the pattern is extended as described in Ustring patterns and the init
offset is in characters rather than bytes.
mw.ustring.rep
mw.ustring.rep( s, n )
Identical to string.rep().
mw.ustring.sub
mw.ustring.sub( s, i, j )
Much like string.sub(), except that the offsets are characters rather than bytes.
mw.ustring.toNFC
mw.ustring.toNFC( s )
Converts the string to Normalization Form C (also known as Normalization Form Canonical Composition). Returns nil if the string is not valid UTF-8.
mw.ustring.toNFD
mw.ustring.toNFD( s )
Converts the string to Normalization Form D (also known as Normalization Form Canonical Decomposition). Returns nil if the string is not valid UTF-8.
mw.ustring.toNFKC
mw.ustring.toNFKC( s )
Converts the string to Normalization Form KC (also known as Normalization Form Compatibility Composition). Returns nil if the string is not valid UTF-8.
mw.ustring.toNFKD
mw.ustring.toNFKD( s )
Converts the string to Normalization Form KD (also known as Normalization Form Compatibility Decomposition). Returns nil if the string is not valid UTF-8.
mw.ustring.upper
mw.ustring.upper( s )
Much like string.upper(), except that all characters with uppercase to lowercase definitions in Unicode are converted.
If the Language library is also loaded, this will instead call uc() on the default language object.
Ustring patterns
Patterns in the ustring functions use the same syntax as the String library patterns. The major difference is that the character classes are redefined in terms of Unicode character properties:
%a
: represents all characters with General Category "Letter".%c
: represents all characters with General Category "Control".%d
: represents all characters with General Category "Number, decimal digit".%l
: represents all characters with General Category "Lowercase Letter".%p
: represents all characters with General Category "Punctuation".%s
: represents all characters with General Category "Separator", plus tab, linefeed, carriage return, vertical tab, and form feed.%u
: represents all characters with General Category "Uppercase Letter".%w
: represents all characters with General Category "Letter" or "Decimal Number".%x
: adds fullwidth character versions of the hex digits.
Like in String library patterns, %A
, %C
, %D
, %L
, %P
, %S
, %U
, %W
and %X
here represent the complementary set ("all characters without given General Category").
In all cases, characters are interpreted as Unicode characters instead of bytes, so ranges such as [ïŒ-ïŒ]
, patterns such as %b«»
, and quantifiers applied to multibyte characters will work correctly. Empty captures will capture the position in code points rather than bytes.
Known limitations: Unlike String library patterns, Ustring library patterns have a maximum length of 10,000 bytes. If the pattern exceeds this length, then the Ustring function will throw an error. Because the String library has its own maximum of 32 captures (unlike the Ustring library), it is therefore impossible to use a pattern which exceeds both limits, as it will be incompatible with both libraries.
Note: 9 ASCII characters, $
, +
, <
, =
, >
, ^
, `
, |
, ~
, can be matched by %p
in the string library but not in the ustring library, as Unicode classifies them as Symbols rather than Punctuation.
Loadable libraries
These libraries are not included by default, but if needed may be loaded using require()
.
bit32
This emulation of the Lua 5.2 bit32
library may be loaded using:
bit32 = require( 'bit32' )
The bit32 library provides bitwise operations on unsigned 32-bit integers. Input numbers are truncated to integers (in an unspecified manner) and reduced modulo 232 so the value is in the range 0 to 232â1; return values are also in this range.
When bits are numbered (as in bit32.extract()), 0 is the least-significant bit (the one with value 20) and 31 is the most-significant (the one with value 231).
bit32.band
bit32.band( ... )
Returns the bitwise AND of its arguments: the result has a bit set only if that bit is set in all of the arguments.
If given zero arguments, the result has all bits set.
bit32.bnot
bit32.bnot( x )
Returns the bitwise complement of x
.
bit32.bor
bit32.bor( ... )
Returns the bitwise OR of its arguments: the result has a bit set if that bit is set in any of the arguments.
If given zero arguments, the result has all bits clear.
bit32.btest
bit32.btest( ... )
Equivalent to bit32.band( ... ) ~= 0
bit32.bxor
bit32.bxor( ... )
Returns the bitwise XOR of its arguments: the result has a bit set if that bit is set in an odd number of the arguments.
If given zero arguments, the result has all bits clear.
bit32.extract
bit32.extract( n, field, width )
Extracts width
bits from n
, starting with bit field
. Accessing bits outside of the range 0 to 31 is an error.
If not specified, the default for width
is 1.
bit32.replace
bit32.replace( n, v, field, width )
Replaces width
bits in n
, starting with bit field
, with the low width
bits from v
. Accessing bits outside of the range 0 to 31 is an error.
If not specified, the default for width
is 1.
bit32.lshift
bit32.lshift( n, disp )
Returns the number n
shifted disp
bits to the left. This is a logical shift: inserted bits are 0. This is generally equivalent to multiplying by 2disp
.
Note that a displacement over 31 will result in 0.
bit32.rshift
bit32.rshift( n, disp )
Returns the number n
shifted disp
bits to the right. This is a logical shift: inserted bits are 0. This is generally equivalent to dividing by 2disp
.
Note that a displacement over 31 will result in 0.
bit32.arshift
bit32.arshift( n, disp )
Returns the number n
shifted disp
bits to the right. This is an arithmetic shift: if disp
is positive, the inserted bits will be the same as bit 31 in the original number.
Note that a displacement over 31 will result in 0 or 4294967295.
bit32.lrotate
bit32.lrotate( n, disp )
Returns the number n
rotated disp
bits to the left.
Note that rotations are equivalent modulo 32: a rotation of 32 is the same as a rotation of 0, 33 is the same as 1, and so on.
bit32.rrotate
bit32.rrotate( n, disp )
Returns the number n
rotated disp
bits to the right.
Note that rotations are equivalent modulo 32: a rotation of 32 is the same as a rotation of 0, 33 is the same as 1, and so on.
libraryUtil
This library contains methods useful when implementing Scribunto libraries. It may be loaded using:
libraryUtil = require( 'libraryUtil' )
libraryUtil.checkType
libraryUtil.checkType( name, argIdx, arg, expectType, nilOk )
Raises an error if type( arg )
does not match expectType
. In addition, no error will be raised if arg
is nil and nilOk
is true.
name
is the name of the calling function, and argIdx
is the position of the argument in the argument list. These are used in formatting the error message.
libraryUtil.checkTypeMulti
libraryUtil.checkTypeMulti( name, argIdx, arg, expectTypes )
Raises an error if type( arg )
does not match any of the strings in the array expectTypes
.
This is for arguments that have more than one valid type.
libraryUtil.checkTypeForIndex
libraryUtil.checkTypeForIndex( index, value, expectType )
Raises an error if type( value )
does not match expectType
.
This is intended for use in implementing a __newindex
metamethod.
libraryUtil.checkTypeForNamedArg
libraryUtil.checkTypeForNamedArg( name, argName, arg, expectType, nilOk )
Raises an error if type( arg )
does not match expectType
. In addition, no error will be raised if arg
is nil and nilOk
is true.
This is intended to be used as an equivalent to libraryUtil.checkType()
in methods called using Lua's "named argument" syntax, func{ name = value }
.
libraryUtil.makeCheckSelfFunction
libraryUtil.makeCheckSelfFunction( libraryName, varName, selfObj, selfObjDesc )
This is intended for use in implementing "methods" on object tables that are intended to be called with the obj:method()
syntax. It returns a function that should be called at the top of these methods with the self
argument and the method name, which will raise an error if that self
object is not selfObj
.
This function will generally be used in a library's constructor function, something like this:
function myLibrary.new()
local obj = {}
local checkSelf = libraryUtil.makeCheckSelfFunction( 'myLibrary', 'obj', obj, 'myLibrary object' )
function obj:method()
checkSelf( self, 'method' )
end
function obj:method2()
checkSelf( self, 'method2' )
end
return obj
end
luabit
The luabit library modules "bit" and "hex" may be loaded using:
bit = require( 'luabit.bit' )
hex = require( 'luabit.hex' )
Note that the bit32 library contains the same operations as "luabit.bit", and the operations in "luabit.hex" may be performed using string.format()
and tonumber()
.
The luabit module "noki" is not available, as it is entirely useless in Scribunto. The luabit module "utf8" is also not available, as it was considered redundant to the Ustring library.
strict
The strict library is not a normal library; it causes an error to be raised whenever a new variable is used that is not explicitly scoped as a local variable (e.g., global variable assignment references). This functionality is typically enabled by loading at the top of a module using:
require( 'strict' )
On many Wikimedia wikis this was formerly implemented in Module:No globals
, which was replaced via phab:T209310. It is in part derived from strict.lua.
ustring
The pure-Lua backend to the Ustring library may be loaded using:
ustring = require( 'ustring' )
In all cases the Ustring library (mw.ustring
) should be used instead, as that replaces many of the slower and more memory-intensive operations with callbacks into PHP code.
Extension libraries
Some MediaWiki extensions provide additional Scribunto libraries. These are also located in the table mw
, usually in the table mw.ext
, however, they are only present when certain extensions are installed (in addition to the Scribunto extension itself).
Such extensions use Scribunto provided hooks:
Writing Scribunto libraries provides information on how such libraries can be developed to provide Lua interfaces for MediaWiki extensions.
mw.wikibase
Wikibase Client provides access to localizable structured data, most notably Wikidata. See docs_topics_lua.html and Extension:Wikibase Client/Lua .
mw.wikibase.lexeme
WikibaseLexeme provides access to Wikibase Lexeme entities. This is supported by Wikidata:Lexicographical data. See md_docs_2topics_2lua.html and Extension:WikibaseLexeme/Lua .
mw.wikibase.mediainfo
WikibaseMediaInfo provides access to Wikibase MediaInfo entities. See WikibaseMediaInfo/Lua . This is supported by Structured Data on Commons. See Structured data/Lua.
mw.bcmath
BCmath provides arbitrary-precision arithmetic to Lua modules. See BCmath documentation via "LDoc" link at BCmath 変Usage.
mw.smw
Semantic Scribunto provides native Scribunto support for the Semantic MediaWiki extension.
mw.ext.data
JsonConfig provides access to localizable tabular and map data. See JsonConfig/Tabular . Tabular Data and GeoJSON Map Data is supported in the "Data:" namespace at Commons.
mw.ext.data.get( pagename )
mw.ext.cargo
Cargo provides a means to query its data store from Lua. See Extension:Cargo/Other features#Lua support .
mw.ext.cattools
CategoryToolbox provides a means to check from Lua if a certain page belongs to a category. Is is experimental and not enabled on public WikiMedia wikis.
mw.ext.FlaggedRevs
FlaggedRevs provides a means to access the stability settings of a page from Lua.
mw.ext.TitleBlacklist
TitleBlacklist provides a means to test and obtain information about blacklisted page naming entries from Lua.
mw.ext.ParserFunctions
ParserFunctions provides a means from Lua to evaluate expressions in the same way as its PHP-based parser function #expr
.
mw.ext.proofreadPage
Proofread Page provides access to Index and Page namespaces. See Extension:Proofread Page/Lua reference . This is supported by Wikisource:ProofreadPage. See Help:Extension:ProofreadPage .
mw.ext.articlePlaceholder
ArticlePlaceholder provides a means to override default Wikibase renderings from Lua. See Extension:ArticlePlaceholder/Module:AboutTopic .
mw.ext.externalData
ExternalData provides a means to get structured data from Internet from Lua. See Extension:External Data/Lua .
mw.ext.UnlinkedWikibase
See UnlinkedWikibase
mw.ext.UnlinkedWikibase.getEntity( id )
mw.ext.UnlinkedWikibase.query( sparql )
mw.ext.seo
WikiSEO provides a means to set SEO Data for the current page. See Extension:WikiSEO#Usage in lua modules.
mw.slots
WSSlots provides a number of Lua functions for working with MCR slots:
mw.slots.slotContent(slotName, pageName)
mw.slots.slotTemplates(slotName, pageName)
(deprecated)mw.slots.slotContentModel(slotName, pageName)
mw.slots.slotData(slotName, pageName)
Differences from standard Lua
Changed functions
The following functions have been modified:
- setfenv()
- getfenv()
- May not be available, depending on the configuration. If available, attempts to access parent environments will fail.
- getmetatable()
- Works on tables only to prevent unauthorized access to parent environments.
- tostring()
- Pointer addresses of tables and functions are not provided. This is to make memory corruption vulnerabilities more difficult to exploit.
- pairs()
- ipairs()
- Support for the __pairs and __ipairs metamethods (added in Lua 5.2) has been added.
- pcall()
- xpcall()
- Certain internal errors cannot be intercepted.
- require()
- Can fetch certain built-in modules distributed with Scribunto, as well as modules present in the Module namespace of the wiki. To fetch wiki modules, use the full page name including the namespace. Cannot otherwise access the local filesystem.
Removed functions and packages
The following packages are mostly removed. Only those functions listed are available:
- package.*
- Filesystem and C library access has been removed. Available functions and tables are:
- package.loaded
- package.preload
- package.loaders
- Loaders which access the local filesystem or load C libraries are not present. A loader for Module-namespace pages is added.
- package.seeall()
- os.*
- There are some insecure functions in here, such as os.execute(), which can't be allowed. Available functions are:
- debug.*
- Most of the functions are insecure. Available functions are:
The following functions and packages are not available:
- collectgarbage()
- module()
- coroutine.*
- No application is known for us, so it has not been reviewed for security.
- dofile()
- loadfile()
- io.*, file.*
- Allows local filesystem access, which is insecure.
- load()
- loadstring()
- These were omitted to allow for static analysis of the Lua source code. Also, allowing these would allow Lua code to be added directly to article and template pages, which was not desired for usability reasons.
- print()
- This was discussed on wikitech-l and it was decided that it should be omitted in favour of return values, to improve code quality. If necessary, mw.log() may be used to output information to the debug console.
- string.dump()
- May expose private data from parent environments.
Additional caveats
- Referential data structures
- Circular data structures and data structures where the same node may be reached by more than one path cannot be correctly sent to PHP. Attempting to do so will cause undefined behavior. This includes (but is not limited to) returning such data structures from the module called by
{{#invoke:}}
and passing such data structures as parameters to Scribunto library functions that are implemented as callbacks into PHP.
Such data structures may be used freely within Lua, including as the return values of modules loaded withmw.loadData()
.
Writing Scribunto libraries
This information is useful to developers writing additional Scribunto libraries, whether for inclusion in Scribunto itself or for providing an interface for their own extensions.
A Scribunto library will generally consist of five parts:
- The PHP portion of the library.
- The Lua portion of the library.
- The PHP portion of the test cases.
- The Lua portion of the test cases.
- The documentation.
Existing libraries serve as a good example.
Library
The PHP portion of the library is a class that must extend Scribunto_LuaLibraryBase
. See the documentation for that class for implementation details. In the Scribunto extension, this file should be placed in engines/LuaCommon/NameLibrary.php
, and a mapping added to Scribunto_LuaEngine::$libraryClasses
. Other extensions should use the ScribuntoExternalLibraries hook. In either case, the key should match the Lua module name ("mw.name" for libraries in Scribunto, or "mw.ext.name" for extension libraries).
The Lua portion of the library sets up the table containing the functions that can be called from Lua modules. In the Scribunto extension, the file should be placed in engines/LuaCommon/lualib/mw.name.lua
. This file should generally include boilerplate something like this:
local object = {}
local php
function object.setupInterface( options )
-- Remove setup function
object.setupInterface = nil
-- Copy the PHP callbacks to a local variable, and remove the global
php = mw_interface
mw_interface = nil
-- Do any other setup here
-- Install into the mw global
mw = mw or {}
mw.ext = mw.ext or {}
mw.ext.NAME = object
-- Indicate that we're loaded
package.loaded['mw.ext.NAME'] = object
end
return object
The module in engines/LuaCommon/lualib/libraryUtil.lua
(load this with local util = require 'libraryUtil'
) contains some functions that may be helpful.
Be sure to run the Scribunto test cases with your library loaded, even if your library doesn't itself provide any test cases. The standard test cases include tests for things like libraries adding unexpected global variables. Also, if the library is loaded with PHP, any upvalues that its Lua functions have will not be reset between #invoke's. Care must be taken to ensure that modules can't abuse this to transfer information between #invoke's.
Test cases
The Scribunto extension includes a base class for test cases, Scribunto_LuaEngineTestBase
, which will run the tests against both the LuaSandbox and LuaStandalone engines.
The library's test case should extend this class, and should not override static function suite()
.
In the Scribunto extension, the test case should be in tests/engines/LuaCommon/NameLibraryTest.php
and added to the array in ScribuntoHooks::unitTestsList()
(in common/Hooks.php
); extensions should add the test case in their own UnitTestsList
hook function, probably conditional on whether $wgAutoloadClasses['Scribunto_LuaEngineTestBase']
is set.
Most of the time, all that is needed to make the test case is this:
class ClassNameTest extends Scribunto_LuaEngineTestBase { protected static $moduleName = 'ClassNameTest'; function getTestModules() { return parent::getTestModules() + array( 'ClassNameTest' => __DIR__ . '/ClassNameTests.lua'; ); } }
This will load the file ClassNameTests.lua
as if it were the page "Module:ClassNameTests", expecting it to return an object with the following properties:
- count: Integer, number of tests
- provide( n ): Function that returns three values:
n
, the name of testn
, and a string that is the expected output for testn
. - run( n ): Function that runs test
n
and returns one string.
If getTestModules()
is declared as shown, "Module:TestFramework" is available which provides many useful helper methods. If this is used, ClassNameTests.lua
would look something like this:
local testframework = require 'Module:TestFramework' return testframework.getTestProvider( { -- Tests go here } )
Each test is itself a table, with the following properties:
- name: The name of the test.
- func: The function to execute.
- args: Optional table of arguments to pass to the function.
- expect: Results to expect.
- type: Optional "type" of the test, default is "Normal".
The type controls the format of expect
and how func
is called. Included types are:
- Normal:
expect
is a table of return values, or a string if the test should raise an error.func
is simply called. - Iterator:
expect
is a table of tables of return values.func
is called as with an iterated for loop, and each iteration's return values are accumulated. - ToString: Like "Normal", except each return value is passed through
tostring()
.
Test cases in another extension
There are (at least) two ways to run PHPUnit tests:
- Run phpunit against core, allowing the tests/phpunit/suites/ExtensionsTestSuite.php to find the extension's tests using the UnitTestsList hook. If your extension's test class names all contain a unique component (e.g. the extension's name), the
--filter
option may be used to run only your extension's tests. - Run phpunit against the extension directory, where it will pick up any file ending in "Test.php".
Either of these will work fine if Scribunto is loaded in LocalSettings.php. And it is easy for method #1 to work if Scribunto is not loaded, as the UnitTestsList hook can easily be written to avoid returning the Scribunto test when $wgAutoloadClasses[ 'Scribunto_LuaEngineTestBase' ]
is not set.
But Jenkins uses method #2. For Jenkins to properly run the tests, you will need to add Scribunto as a dependency for your extension. See Gerrit change 56570 for an example of how this is done.
If for some reason you need the tests to be able to run using method #2 without Scribunto loaded, one workaround is to add this check to the top of your unit test file:
if ( !isset( $GLOBALS['wgAutoloadClasses']['Scribunto_LuaEngineTestBase'] ) ) {
return;
}
Documentation
Modules included in Scribunto should include documentation in the Scribunto libraries section above. Extension libraries should include documentation in a subpage of their own extension page, and link to that documentation from the Extension libraries subsection above.
See also
License
This manual is derived from the Lua 5.1 reference manual, which is available under the MIT license.
Copyright © 1994â2012 Lua.org, PUC-Rio.
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
This derivative manual may also be copied under the terms of the same license.